I spend a lot of my day in the terminal, and my shell of choice is Zsh — a highly customizable Unix shell that packs some very powerful features. As I’m a lazy developerTM, I’m always looking for ways to type less and to automate all the things. Luckily this is something that Zsh lends itself well to.
In this post, I’m going to share with you 75 commands, plugins, aliases and tools that will hopefully save you some keystrokes and make you more productive in your day-to-day work.
If you don’t have Zsh installed on your machine, then check out this post, where I show you how to get up and running.
15 Things Zsh Can Do out of the Box
Zsh shares a lot of handy features with Bash. None of the following are unique to Zsh, but they’re good to know nonetheless. I encourage you to start using the command line to perform operations such as those listed below. It might seem like more work than using a GUI at first, but once you get the hang of things, you’ll never look back.
- Entering
cd
from anywhere on the file system will bring you straight back to your home directory. - Entering
!!
will bring up the last command. This is handy if a command fails because it needs admin rights. In this case you can typesudo !!
. - You can use
&&
to chain multiple commands. For example,mkdir project && cd project && npm init -y
. - Conditional execution is possible using
||
. For example,git commit -m "whatever..." || echo "Commit failed"
. - Using a
-p
switch with themkdir
command will allow you to create parent directories as needed. Using brace expansion reduces repetition. For example,mkdir -p articles/jim/sitepoint/article{1,2,3}
. - Set environment variables on a per-command basis like so:
NODE_DEBUG=myapp node index.js
. Or, on a per-session basis like so:export NODE_DEBUG=myapp
. You can check it was set by typingecho $<variable-name>
. - Pipe the output of one command into a second command. For example,
cat /var/log/kern.log | less
to make a long log readable, orhistory | grep ssh
to search for any history entries containing “ssh”. - You can open files in your editor from the terminal. For example,
nano ~/.zshrc
(nano),subl ~/.zshrc
(Sublime Text),code ~/.zshrc
(VS Code). If the file doesn’t exist, it will be created when you press Save in the editor. - Navigation is an important skill to master. Don’t just rely on your arrow keys. For example, Ctrl + a will take you to the beginning of a line.
- Whereas Ctrl + e will take you to the end.
- You can use Ctrl + w to delete one word (backwards).
- Ctrl + u will remove everything from the cursor to the beginning of the line.
- Ctrl + k will clear everything from the cursor to the end of the line. These last three can be undone with Ctrl + y.
- You can copy text with Ctrl + Shift + c. This is much more elegant than right clicking and selecting Copy.
- Conversely, you can paste copied text with Ctrl + shift + v.
Try to commit those key combos to memory. You’ll be surprised at how often they come in handy.
15 Custom Aliases to Boost Your Productivity
Aliases are terminal shortcuts for regular commands. You can add them to your ~/.zshrc
file, then reload your terminal (using source ~/.zshrc
) for them to take effect.
The syntax for declaring a (simple) alias is as follows:
alias [alias-name]='[command]'
Aliases are great for often-used commands, long commands, or commands with a hard-to-remember syntax. Here are some of the ones I use on a regular basis:
- A
myip
alias, which prints your current public IP address to the terminal:alias myip='curl http://ipecho.net/plain; echo'
. - A
distro
alias to output information about your Linux distribution:alias distro='cat /etc/*-release'
. - A
reload
alias, as I can never seem to remember how to reload my terminal:alias reload='source ~/.zshrc'
. - An
undo-git-reset
alias:alias undo-git-reset-head="git reset 'HEAD@{1}'"
. This reverts the effects of runninggit reset HEAD~
. - An alias to update package lists:
alias sapu='sudo apt-get update'
. - An alias to rerun the previous command with
sudo
:alias ffs='sudo !!'
