- How Many People Are Using WebGL?
- What is WebGL Doing and Why Should I Care?
- Asm.js and Emscripten
- Exporting a Unity Game to WebGL with Asm.js
- WebGL Showcase
- How Do I Get Started?
- Engines and Frameworks
- Will It Work on Consoles?
- Podcasts
- Looking to Learn More?
- More Hands-on with Web Development
- Frequently Asked Questions (FAQs) about WebGL
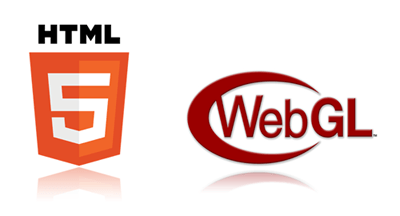
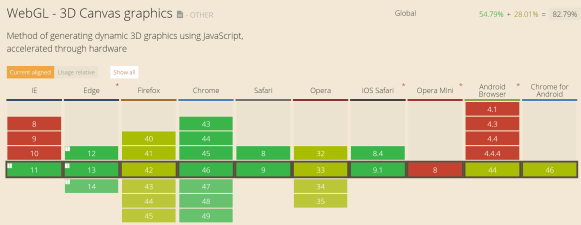
How Many People Are Using WebGL?
User pyalot on reddit has pointed me towards an excellent site for tracking WebGL usage and statistics: https://webglstats.com/. WebGL Stats uses a tracker frame embedded in other sites to collect the data. You can help too, just embed the code below into your page.
<script src="//cdn.webglstats.com" defer="defer" async="async"></script>
What is WebGL Doing and Why Should I Care?
WebGL has three distinct advantages over writing code that simply manipulates the DOM:- Tasks. Drawing reflective materials or complex lighting generate a ton of