If your App needs Login or Registration functionality, then using 3rd party services such as Facebook Authentication are useful tools to utilize.
This tutorial is for developers using Cordova with jQuery Mobile for UI and ngCordovaOauth to add Facebook login functionality. The app will enable users to login via Facebook and then display their basic profile information. The primary motive of this tutorial is to show how to implement Facebook login in Cordova apps and how to use ngCordovaOauth in non-Ionic or non-AngularJS UI based app.
How does OAuth Authentication Work?
The OAuth protocol is an authentication mechanism used by many web services (such as Facebook, Twitter and LinkedIn) to provide user information to third party apps.
According to the OAuth protocol, the user should be first redirected to the web service by the third party app. The user then grants permission to an app and an access token is generated that is returned to the third party app. The access token acts like a permission to access user information.
What is ngCordova and ngCordovaOauth?
ngCordova is a collection of 63+ AngularJS modules. Some are wrappers for Cordova plugins and others are wrappers for pure JavaScript libraries. ngCordovaOauth is one of the ngCordova modules.
ngCordovaOauth is a JavaScript library wrapped within an AngularJS module. The purpose of this library is to obtain an access token from a web service to use their APIs and access user information.
I will be using ngCordovaOauth because it works on all platforms and supports additional web services that can be implemented using the same methods as the Facebook login implementation.
Creating a Facebook Developer App
Every third party app that integrates Facebook login needs to create a Facebook developer app. A developer app helps Facebook track the third party app and provides additional configuration options.
To create a Facebook developer app for our Cordova app do the following:
Visit the Facebook Developer Apps Page and click Add a New App. Select Website as the platform.
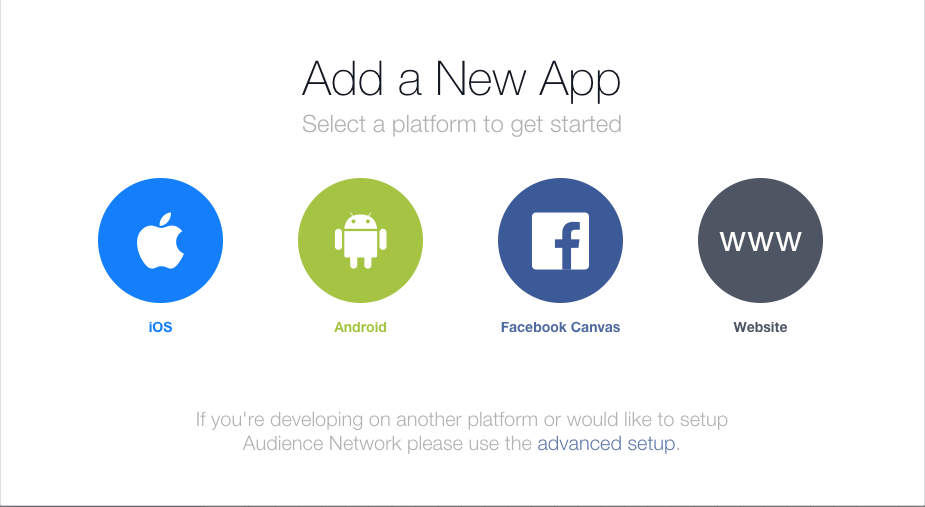
Enter an app name and click Create new Facebook App ID.
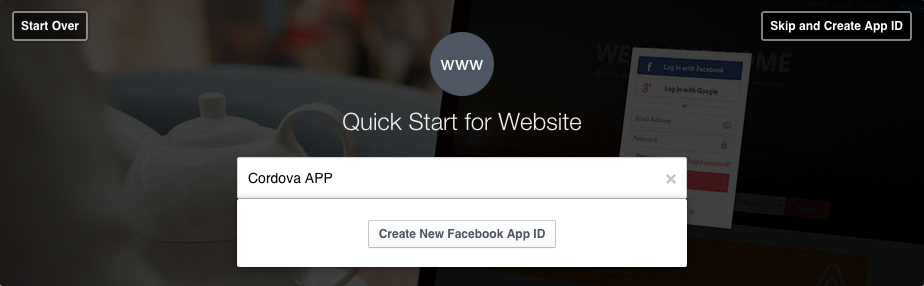
Select your app category and click Create App ID.