. - Because I’m lazy, I have aliased
y
to theyarn
command:alias y='yarn'
. This means I can clone a repo, then just type y to pull in all the dependencies. I learned this one from Scott Tolinski on Syntax. - Not one of the ones I use, but this alias blows away the
node_modules
folder and removes thepackage-lock.json
file, before reinstalling a project’s dependencies:alias yolo='rm -rf node_modules/ && rm package-lock.json && yarn install'
. As you probably know, yolo stands for You Only Live Once. - An alias to open my
.zshrc
file for editing:alias zshconfig='subl $HOME/.zshrc'
. - An alias to update the list of Ruby versions rbenv can install:
alias update-available-rubies='cd ~/.rbenv/plugins/ruby-build && git pull'
- An alias to kick off a server in your current directory (no npm packages required):
alias server='python -m SimpleHTTPServer 8000'
. - You can also create an alias to open documentation in your browser:
alias npmhelp='firefox https://github.com/robbyrussell/oh-my-zsh/tree/master/plugins/npm'
. - A global alias to pipe a command’s output to
less
:alias -g L='| less'
. You can use it like so:cat production.log L
. - A global alias to pipe a command’s output to
grep
:alias -g G='| grep'
. You can use it like so:history G ssh
. - You can also use functions to create aliases. The following (taken from here) creates an alias that adds, commits, and pushes code to GitHub:
bash
function acp() {
git add .
git commit -m "$1"
git push
}
There are lots of places to find more ideas for aliases online. For example, this Hacker News discussion, or this post on command line productivity with Zsh.
15 Cool Things You Can Do with (Oh My) Zsh
Oh My Zsh is a community-driven framework for managing your Zsh configuration and comes bundled with thousands of helpful functions, helpers, plugins and themes. If you’re going to make the Z shell your daily driver, you should really install Oh My Zsh.
Here are fifteen useful things Oh My Zsh can do for you:
- The
take
command will create a new directory and change into it.take my-project
replacesmkdir my-project && cd my-project
. zsh_stats
will give you a list of the top 20 commands and how many times they’ve been run.- Oh My Zsh simplifies navigating your file system. For example,
..
is an alias forcd ..
. - In the same way,
...
moves you up two directories,....
moves you up three, and.....
moves you up four. - You can omit the
cd
when navigating. Typing/
, for example, will take you straight to your filesystem root. - Partial matching is also supported. For example, typing
/h/j/De
and pressing TAB, then Return, takes me to/home/jim/Desktop/
. rd
is an alias forrmdir
andmd
is an alias formkdir -p
.- You can type
d
to list the last used directories from a terminal session. - You can then navigate to any of these using
cd -n
, wheren
is the directory number. - Tab completion is another great feature. For example, typing
ls -
and pressing TAB will list all of the command’s options, along with a helpful description of what they do. This also works forcap
,rake
,ssh
, andkill
. - Typing
alias
lists all of your current aliases. - With globbing (a Zsh feature), you can list files with a particular extension. For example,
ls *.html
will list all HTML files in the current directory. To include subdirectories, change to:ls **/*.html
. - Glob qualifiers allow you to select types of files by using flags. For example,
ls -l **/*(.x)
will find all executable files in the current directory and all sub-directories. - You can search for files by date modified. For example,
ls *(m-7)
will list all files modified within the last week. - You can search for files by size. For example,
ls *(Lm+1)
will find all files with a size larger than 1MB.
Using Plugins for Fun and Profit
Oh My Zsh ships with a lot of plugins. You should look through these and invest some time learning those that will help your workflow.
Here are three plugins I regularly use, that provide a ton of handy shortcuts and aliases.
10 Nifty Git Aliases
The git plugin provides many aliases and several useful functions. Why not go through these and attempt to memorize your top ten? Here are the ones I use most often.
g
is a handy alias forgit
. This means you can type things likeg clone <whatever>
instead ofgit clone <whatever>
. Might only be two keystrokes, but they soon add up.gaa
is an alias forgit add all
. I use this one all the time.gb
is an alias forgit branch
, which will list all of the branches in the current repo and show you which one you’re on.gcb
is an alias forgit checkout -b
, the command that allows you to create a new branch.gcm
is an alias forgit checkout master
. This returns you to the master branch.gdca
is an alias forgit diff --cached
. This allows you to diff any files you’ve staged for commit.gf
is an alias forgit fetch
.gm
is an alias forgit merge
.gp
is an alias forgit push
. To sync a fork of a repo, you could do:gf upstream
,gm upstream/master
, followed bygp
.glog
is an alias forgit log --oneline --decorate --graph
, which will give you a pretty git branch graph.