overhead, and seeing that JavaScript is single threaded and CPU bound, why not offload some of that to the GPU in your device and have that do the heavy lifting?
- Performance.Utilizing hardware acceleration (the GPU built into your device), WebGL is a great fit for games or complex visualizations.
- Shaders.Complex visual effects can be produced with small programs known as “shaders”. This may be as simple as producing a sepia coloring effect, or more complex simulations such as water or flames. Visit Shadertoy for a showcase of some examples which really highlight this.
Asm.js and Emscripten

Exporting a Unity Game to WebGL with Asm.js
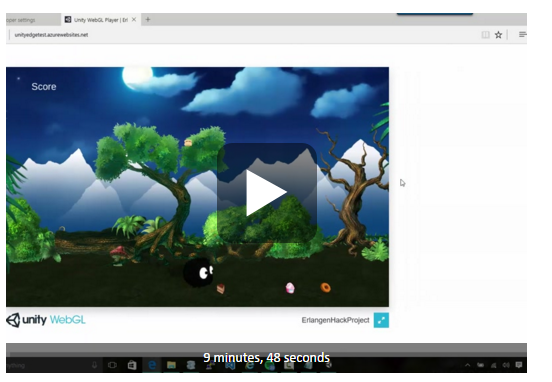
WebGL Showcase
Score Rush – Xona Games

Dolby Audio Room
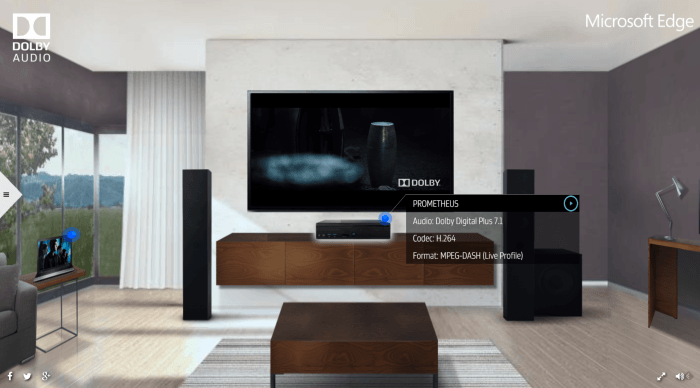
World Flights
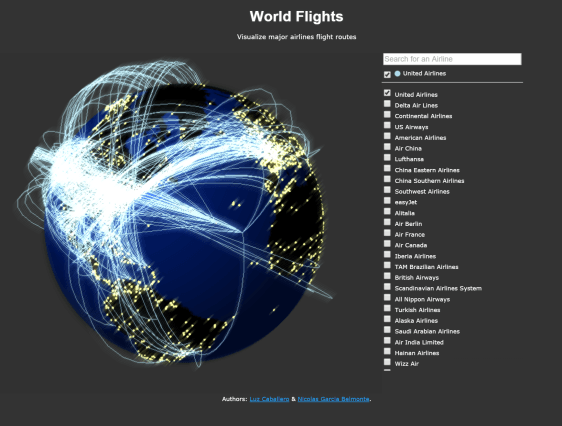
Monopoly – PlayCanvas
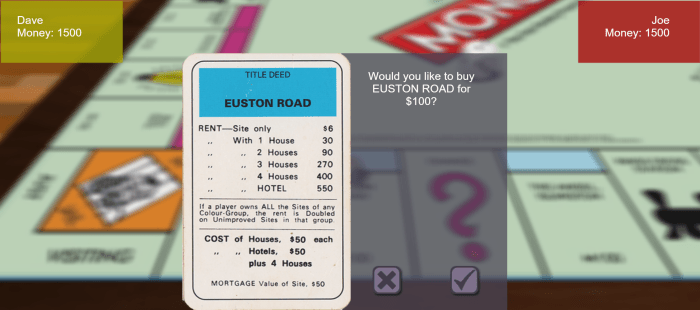
WebGlSamples.org
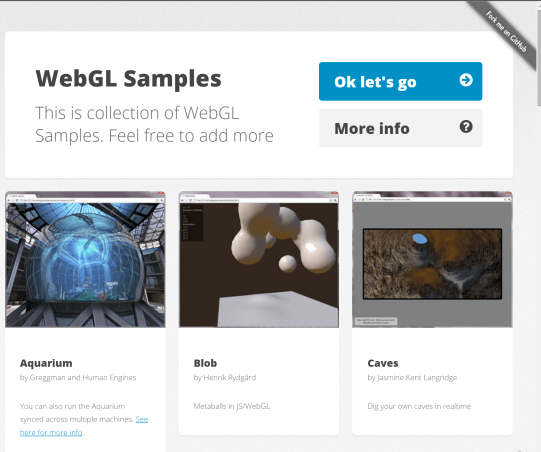
How Do I Get Started?