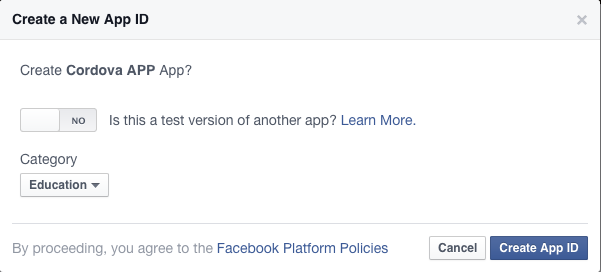
Click Skip Quick Start in the top right corner.
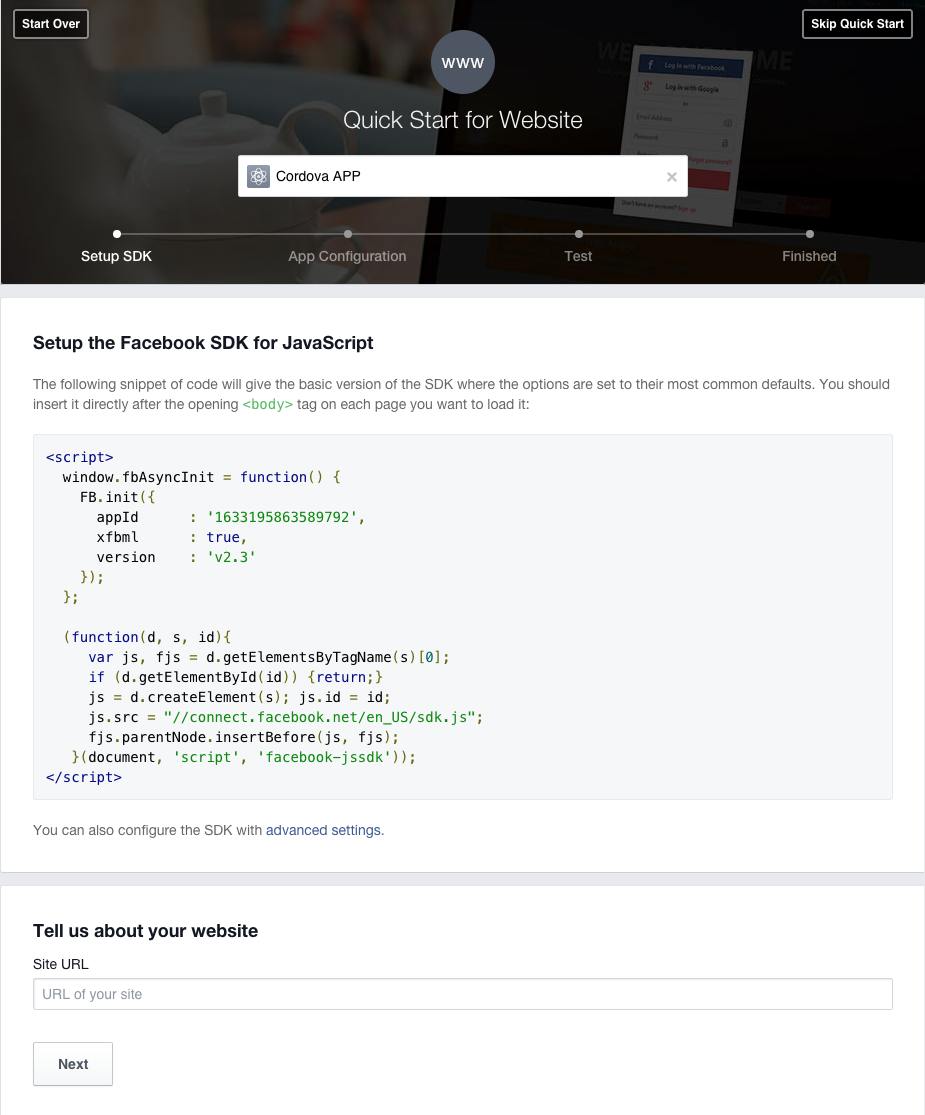
Enter the app domain and Site URL into the Basic settings tab as shown below
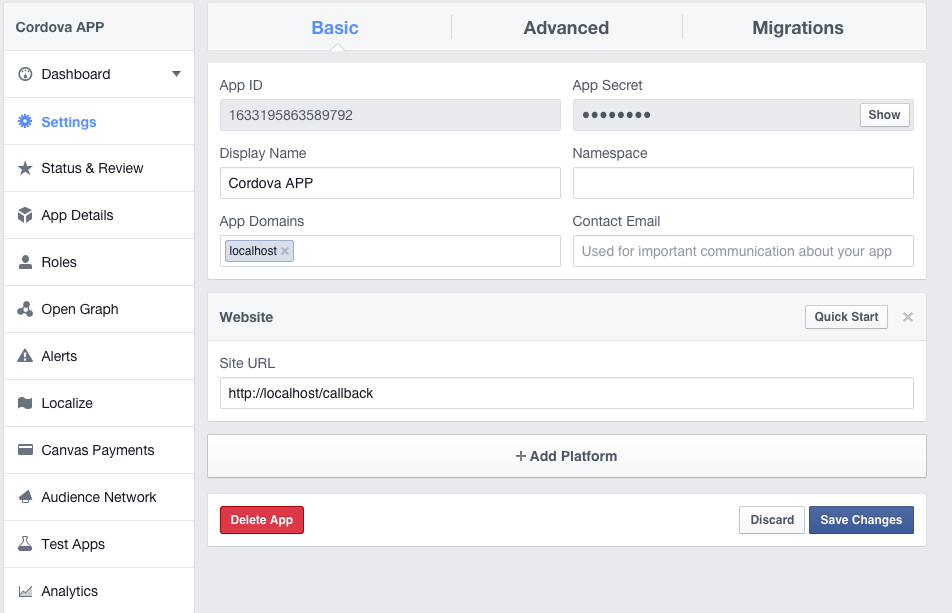
Under the Advanced tab add the redirect OAuth URL as shown below.
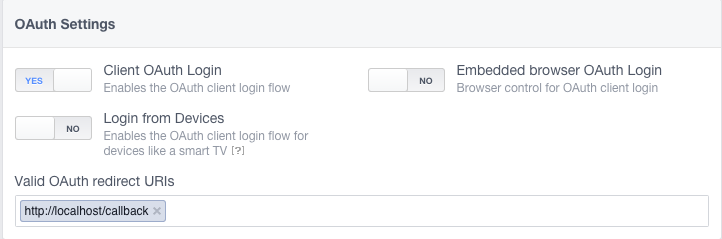
Creating a Cordova App
To create my app template I need to include jQuery mobile and ngCordovaOauth with their dependencies. I won’t cover installing and creating a Cordova Application here, if you haven’t done this before, read this getting started guide. Instructions to run and build the application are available on the same page.
Give the Application an appropriate name and add the supported platforms. I am using Cordova’s InAppBrowser plugin in this tutorial, you can find instructions here on adding plugins here.
You can find the final code of this project on gitHub.
Inside the www/index.html file, add the following JavaScript and CSS files in the head
tag. These are the jQuery Mobile and ngCordovaOauth loaded from CDNs. You should embed these files locally so that the app works without an Internet connection.
<link rel="stylesheet" href="https://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.css">
<script src="https://code.jquery.com/jquery-2.1.4.min.js"></script>
<script src="https://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.js"></script>
<script src="http://code.ionicframework.com/nightly/js/ionic.bundle.js"></script>
<script src="https://cdn.rawgit.com/driftyco/ng-cordova/master/dist/ng-cordova.min.js"></script>
<script src="https://cdn.rawgit.com/nraboy/ng-cordova-oauth/master/dist/ng-cordova-oauth.min.js"></script>
<script type="text/javascript" src="cordova.js"></script>
<script type="text/javascript" src="cordova_plugins.js"></script>
Creating the Home Screen
The home screen of our app will display a button instructing the user to login via Facebook. When the user clicks the button the user will be redirected to Facebook.