10 Handy npm Aliases
The npm plugin provides completion as well a bunch of useful aliases.
npmg
is an alias fornpm install --global
, which you can use to install dependencies globally on your system. For example,npmg nodemon
.npmS
is an alias fornpm install --save
, which you use to install dependencies and add them to thedependencies
section of yourpackage.json
. Note that, as of npm 5.0.0, this is the default when runningnpm i <package>
.npmD
is an alias fornpm install --save-dev
, which you use to install dependencies and add them to thedevDependencies
section of yourpackage.json
.npmO
is an alias fornpm outdated
, which will check the registry to see if any (or, specific) installed packages are currently outdated.npmL
is an alias fornpm list
, which will list installed packages.npmL0
is an alias fornpm list --depth=0
, which lists top-level packages. This is especially useful for seeing which modules are installed globally without flooding your terminal with a huge dependency tree:npmL0 -g
.npmst
is an alias fornpm run start
, an npm script often used to start an application.npmt
is an alias fornpm run test
, which, as you might guess, is used to run your tests.npmR
is an alias fornpm run
. On its own, this command will list all of a project’s available npm scripts, along with a description of what they do. Used in conjunction with a script name, it will run that script, For example,npmR build
.npmI
is an alias fornpm init
. This will ask you a bunch of questions, then create apackage.json
based on your answers. Use the-y
flag to automate the process. For example,npmI -y
.
10 Time-saving Rails/Rake Aliases
This plugin adds completion for the Ruby on Rails framework and the Rake program, as well as some aliases for logs and environment variables.
rc
is an alias forrails console
, which allows you to interact with your Rails app from the CLI.rdc
is an alias forrake db:create
, which (unlessRAILS_ENV
is set) creates the development and test databases for your app.rdd
is an alias forrake db:drop
, which drops your app’s development and test databases.rdm
is an alias forrake db:migrate
, which will run any pending database migrations.rds
is an alias forrake db:seed
, which runs thedb/seeds.rb
file to populate your development database with data.rgen
is an alias forrails generate
, which will generate boilerplate code. For example:rgen scaffold item name:string description:text
.rgm
is an alias forrails generate migration
, which will generate a database migration. For example:rgm add_description_to_products description:string
.rr
is an alias forrake routes
, which list all of an app’s defined routes.rrg
is an alias forrake routes | grep
, which will allow you to list and filter the defined routes. For example,rrg user
.rs
is an alias forrails server
, which launches the Rails default web server.
Additional Resources
The main job of the plugins listed above is to provide aliases to often-used commands. Please be aware that there are lots more plugins out there that augment your shell with additional functionality.
Here are four of my favorites:
- sudo allows you to easily prefix your current or previous commands with
sudo
by pressing ESC twice. - autosuggestions suggests commands as you type based on history and completions. If the suggestion is the one you’re looking for, press the → key to accept it. A real time saver!
- command-not-found: if a command isn’t recognized in the
$PATH
, this will use Ubuntu’s command-not-found package to find it or suggest spelling mistakes. - z is a handy plugin that builds a list of your most frequent and recent folders (it calls these “frecent”) and allows you to jump to them with one command.
And don’t forget, if you spend a lot of time in the terminal, it’s worth investing some effort in making it visually appealing. Luckily, Oh My Zsh ships with a whole bunch of themes for you to choose from. My pick of the bunch is Agnoster.
You can find out more about themes in my article 10 Zsh Tips & Tricks: Configuration, Customization & Usage.
Conclusion
So there we have it: 75 Zsh commands, plugins, aliases and tools. I hope you’ve learned a trick or two along the way, and I encourage you to get out of your GUIs and into the terminal. It’s easier than it looks and a great way to boost your productivity.
If I’ve missed your favorite plugin, or time-saving alias/command, let me know on Twitter.
Want to get even more out of your toolkit? Check out Visual Studio Code: End-to-End Editing and Debugging Tools for Web Developers from Wiley.
FAQs About Zsh Commands, Plugins, Aliases and Tools
What are the benefits of using Zsh over other shells like Bash?
