Design a Multi-Page Form in WordPress: Introduction
Key Takeaways
- Custom multi-page forms in WordPress can be created from scratch using basic PHP and HTML form knowledge. These forms use the POST method to pass information from the form back to itself and IF statements in PHP to display the next part of the form.
- Multi-page forms are useful for collecting information in stages and can be conditionally or dynamically generated based on user responses. This can help reduce form abandonment and collect critical data about user behavior.
- The process of creating a multi-page form involves setting up a shortcode, creating a new post or page for the form, and creating form elements. The form data can be stored within a database table and displayed on the next page of the form.
Have you ever found yourself needing to create a custom, multiple-page form in WordPress? I do… all the time! While you can use a variety of plugins to get the job done, they’re rarely perfect for your purposes. I’ve found that the easiest way for me to get everything right — from the forms to the data to the reporting — is to simply create these from scratch myself.
In this series of articles, I will walk you through the process of creating multiple page forms from scratch. Believe it or not, it’s fairly straightforward if you understand some basic PHP and know your HTML form basics. (If you’re a PHP veteran or interested in becoming one, you should head over to our PHP partner site, PHPMaster.com)
We’ll be using the POST method to pass information from the form back to itself and IF statements in PHP to display the next part of the form. As the users submit form data, the page will update with the next page of the form, storing the submitted information as it goes.
The result is that users stay on the same page, and you can conditionally — even dynamically — generate the next page of the form based upon their answers. Or, you could just keep the form fields static for simplicity’s sake.
Why a Multi-Page Form?
There are lots of reasons why you might need a multi-page form. In some cases, you might not want more than 3-5 questions per page. In other cases, you might want to only collect additional information based upon their initial responses. For example, if the user indicates that they’re under 18, you may not want to ask certain questions, or you might want to display a different form based upon male or female respondents.
Further, a multiple-page form allows you to collect information in stages. If users abandon the questionnaire for whatever reason, you’ve collected at least some information that might be useful. Form abandonment is common, and multi-page form collection gives you critical data, such as where forms get abandoned most often, basic contact info from the user for follow-up, etc.
Case Study
For planning purposes, I’ll be collecting basic information on users and their shopping preferences. So, here’s how our form will progress:
- Page 1 – Basic contact info
- Name (first and last),
- Email,
- Phone,
- Zip code
- Page 2 – Socioeconomic data
- Gender
- Age
- Education
- Income
- Page 3 – Shopping Preferences
- Location (online, store)
- Favorite Categories
- Page 4 – “Thank You!” landing page
I’ll be setting this up in our functions.php file, which is fine for testing, but when you’re ready to make this live on your site, you should consider making this a plugin or at a minumum separating this code from your functions.php file and using an include_once statement to link the files in your functions.php doc.
Part of my rationale for this method is to avoid having to delve into FTP and other less pertinent methods of creating custom functionality in WordPress. So, for you veterans, just bear with my less-than-ideal methods.
Step 1: Set up a Shortcode
This is optional, but it sure makes it easier for me to use shortcodes to display these multi-page forms. So, that’s the method I’ll teach, and you can implement your own if you prefer.
In your functions.php file, add the following:
[sourcecode language=”php”]
add_shortcode(‘multipage_form_sc’,’multipage_form’);
function multipage_form(){
echo ‘<h3>New Form</h3>’;
};
[/sourcecode]
This lets WordPress know that you can put the shortcode multipage_form_sc into a post or page, and that WordPress should execute the function multipage_form when it finds the shortcode. Because I often have several multi-page forms that I’ll use for a given site, I give these forms unique names, for example, multipage_form_userinfo_sc and its corresponding function multipage_form_userinfo.
Step 2: Set up a Post or Page
Create the new post or page on which you want your multiple-page form to exist. Insert the shortcode into the form, publish it, and view the page. You should see the “New Form” message we created in our multipage_form() function.
Here’s what your shortcode should look like within your post/page editor:
[multipage_form_sc]
Step 3: First Form Elements
Let’s jump into creating the first form elements. I will be keeping this incredibly simple for the sake of focusing on the concepts of multi-page forms, not CSS or other eye-candy that we can delve into later.
So, here’s our basic information you can paste into your multipage_form() function:
[sourcecode language=”php”]
add_shortcode(‘multipage_form_sc’,’multipage_form’);
function multipage_form(){
global $wpdb;
$this_page = $_SERVER[‘REQUEST_URI’];
$page = $_POST[‘page’];
if ( $page == NULL ) {
echo ‘<form method=”post” action=”‘ . $this_page .'”>
<label for=”first_name” id=”first_name”>First Name: </label>
<input type=”text” name=”first_name” id=”first_name” />
<label for=”last_name” id=”last_name”>Last Name: </label>
<input type=”text” name=”last_name” id=”last_name” />
<label for=”email” id=”email”>Email: </label>
<input type=”text” name=”email” id=”email” />
<label for=”phone” id=”phone”>Phone: </label>
<input type=”text” name=”phone” id=”phone” />
<label for=”first_name” id=”first_name”>Zip Code: </label>
<input type=”text” name=”zip_code” id=”zip_code” />
<input type=”hidden” value=”1″ name=”page” />
<input type=”submit” />
</form>’;
}//End Page 1 of Form
elseif ( $page == 1 ) {
$first_name = $_POST[‘first_name’];
$last_name = $_POST[‘last_name’];
$email = $_POST[’email’];
$phone = $_POST[‘phone’];
$zip_code = $_POST[‘zip_code’];
echo ‘<h3>You made it to the 2nd page!</h3>
<p>Here are your form inputs: </p>
<p>First Name: ‘ . $first_name . ‘</p>
<p>Last Name: ‘ . $last_name . ‘</p>
<p>Email: ‘ . $email . ‘</p>
<p>Phone: ‘ . $phone . ‘</p>
<p>Zip Code: ‘ . $zip_code . ‘</p>’;
}//End Page 2 of Form
};
[/sourcecode]
That’s a lot of code, so let’s hit the highlights.