Create a AngularJS app using the ng-app
directive and a controller using the ng-controller
directive. Place this code inside the body
tag of www/index.html replacing the div
s that are currently there:
<div ng-app="facebookApp">
<div ng-controller="mainCtrl">
</div>
</div>
Now create the home screen. Place this code inside the body
tag of the www/index.html file below the code we just added:
<div data-role="page" id="home">
<div data-role="header">
<h1>Login</h1>
</div>
<div data-role="main" class="ui-content">
<p>You need to login to view your Facebook profile information</p>
<a target="_blank" href="#" onclick="login()" style="text-decoration: none"><button>Login using Facebook</button></a>
</div>
</div>
This is how the home screen should now look:
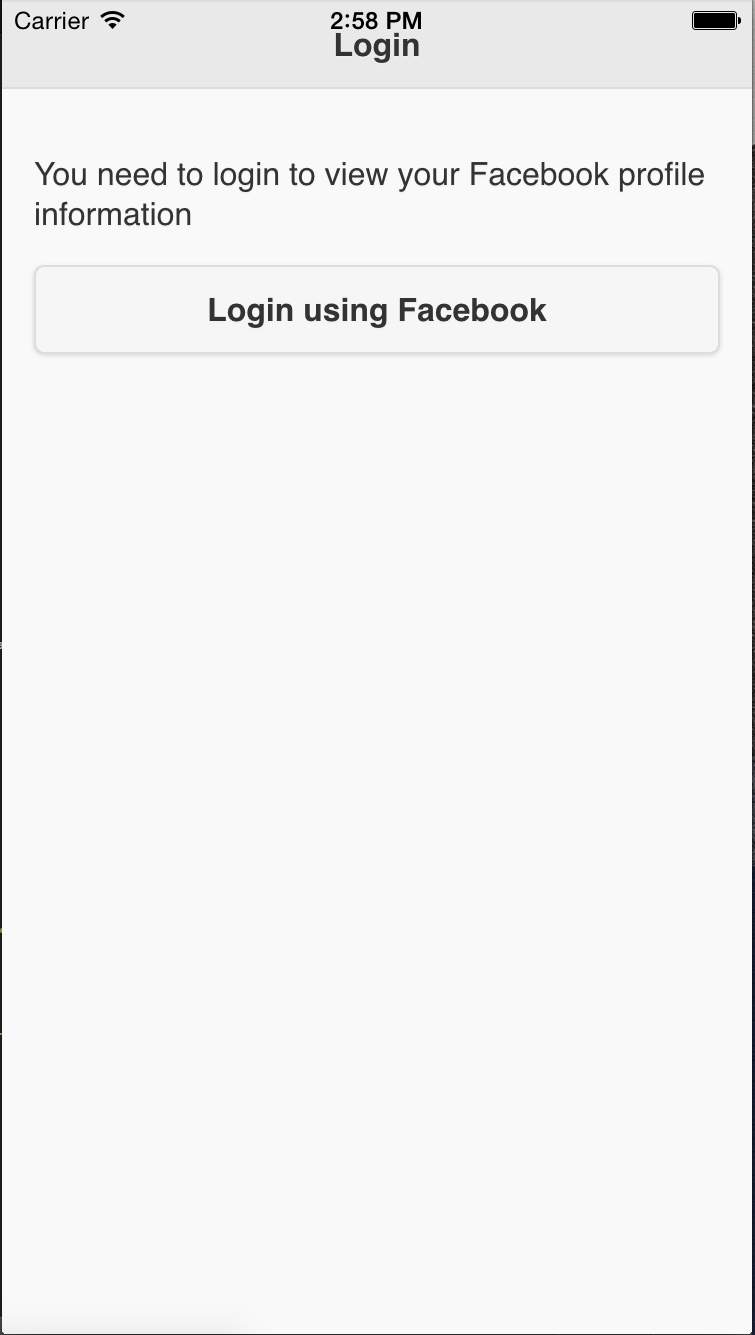
Creating the Profile Screen
Once the user gives access to the app, display the user profile information. Here is the code for the profile page, place this code below the home screen page:
<div data-role="page" id="profile">
<div data-role="header">
<a target="_blank" href="#home" class="ui-btn ui-icon-home ui-btn-icon-left">Home</a>
<h1>Profile</h1>
</div>
<div data-role="main" class="ui-content" id="listTable"></div>
</div>
Implementing Facebook Login
To initiate the AngularJS app we need to get a reference of the ngCordovaOauth
methods for the Facebook login. Place this code inside a script
tag before the closing body
tag in the www/index.html page:
angular.module("facebookApp", ["ionic", "ngCordova"]).controller("mainCtrl", ["$scope", "$cordovaOauth", "$http", function($scope, $cordovaOauth, $http) {
window.cordovaOauth = $cordovaOauth;
window.http = $http;
}]);
Here the AngularJS app is initiated and then loads the necessary modules to use ngCordovaOauth
. Then the reference to the ngCordovaOauth
and http
module is saved globally.
