OK, somehow you’ve managed to get into a situation where you need to bind to elements of the same id. Now, if you can you should add a class to each element and bind to that! Note: You should only use the live() function on elements that have been introduced dynamically within the page, otherwise use the bind() function. This little function find elements with duplicate ids.
(function(document, $){
// little debug helper script to notify us when the
// dom is updated with a contains duplicate ID'd elements
$(document).bind('DOMNodeInserted', function (event) {
var duplicateDomElements = [];
$('[id]').map(function () {
var ids = $('[id=' + this.id + ']');
if (ids.length > 1 && ids[0] == this) {
duplicateDomElements.push(this.id);
}
});
if (duplicateDomElements.length > 0) {
alert('Recent action duplicated a dom element ID with name(s) [' + duplicateDomElements + ']');
}
});
})(document, jQuery);
Note: using div#id will soemtimes produce a slower result than just #id so be careful how many preceding tags you put on your selectors. Also as a tip, if the two classes are calling the same function then you can add the selectors together like this:
$('.clickButton1, .clickButton2').bind('click', function() {
//your code
}
//instead of
$('.clickButton1').bind('click', function() {
//your code
}
$('.clickButton2').bind('click', function() {
//your code
}
Stopping Action on Duplicate Elements
A fix to stop further actions on duplicate elements is to use both prevent default and stop propagation which will stop the default action and any immediate actions for elements with the same id. Like so:e.preventDefault();
e.stopImmediatePropagation();
Further Problems: assigning classes to elements with the same id
.each is applying the class tag to the first element with that id
0 [object HTMLDivElement]
SSP0
0 [object HTMLDivElement]
SSP0
If you apply “div” to the jQuery selector it seems to work.
$('div#searchResultsContainer').each(function(index, value)
{
console.log(index);
// $(this).addClass('SSP'+index);
});
Also see: jquery binding to created elements
Frequently Asked Questions (FAQs) about jQuery Bind Elements by ID
What is the purpose of using jQuery bind elements by ID?
The primary purpose of using jQuery bind elements by ID is to attach one or more event handlers to specific elements on a webpage. This is particularly useful when you want to execute certain JavaScript functions when an event, such as a click or mouseover, occurs on the specified element. By using the ID of the element, you can precisely target the specific element you want the event handler to be attached to. This allows for greater control and specificity in your code.
How does jQuery bind differ from JavaScript bind?
While both jQuery bind and JavaScript bind serve to attach event handlers to elements, they do so in slightly different ways. JavaScript bind is a method that creates a new function, where “this” refers to a specified object. On the other hand, jQuery bind attaches an event handler directly to elements. It’s worth noting that jQuery is a library built on JavaScript, so it essentially provides a more convenient and simpler syntax for achieving the same results.
Can I bind multiple events to the same element?
Yes, you can bind multiple events to the same element using jQuery. This can be done by passing multiple event-handler pairs separated by a comma in the bind() method. This allows you to handle multiple events, such as click, mouseover, and keypress, on the same element.
How can I unbind events from an element?
You can unbind events from an element using the unbind() method in jQuery. This method removes one or all event handlers from the specified elements. You can either remove all event handlers or specify the type of event you want to unbind.
Can I use jQuery bind to attach events to elements that are dynamically added to the page?
While you can use jQuery bind to attach events to elements that are currently on the page, it does not work for elements that are added dynamically after the bind function has been called. For dynamically added elements, you should use the on() method, which attaches event handlers to current and future elements.
What is the difference between bind() and on() in jQuery?
The main difference between bind() and on() in jQuery is that bind() only attaches event handlers to elements that are currently on the page, while on() attaches event handlers to current and future elements. This makes on() more suitable for dealing with dynamically added elements.
How can I bind events to multiple elements at once?
You can bind events to multiple elements at once by using a class selector or element selector in the jQuery bind function. This will attach the specified event handler to all elements that match the selector.
Can I bind custom events using jQuery?
Yes, you can bind custom events using jQuery. This can be done by simply passing the name of your custom event as the first argument to the bind() method. You can then trigger these custom events using the trigger() method.
How can I stop event propagation using jQuery bind?
You can stop event propagation in jQuery bind by using the event.stopPropagation() method inside your event handler. This prevents the event from bubbling up the DOM tree, preventing any parent handlers from being notified of the event.
Can I pass data to the event handler using jQuery bind?
Yes, you can pass data to the event handler using jQuery bind. This can be done by passing an object as the second argument to the bind() method. The data can then be accessed inside the event handler through the event.data property.
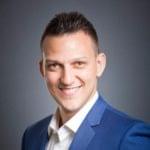
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.