Here are the essentials steps to create create your first WebGL project:- Create <canvas> element
- Obtain drawing context
- Initialize viewport
- Create buffers
- Create matrices
- Create shaders
- Initialize shaders
- Draw primitives
Engines and Frameworks
BabylonJS
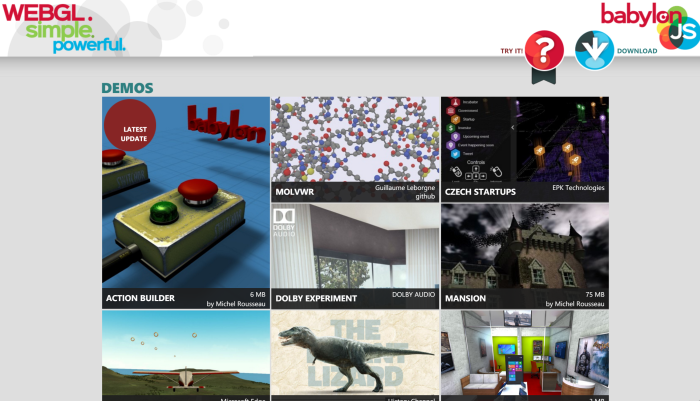
PlayCanvas
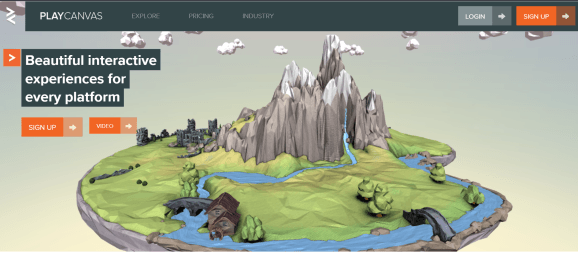
Turbulenz
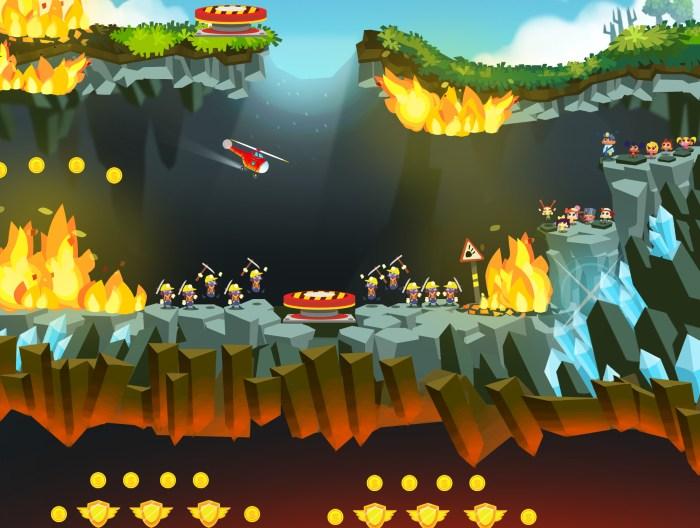
Pixi.js
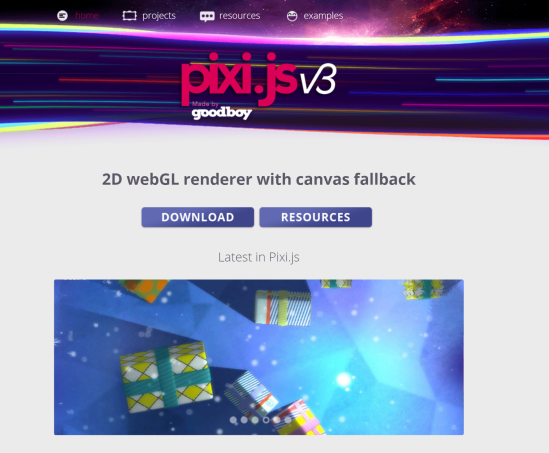
Phaser.io
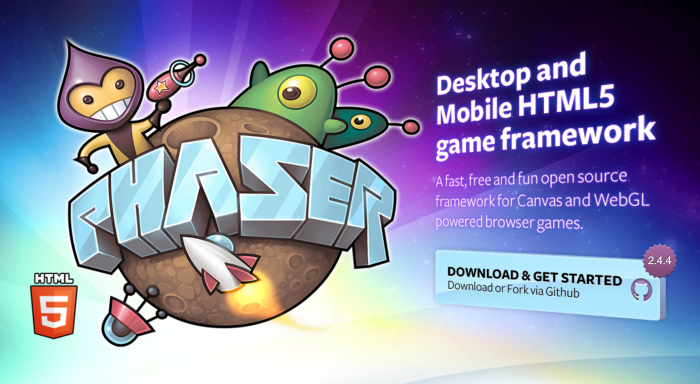
Construct 2
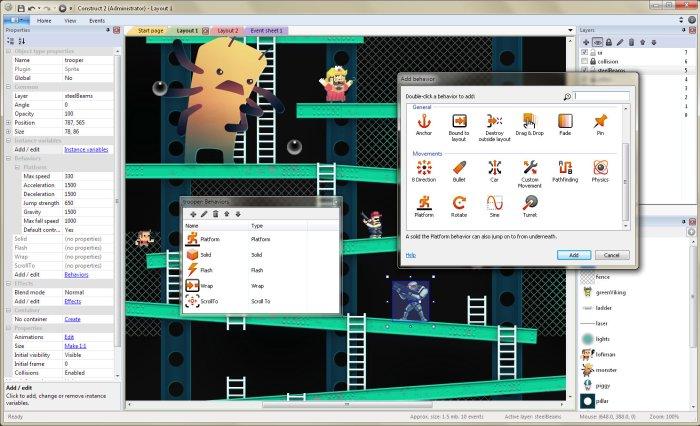
Three.js
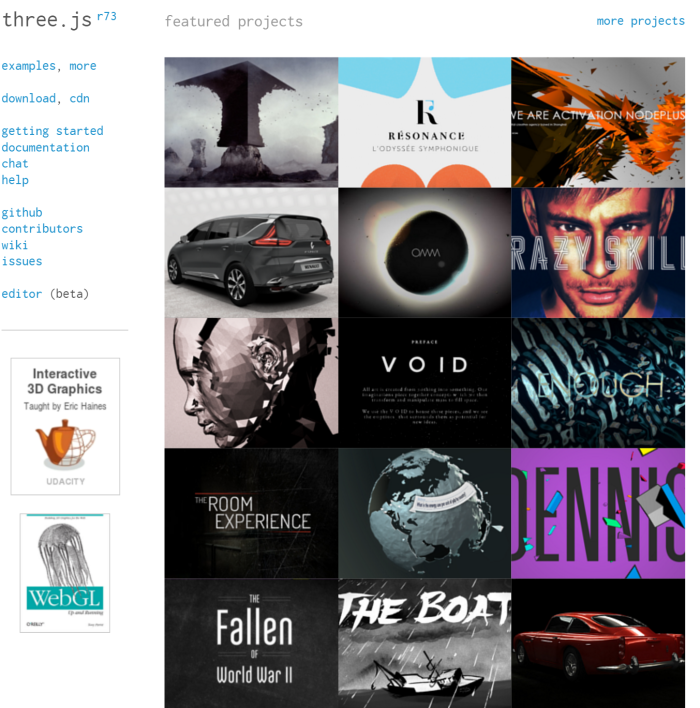
PhiloGL
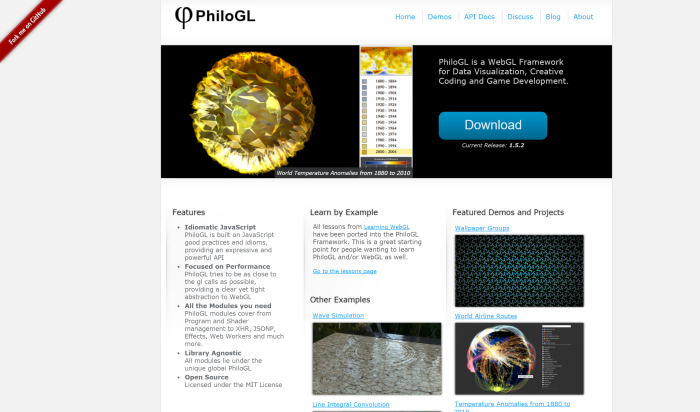
Will It Work on Consoles?
David Rousset (@davrous), a Program Manager at Microsoft who is working with Edge, had a great blog post this week where he showcased BabylonJS running on an Xbox One. As of November 12th, a new update is available on Xbox One. As a web developer, I’m more than happy to now have Microsoft Edge running on my console! This means that you can now run very modern content inside the Xbox