When the login button is clicked, the login
method is invoked and the login
method calls the facebookLogin
function. facebookLogin
is a custom function which redirects to the Facebook website. Place this code in the script
tag created previously:
function login()
{
facebookLogin(window.cordovaOauth, window.http);
}
function facebookLogin($cordovaOauth, $http)
{
$cordovaOauth.facebook("1633195863589792", ["email", "public_profile"], {redirect_uri: "http://localhost/callback"}).then(function(result){
displayData($http, result.access_token);
}, function(error){
alert("Error: " + error);
});
}
The $cordovaOauth.facebook()
method requires several parameters:
1. APP ID: The ID of the facebook developer app. This is found on the dashboard of the app.
2. Permissions: These are for accessing information about the user. A complete list of permissions can be found on the Facebook permissions page.
The $cordovaOauth.facebook()
method returns a promise object. Once the user has given access to our app, the displayData
function is called that makes a Graph API request to retrieve a range of information about the user.
Here is the displayData
function, which should be placed in the script
tag created above:
function displayData($http, access_token)
{
$http.get("https://graph.facebook.com/v2.2/me", {params: {access_token: access_token, fields: "name,gender,location,picture", format: "json" }}).then(function(result) {
var name = result.data.name;
var gender = result.data.gender;
var picture = result.data.picture;
var html = '<table id="table" data-role="table" data-mode="column" class="ui-responsive"><thead><tr><th>Field</th><th>Info</th></tr></thead><tbody>';
html = html + "<tr><td>" + "Name" + "</td><td>" + name + "</td></tr>";
html = html + "<tr><td>" + "Gender" + "</td><td>" + gender + "</td></tr>";
html = html + "<tr><td>" + "Picture" + "</td><td><img src='" + picture.data.url + "' /></td></tr>";
html = html + "</tbody></table>";
document.getElementById("listTable").innerHTML = html;
$.mobile.changePage($("#profile"), "slide", true, true);
}, function(error) {
alert("Error: " + error);
});
}
Here a Graph API request is made to retrieve the user’s name, profile picture and gender. While making Graph API requests the access token is needed. Then a responsive jQuery mobile table is created and added to the profile page content.
Here is how the profile page should now look:
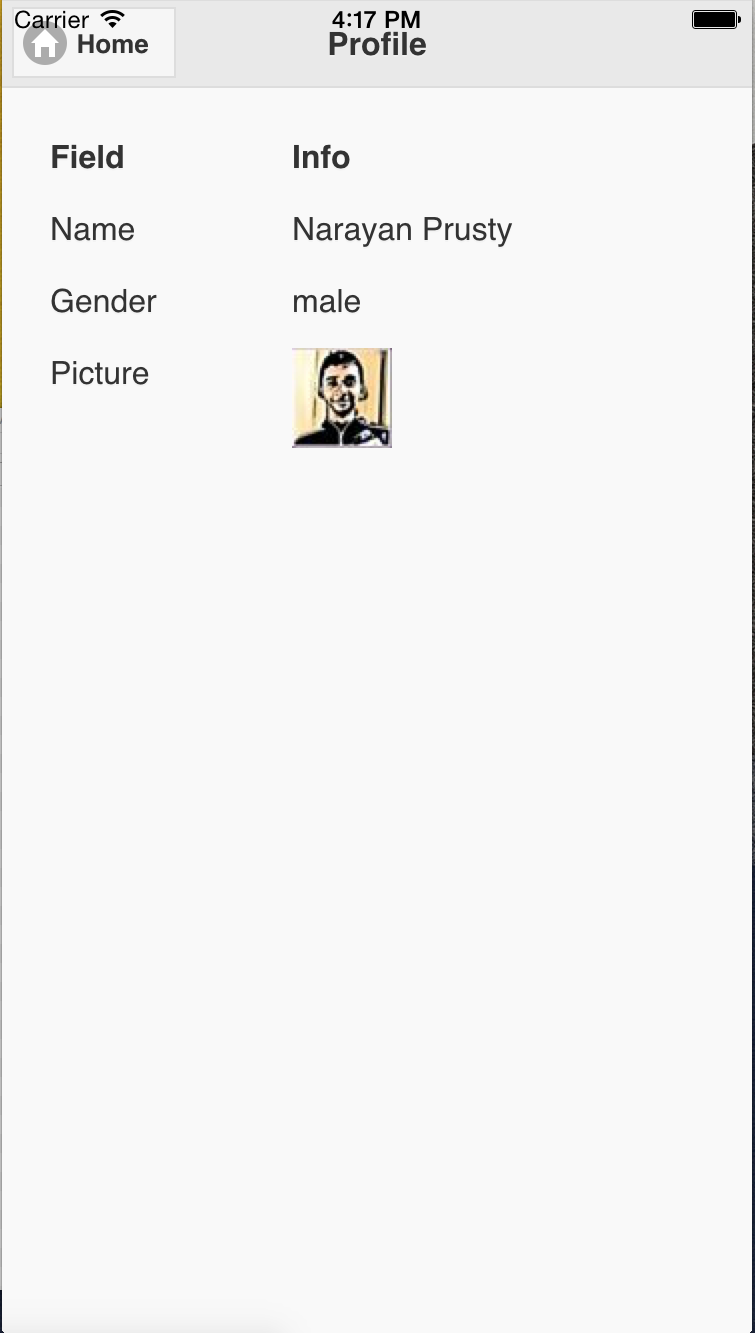
Conclusion
This app shows developers how to implement a Facebook login in Cordova apps and how to use ngCordovaOauth
in non-Ionic or non-AngularJS UI based apps. Currently the app is functional, but basic and can be deployed to multiple platforms. The next steps are displaying more information and letting users post or change their profile picture.
