This is a good starting point for your next jQuery Plugin. I have created a skeleton example https://jsfiddle.net/jquery4u/kp8bS/ for you to check out on jsfiddle also might be of help to you.
jQuery Plugin Template Code
/*!
jQuery [name] plugin
@name jquery.[name].js
@author [author name] ([author email] or @[author twitter])
@version 1.0
@date 01/01/2013
@category jQuery Plugin
@copyright (c) 2013 [company/person name] ([company/person website])
@license Licensed under the MIT (http://www.opensource.org/licenses/mit-license.php) license.
*/
(function($){
var myPlugin, defaultOptions, __bind;
__bind = function(fn, me) {
return function() {
return fn.apply(me, arguments);
};
};
// Plugin default options.
defaultOptions = {
myvar1: 1,
myvar2: 2,
myvar3: 3
resizeDelay: 50
//etc...
};
myPlugin = (function(options) {
function myPlugin(handler, options) {
this.handler = handler;
// plugin variables.
this.resizeTimer = null;
// Extend default options.
$.extend(true, this, defaultOptions, options);
// Bind methods.
this.update = __bind(this.update, this);
this.onResize = __bind(this.onResize, this);
this.init = __bind(this.init, this);
this.clear = __bind(this.clear, this);
// Listen to resize event if requested.
if (this.autoResize) {
$(window).bind('resize.myPlugin', this.onResize);
};
};
// Method for updating the plugins options.
myPlugin.prototype.update = function(options) {
$.extend(true, this, options);
};
// This timer ensures that layout is not continuously called as window is being dragged.
myPlugin.prototype.onResize = function() {
clearTimeout(this.resizeTimer);
this.resizeTimer = setTimeout(this.resizeFunc, this.resizeDelay);
};
// Example API function.
myPlugin.prototype.resizeFunc = function() {
//...do something when window is resized
};
// Main method.
myPlugin.prototype.init = function() {
//...do something to initialise plugin
};
// Clear event listeners and time outs.
myPlugin.prototype.clear = function() {
clearTimeout(this.resizeTimer);
$(window).unbind('resize.myPlugin', this.onResize);
};
return myPlugin;
})();
$.fn.myPlugin = function(options) {
// Create a myPlugin instance if not available.
if (!this.myPluginInstance) {
this.myPluginInstance = new myPlugin(this, options || {});
} else {
this.myPluginInstance.update(options || {});
}
// Init plugin.
this.myPluginInstance.init();
// Display items (if hidden) and return jQuery object to maintain chainability.
return this.show();
};
})(jQuery);
refs:
https://raw.github.com/GBKS/Wookmark-jQuery/master/jquery.wookmark.js
https://github.com/GBKS/Wookmark-jQuery
Frequently Asked Questions (FAQs) about jQuery Plugin Template
What is the basic structure of a jQuery plugin template?
A jQuery plugin template typically starts with an immediately invoked function expression (IIFE) to create a local scope and prevent conflicts with other scripts. Inside this function, the plugin is defined as a method of the jQuery object prototype. The method usually takes an options object as a parameter, which is merged with a default options object using the jQuery extend method. The method then returns the jQuery object it was called on, to allow for method chaining. Inside the method, functionality specific to the plugin is implemented.
How can I create a basic jQuery plugin?
Creating a basic jQuery plugin involves extending the jQuery prototype object with a new method. This method defines the functionality of the plugin. Here’s a simple example:(function($) {
$.fn.myPlugin = function() {
// Plugin functionality goes here
};
})(jQuery);
In this example, myPlugin
is the name of the plugin. You can replace it with whatever you want to name your plugin.
How can I add options to my jQuery plugin?
You can add options to your jQuery plugin by passing an options object to your plugin method. You can then merge this options object with a default options object using the jQuery extend
method. Here’s an example:(function($) {
$.fn.myPlugin = function(options) {
var defaults = {
color: 'red',
size: 'medium'
};
var settings = $.extend({}, defaults, options);
// Use settings.color and settings.size in your plugin
};
})(jQuery);
In this example, the myPlugin
method takes an options object as a parameter. The extend
method is used to merge the options object with the defaults
object. The resulting settings
object is then used in the plugin.
How can I make my jQuery plugin chainable?
You can make your jQuery plugin chainable by returning the jQuery object from your plugin method. This allows you to call other jQuery methods on the same object after calling your plugin method. Here’s an example:(function($) {
$.fn.myPlugin = function() {
// Plugin functionality goes here
return this;
};
})(jQuery);
In this example, the myPlugin
method returns the jQuery object it was called on, allowing for method chaining.
How can I add private methods to my jQuery plugin?
You can add private methods to your jQuery plugin by defining them inside your plugin method. These methods will not be accessible outside the plugin method. Here’s an example:(function($) {
$.fn.myPlugin = function() {
function privateMethod() {
// Private method functionality goes here
}
// Call privateMethod from within myPlugin
privateMethod();
};
})(jQuery);
In this example, the privateMethod
function is defined inside the myPlugin
method. It can be called from within the myPlugin
method, but not from outside it.
How can I add public methods to my jQuery plugin?
You can add public methods to your jQuery plugin by attaching them to the jQuery object inside your plugin method. These methods will be accessible outside the plugin method. Here’s an example:(function($) {
$.fn.myPlugin = function() {
this.publicMethod = function() {
// Public method functionality goes here
};
return this;
};
})(jQuery);
In this example, the publicMethod
function is attached to the jQuery object inside the myPlugin
method. It can be called from outside the myPlugin
method.
How can I handle events in my jQuery plugin?
You can handle events in your jQuery plugin by using the jQuery on
method inside your plugin method. This allows you to bind event handlers to elements selected by your plugin. Here’s an example:(function($) {
$.fn.myPlugin = function() {
this.on('click', function() {
// Handle click event here
});
return this;
};
})(jQuery);
In this example, a click event handler is bound to the elements selected by the myPlugin
method.
How can I access the elements selected by my jQuery plugin?
You can access the elements selected by your jQuery plugin by using the this
keyword inside your plugin method. This refers to the jQuery object the plugin method was called on, which contains the selected elements. Here’s an example:(function($) {
$.fn.myPlugin = function() {
this.each(function() {
// Access each selected element here
});
return this;
};
})(jQuery);
In this example, the each
method is used to iterate over the elements selected by the myPlugin
method.
How can I maintain the state of my jQuery plugin?
You can maintain the state of your jQuery plugin by using data attributes. The jQuery data
method allows you to attach data to elements, which can be used to store the state of your plugin. Here’s an example:(function($) {
$.fn.myPlugin = function() {
this.data('myPluginState', 'active');
// Access the state later with this.data('myPluginState')
return this;
};
})(jQuery);
In this example, the data
method is used to attach a myPluginState
attribute to the elements selected by the myPlugin
method.
How can I destroy my jQuery plugin?
You can destroy your jQuery plugin by defining a destroy method that removes any modifications made by your plugin. This might involve unbinding event handlers, removing data attributes, and reverting any changes to the DOM. Here’s an example:(function($) {
$.fn.myPlugin = function() {
this.destroy = function() {
// Destroy plugin functionality goes here
};
return this;
};
})(jQuery);
In this example, a destroy
method is defined that can be called to destroy the myPlugin
plugin.
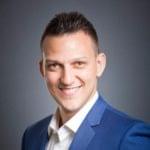
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.