One browser! You can also remote debug the application using VorlonJS. Vorlon.js is an open-source cross-platforms remote debugging tool that has been made to easily remote debug any web page running on any device. WebGL also works on the Playstation 4. In fact, their UX is largely powered by WebGL. From Don Olmstead, one of the head architects at Sony:When you login to your PS4 you are running #WebGL code. The PlayStation Store, the Music and Video Applications, as well as a good chunk of UX are all rendered within the browser. I spent a good amount of time tuning our WebGL rendering engine, and I will be speaking at+SFHTML5 about how to optimize WebGL usage within the context of that work. There will be plenty of great tips on how you can speed up your own WebGL applications so get your slot now.This talk from Google Developers offers a great overview of how it works on their platform. Silicon Angle also has a great article on the topic.
Podcasts
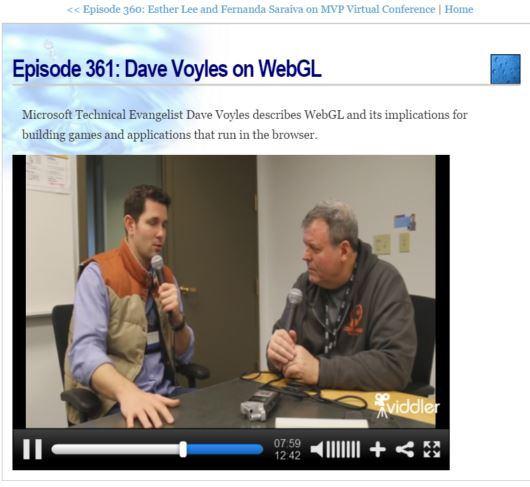
Looking to Learn More?