Let me know your experiences trying this tutorial and ideas for expanding it.
Frequently Asked Questions on Integrating Facebook Login into a Cordova-based App
How do I set up Facebook Login for my Cordova-based app?
Setting up Facebook Login for your Cordova-based app involves a few steps. First, you need to create a Facebook Developer account and create a new app. Once you’ve done that, you can get your App ID and App Secret, which are needed for the integration. Next, you need to install the Cordova Facebook Connect plugin, which allows your app to connect to Facebook. After that, you need to configure the plugin with your App ID and App Secret. Finally, you can implement the Facebook Login functionality in your app using the plugin’s API.
What is the Cordova Facebook Connect plugin?
The Cordova Facebook Connect plugin is a tool that allows Cordova-based apps to connect to Facebook. It provides a simple API for logging in and out of Facebook, as well as for accessing Facebook’s Graph API. This plugin is essential for integrating Facebook Login into your Cordova-based app.
How do I install the Cordova Facebook Connect plugin?
You can install the Cordova Facebook Connect plugin using the Cordova command line interface (CLI). The command to install the plugin is cordova plugin add cordova-plugin-facebook-connect --save
. This will download and install the plugin into your Cordova project.
How do I configure the Cordova Facebook Connect plugin?
Configuring the Cordova Facebook Connect plugin involves setting your Facebook App ID and App Secret in the plugin’s configuration file. You can find these values in your Facebook Developer account. Once you’ve set these values, the plugin will be able to connect to your Facebook app.
How do I implement Facebook Login in my Cordova-based app?
Implementing Facebook Login in your Cordova-based app involves calling the facebookConnectPlugin.login
function provided by the Cordova Facebook Connect plugin. This function will prompt the user to log in to Facebook, and then return an access token that your app can use to make requests to the Facebook Graph API.
What is the Facebook Graph API?
The Facebook Graph API is a tool that allows apps to read and write data to Facebook. It provides access to the data stored on Facebook, such as user profiles, photos, and posts. Your app can use the Graph API to fetch information about the user who has logged in through Facebook Login.
How do I use the Facebook Graph API in my Cordova-based app?
You can use the Facebook Graph API in your Cordova-based app by making requests to the API using the access token returned by the facebookConnectPlugin.login
function. The Graph API provides a variety of endpoints that you can use to fetch different types of data.
How do I handle Facebook Login errors in my Cordova-based app?
You can handle Facebook Login errors in your Cordova-based app by providing a callback function to the facebookConnectPlugin.login
function. This callback function will be called if an error occurs during the login process. The error will be passed to the callback function as an argument, allowing you to handle it appropriately.
How do I log out of Facebook in my Cordova-based app?
You can log out of Facebook in your Cordova-based app by calling the facebookConnectPlugin.logout
function provided by the Cordova Facebook Connect plugin. This will log the user out of Facebook and invalidate the access token.
How do I test Facebook Login in my Cordova-based app?
You can test Facebook Login in your Cordova-based app by running your app on a device or emulator and attempting to log in to Facebook. You should see a Facebook Login dialog, and after logging in, your app should receive an access token. You can then use this token to make requests to the Facebook Graph API.

Narayan is a web astronaut. He is the founder of QNimate. He loves teaching. He loves to share ideas. When not coding he enjoys playing football. You will often find him at QScutter classes.