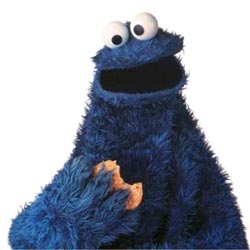
window.name = "This message will survive between page loads.";
Now add the following code to any other page:
alert(window.name);
Navigate from the first page to the second and you’ll find that the message data is retained.
As normal, there are a number of caveats:
- The window.name property can be analysed and changed if you navigate to page on another website. That’s easily thwarted by not providing external links within your application’s main window. However, to be on the safe side, don’t use window.name for storing secure data (unlikely to be a major problem for a client-side-only temporary data store).
- window.name can only store strings. What if we need to save other data types or even complex objects? Serialization is the answer and, fortunately, we have already developed cross-browser code to generate JSON strings from any JavaScript object.
Frequently Asked Questions (FAQs) about Cookieless JavaScript Session Variables
What are the benefits of using cookieless sessions in JavaScript?
Cookieless sessions in JavaScript offer several benefits. Firstly, they can enhance the user experience by maintaining state information across multiple pages without the need for cookies. This is particularly useful for users who have disabled cookies in their browser settings. Secondly, cookieless sessions can improve the security of your web application by reducing the risk of session hijacking through cookie theft. Lastly, they can also help to ensure compliance with privacy regulations that restrict the use of cookies.
How can I implement cookieless sessions in JavaScript?
Implementing cookieless sessions in JavaScript involves storing session data on the server side and associating it with a unique session ID. This session ID can be passed between the client and server via the URL or a hidden form field. On the server side, you can use a session management library or framework to handle the creation, storage, and retrieval of session data.
Can I use sessionStorage for cookieless sessions in JavaScript?
Yes, the sessionStorage object in JavaScript provides a way to store session data on the client side without using cookies. However, it’s important to note that data stored in sessionStorage is only available for the duration of the page session and is deleted when the tab is closed. Also, sessionStorage does not provide any built-in mechanism for associating session data with a unique session ID.
What are the security implications of using cookieless sessions?
While cookieless sessions can reduce the risk of session hijacking through cookie theft, they can potentially expose session IDs in the URL, which could be captured by malicious actors. To mitigate this risk, it’s important to use secure communication protocols such as HTTPS and to implement measures to prevent session fixation attacks, such as regenerating the session ID after login.
How can I test the security of cookieless sessions?
Testing the security of cookieless sessions involves checking for vulnerabilities such as session fixation and session sidejacking. Tools like OWASP ZAP and Burp Suite can be used to perform these tests. It’s also important to review your application’s session management code to ensure that it follows best practices for secure session management.
How do cookieless sessions work in ASP.NET?
In ASP.NET, cookieless sessions work by embedding the session ID in the URL. This is done by setting the cookieless attribute of the sessionState configuration element to true in the Web.config file. The ASP.NET session state module then automatically handles the creation, storage, and retrieval of session data.
Can I access session variables in JavaScript?
Yes, you can access session variables in JavaScript using the sessionStorage object. However, this only works for data stored on the client side. To access server-side session data in JavaScript, you would need to use AJAX or a similar technique to make a request to the server.
What are the alternatives to cookieless sessions?
Alternatives to cookieless sessions include using cookies, local storage, and IndexedDB for client-side session management. On the server side, you can use database-backed sessions or distributed session stores like Redis or Memcached.
How do cookieless sessions compare to cookie-based sessions?
Cookieless sessions and cookie-based sessions each have their pros and cons. Cookieless sessions can provide a better user experience for users who have disabled cookies and can improve security by reducing the risk of session hijacking. However, they can potentially expose session IDs in the URL. Cookie-based sessions, on the other hand, are easier to implement and do not expose session IDs, but they can be vulnerable to cookie theft and are affected by the user’s cookie settings.
Can I use cookieless sessions with frameworks like Vue or React?
Yes, you can use cookieless sessions with JavaScript frameworks like Vue or React. However, these frameworks do not provide built-in support for cookieless sessions, so you would need to implement it yourself or use a third-party library.
Craig is a freelance UK web consultant who built his first page for IE2.0 in 1995. Since that time he's been advocating standards, accessibility, and best-practice HTML5 techniques. He's created enterprise specifications, websites and online applications for companies and organisations including the UK Parliament, the European Parliament, the Department of Energy & Climate Change, Microsoft, and more. He's written more than 1,000 articles for SitePoint and you can find him @craigbuckler.