Some more jQuery snippets to check if element is in view.
function isScrolledIntoView(elem)
{
var docViewTop = $(window).scrollTop();
var docViewBottom = docViewTop + $(window).height();
var elemTop = $(elem).offset().top;
var elemBottom = elemTop + $(elem).height();
return ((elemBottom < = docViewBottom) && (elemTop >= docViewTop));
}
isInView: function(elem)
{
var docViewTop = $(window).scrollTop(),
docViewBottom = docViewTop + $(window).height(),
elemTop = $(elem).offset().top,
elemBottom = elemTop + $(elem).height();
return ((elemBottom < = docViewBottom) && (elemTop >= docViewTop));
}
The best method I have found so far is the jQuery appear plugin. Works like a charm.
Frequently Asked Questions (FAQs) about jQuery Check Element View
How can I use jQuery to check if an element is in the viewport?
To check if an element is in the viewport using jQuery, you can use the :in-viewport
selector. This selector will return all elements that are currently visible in the viewport. Here is a simple example:if ($("element").is(":in-viewport")) {
// Element is in the viewport
} else {
// Element is not in the viewport
}
This code checks if the specified element is in the viewport and performs an action based on the result.
What is the difference between :visible
and :in-viewport
in jQuery?
The :visible
selector in jQuery selects elements that are not hidden. This means that even if an element is not currently in the viewport (i.e., it’s off-screen due to scrolling), it will still be selected by :visible
if it’s not hidden.
On the other hand, :in-viewport
selects only those elements that are currently visible in the viewport. This means that if an element is off-screen due to scrolling, it will not be selected by :in-viewport
, even if it’s not hidden.
How can I use jQuery to check if a specific part of an element is in the viewport?
To check if a specific part of an element is in the viewport, you can use the offset()
method in jQuery to get the position of the element, and then compare it with the viewport’s dimensions. Here is an example:var top_of_element = $("#element").offset().top;
var bottom_of_element = $("#element").offset().top + $("#element").outerHeight();
var top_of_screen = $(window).scrollTop();
var bottom_of_screen = $(window).scrollTop() + $(window).innerHeight();
if ((bottom_of_screen > top_of_element) && (top_of_screen < bottom_of_element)){
// The element is visible, do something
} else {
// The element is not visible, do something else
}
This code checks if the top and bottom of the element are within the viewport, and performs an action based on the result.
Can I use jQuery to check if an element is in the viewport without using any plugins?
Yes, you can use jQuery to check if an element is in the viewport without using any plugins. Here is a simple example:function isElementInViewport (el) {
var rect = el.getBoundingClientRect();
return (
rect.top >= 0 &&
rect.left >= 0 &&
rect.bottom <= (window.innerHeight || document.documentElement.clientHeight) &&
rect.right <= (window.innerWidth || document.documentElement.clientWidth)
);
}
This function uses the getBoundingClientRect()
method to get the position of the element relative to the viewport, and then checks if the element is within the viewport.
How can I use jQuery to check if an element is partially in the viewport?
To check if an element is partially in the viewport, you can use the offset()
method in jQuery to get the position of the element, and then compare it with the viewport’s dimensions. Here is an example:var top_of_element = $("#element").offset().top;
var bottom_of_element = $("#element").offset().top + $("#element").outerHeight();
var top_of_screen = $(window).scrollTop();
var bottom_of_screen = $(window).scrollTop() + $(window).innerHeight();
if ((bottom_of_screen > top_of_element) && (top_of_screen < bottom_of_element)){
// The element is partially visible, do something
} else {
// The element is not visible, do something else
}
This code checks if the top or bottom of the element is within the viewport, and performs an action based on the result.
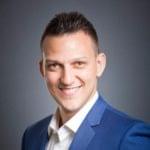
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.