First, we have our initial POST statements we’re looking for — $this_page is the page we’re currently on for our form handling, and the $page is the page number. I’m using “1” for page one, “2” for page two, etc. Once we store this information, we’ll have a record of the page number completed. We’ll also use the page number to test which page of the form we’re on and display information accordingly.
Next, we have our first IF statement that tests which page number we’re on. If it’s blank (NULL), we display the first page of the form. That’s where we have our basic form elements — labels and inputs. I included the hidden form value for the page number, but you can also add all kinds of extra hidden form fields to collect the username (if they’re logged in), as well as other information you may want.
After that, we have page two information starting with the ELSEIF statement. We’ll continue down this path of ELSEIF statements for form data handling and display. You can use the SWITCH statement if you have many, many pages, but the IF format works just fine for us in this scenario.
On page two, we grab the form inputs and display them for testing purposes. In the next article, we will actually store this information within a database table and display the next page of the form.
Try it Out
Save your functions.php file with the above code (don’t miss anything; a single misplaced semi-colon or brace will crash your site!) Go to the post or page you created in Step 2, fill out page one of the form, and click the “Submit” button. Voila!
Coming Up Next!
In the next installment of this series, we’re going to jump into phpMyAdmin and create a table in the database to start storing all this wonderful information we’re collecting. Don’t worry, I’ll keep it very basic and will even write the MySQL scripts for you if you’re brand new to that side of things.
Did you know that we have an entire website dedicated to PHP mastery? Check out our partner site at PHPMaster.com.
Frequently Asked Questions about Designing a Multi-Page Form in WordPress
What are the benefits of using a multi-page form in WordPress?
Multi-page forms in WordPress offer several benefits. They help to improve user experience by breaking down long forms into manageable sections, reducing the intimidation factor and making it easier for users to complete. They also help to increase conversion rates as users are more likely to complete shorter forms. Additionally, multi-page forms allow for better data management and analysis as you can segment and analyze data based on different sections of the form.
Can I create a multi-page form without coding knowledge?
Yes, you can create a multi-page form in WordPress without any coding knowledge. There are several plugins available, such as WPForms and Multi-Step Form, that provide a user-friendly interface for creating multi-page forms. These plugins offer drag-and-drop form builders, making it easy for anyone to create complex forms.
How can I customize the design of my multi-page form?
Most WordPress form plugins offer customization options that allow you to change the design of your form. You can customize the color scheme, font style, button design, and more. Some plugins also offer advanced customization options through CSS for more experienced users.
Can I add conditional logic to my multi-page form?
Yes, many WordPress form plugins support conditional logic. This allows you to create dynamic forms that change based on user input. For example, you can show or hide certain fields or pages based on the answers provided by the user.
How can I track the performance of my multi-page form?
You can track the performance of your multi-page form using various analytics tools. Most form plugins integrate with Google Analytics, allowing you to track form views, submissions, and conversion rates. Some plugins also offer built-in analytics features.
Can I save user progress on my multi-page form?
Yes, some WordPress form plugins offer the ability to save user progress. This allows users to save their progress and continue filling out the form at a later time. This feature can be particularly useful for long forms.
How can I prevent spam submissions on my multi-page form?
There are several ways to prevent spam submissions on your multi-page form. Most form plugins offer built-in spam protection features such as CAPTCHA and honeypot fields. You can also use third-party anti-spam plugins for additional protection.
Can I integrate my multi-page form with other tools and services?
Yes, most WordPress form plugins support integration with various tools and services. You can integrate your form with email marketing services, CRM software, payment gateways, and more. This allows you to automate various tasks and streamline your workflow.
How can I test my multi-page form before publishing it?
Most form plugins offer a preview feature that allows you to test your form before publishing it. You can fill out the form as a user would to ensure that everything works correctly. It’s also a good idea to test your form on different devices and browsers to ensure compatibility.
Can I export or import form data in WordPress?
Yes, most WordPress form plugins support data export and import. You can export form submissions to a CSV file for further analysis or import data from other forms or sources. This can be particularly useful for migrating data or creating backup copies of your form data.
When he's not being a complete goofball, “they” drag Justyn into the office where he pretends to be a Senior Editor and Content Engineer at Creative Content Experts — a content marketing firm out of NW Arkansas. He has 10+ years’ experience in technical writing and geek-related fields. He loves WordPress, coffee, and peanut butter a little too much.
Published in
·Cloud·Debugging & Deployment·Development Environment·PHP·Programming·Web·April 26, 2014
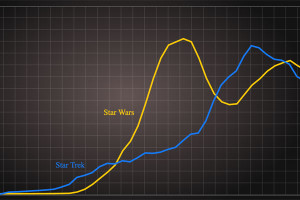
Published in
·Canvas & SVG·Design·Design & UX·HTML·HTML & CSS·Illustration·Usability·Web·May 26, 2016