Here are some of the better resources I’ve found on the internet for learning WebGL:- WebGL Academy
- WebGL fundamentals
- LearningWebGL.com
More Hands-on with Web Development
This article is part of the web development series from Microsoft evangelists and engineers on practical JavaScript learning, open source projects, and interoperability best practices including Microsoft Edge browser and the new EdgeHTML rendering engine. We encourage you to test across browsers and devices including Microsoft Edge – the default browser for Windows 10 – with free tools on dev.microsoftedge.com:- Scan your site for out-of-date libraries, layout issues, and accessibility
- Download free virtual machines for Mac, Linux, and Windows
- Check Web Platform status across browsers including the Microsoft Edge roadmap
- Remotely test for Microsoft Edge on your own device
- Interoperability best practices (series):
- How to avoid Browser Detection
- Using CSS Prefix best practices
- Keeping your JS frameworks & libs updated
- Building plug-in free web experiences
- Coding Lab on GitHub: Cross-browser testing and best practices
- Woah, I can test Edge & IE on a Mac & Linux! (from Rey Bango)
- Advancing JavaScript without Breaking the Web (from Christian Heilmann)
- Unleash 3D rendering with WebGL (from David Catuhe)
- Hosted web apps and web platform innovations (from Kiril Seksenov)
- vorlon.JS (cross-device remote JavaScript testing)
- manifoldJS (deploy cross-platform hosted web apps)
- babylonJS (3D graphics made easy)
- Visual Studio Code (lightweight code-editor for Mac, Linux, or Windows)
- Visual Studio Dev Essentials (free, subscription-based training and cloud benefits)
Frequently Asked Questions (FAQs) about WebGL
What is the difference between WebGL and other 3D graphics APIs?
WebGL is a JavaScript API for rendering interactive 3D and 2D graphics within any compatible web browser without the use of plug-ins. Unlike other 3D graphics APIs, WebGL is integrated directly into the web environment, allowing full integration with other web standards. This means you can combine 3D graphics with text, images, and other HTML elements on your web page. WebGL is also based on OpenGL ES 2.0, making it familiar to many developers who have worked with 3D graphics before.
How can I check if my browser supports WebGL?
You can check if your browser supports WebGL by visiting the website get.webgl.org. If your browser supports WebGL, you will see a spinning cube. If not, you will be given instructions on how to enable it or update your browser. You can also check the WebGL Report website for a detailed report of your browser’s WebGL capabilities.
Why is my WebGL application running slowly?
There could be several reasons why your WebGL application is running slowly. It could be due to inefficient rendering, such as drawing too many objects or using complex shaders. It could also be due to memory issues, such as using too much memory for textures or buffers. To improve performance, try to optimize your rendering, reduce memory usage, and use WebGL extensions when available.
Can I use WebGL on mobile devices?
Yes, WebGL is supported on many modern mobile devices. However, the performance and features available may vary depending on the device and the web browser. To ensure the best compatibility and performance, it is recommended to test your WebGL application on multiple devices and browsers.
How can I learn WebGL?
There are many resources available to learn WebGL. You can start with the WebGL specification on the Khronos Group website, which provides a detailed description of the API. There are also many tutorials and examples available online, such as the ones on the Mozilla Developer Network and WebGL Fundamentals websites. Additionally, there are several books and online courses that cover WebGL in depth.
What are the security implications of using WebGL?
Like any web technology, WebGL has potential security risks. These include the possibility of executing malicious code or accessing sensitive information on the user’s system. However, web browsers implement several security measures to mitigate these risks, such as the same-origin policy and context isolation. It is also recommended to keep your web browser up-to-date to benefit from the latest security updates.
Can I use WebGL with other web technologies?
Yes, WebGL can be used in combination with other web technologies, such as HTML, CSS, and JavaScript. This allows you to create rich and interactive web applications that combine 3D graphics with other web content. For example, you can use WebGL to render 3D models in a canvas element, and then use CSS and JavaScript to control the appearance and behavior of the models.
How can I debug WebGL applications?
Debugging WebGL applications can be challenging due to the low-level nature of the API. However, there are several tools and techniques that can help. For example, you can use the WebGL Inspector extension for Chrome, which provides a variety of debugging features. You can also use the WebGLRenderingContext.getShaderInfoLog and WebGLRenderingContext.getProgramInfoLog methods to get information about shader compilation and linking errors.
What are the limitations of WebGL?
While WebGL is a powerful tool for creating 3D graphics on the web, it does have some limitations. These include limited support for older browsers and devices, performance and memory limitations, and lack of support for some advanced graphics features. However, many of these limitations can be overcome with careful programming and use of WebGL extensions.
What is the future of WebGL?
The future of WebGL looks promising. The API is continuously being improved and extended, with new features and extensions being added regularly. There is also a growing community of developers and artists who are creating amazing WebGL applications and sharing their knowledge and techniques. Furthermore, the upcoming WebGPU standard promises to bring even more power and flexibility to web-based graphics.

Dave Voyles is a Technical Evangelist for Microsoft. He spends a lot of time writing games, writing about games, and writing about how to write games for the game dev community, Read his blog or follow him on Twitter.