Keep up to date on current trends and technologies
Mobile - Swift
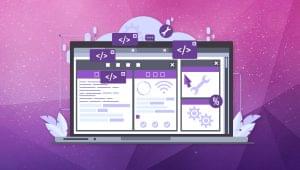
Top Mobile App Development Programming Languages
Lucero del Alba
Dependency Management with the Swift Package Manager
Chris Ward
The Past, Present and Future of Swift
Chris Ward
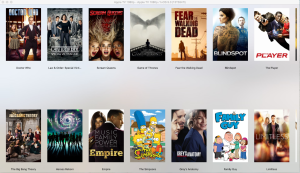
Coding for the Big Screen with the Apple tvOS SDK
Patrick Haralabidis
Extending an iOS App with WatchKit
Brett Romero
Showing 5 of 5