Zsh, also known as the Z shell, is a powerful shell that includes features from other shells such as Bash, tcsh, and ksh. It offers several advantages over these shells. Firstly, Zsh has a robust auto-completion feature that can suggest commands, file names, options, and even hostnames. This feature can significantly speed up your workflow. Secondly, Zsh supports shared command history, which allows you to see commands that are being typed in another terminal session. Thirdly, Zsh allows you to use both Emacs and vi editing modes, making it flexible for different users. Lastly, Zsh has a powerful scripting language with features like associative arrays and floating-point arithmetic, which are not available in Bash.
How can I customize my Zsh prompt?
Zsh allows you to customize your prompt using the PROMPT variable. You can set this variable in your .zshrc file. For example, if you want to display the current directory in your prompt, you can use the %~ parameter. So, your PROMPT variable would look like this: PROMPT=’%~%# ‘. You can also add colors to your prompt using the %F{color} parameter. For example, to make your prompt green, you can use: PROMPT=’%F{green}%~%# %f’. The %f resets the color to the default color.
How can I use aliases in Zsh?
Aliases in Zsh can help you save time by allowing you to create shortcuts for long or frequently used commands. You can create an alias using the alias command followed by the name of the alias and the command you want to shortcut. For example, to create an alias for the ls -l command, you can use: alias ll=’ls -l’. You can add this line to your .zshrc file to make the alias permanent.
What are Zsh plugins and how can I use them?
Zsh plugins are scripts that add additional functionality to your Zsh shell. They can help you automate tasks, add new features, or customize your shell. To use a Zsh plugin, you first need to install it. This usually involves cloning the plugin’s repository into your .oh-my-zsh/plugins directory and then adding the plugin to the plugins array in your .zshrc file. Once the plugin is installed, you can start using its features.
How can I switch from Bash to Zsh?
Switching from Bash to Zsh is a straightforward process. First, you need to install Zsh using your package manager. For example, on Ubuntu, you can use: sudo apt install zsh. Once Zsh is installed, you can make it your default shell using the chsh command: chsh -s $(which zsh). The next time you open a terminal, it will use Zsh.
How can I use Zsh themes?
Zsh themes allow you to customize the appearance of your shell. You can change the color scheme, prompt layout, and even add elements like the current time or git status. To use a Zsh theme, you first need to install it. This usually involves cloning the theme’s repository into your .oh-my-zsh/themes directory and then setting the ZSH_THEME variable in your .zshrc file to the name of the theme. Once the theme is installed, you can activate it by sourcing your .zshrc file: source ~/.zshrc.
What is Oh My Zsh and how can I use it?
Oh My Zsh is a community-driven framework for managing your Zsh configuration. It comes with a lot of helpful functions, plugins, and themes. To use Oh My Zsh, you first need to install it. This usually involves downloading the installation script and running it in your terminal. Once Oh My Zsh is installed, you can start customizing your shell by editing your .zshrc file.
How can I use Zsh functions?
Zsh functions allow you to group commands together and execute them as a single command. You can define a function using the function keyword followed by the name of the function and a block of commands. For example, to create a function that prints “Hello, world!”, you can use: function hello { echo “Hello, world!”; }. You can call this function by typing its name: hello.
How can I use Zsh arrays?
Zsh supports both indexed and associative arrays. You can create an indexed array using the set command followed by the array name and the elements. For example, to create an array of colors, you can use: set -A colors red green blue. You can access an element of the array using its index: echo $colors[1]. To create an associative array, you can use the typeset command: typeset -A colors; colors[red]=#FF0000; colors[green]=#00FF00; colors[blue]=#0000FF.
How can I use Zsh loops?
Zsh supports several types of loops, including for, while, and until loops. A for loop allows you to repeat a block of commands for each element in a list. For example, to print the numbers from 1 to 5, you can use: for i in {1..5}; do echo $i; done. A while loop allows you to repeat a block of commands while a condition is true. For example, to print the numbers from 1 to 5, you can use: i=1; while (( i <= 5 )); do echo $i; ((i++)); done. An until loop is similar to a while loop, but it repeats the commands until the condition is true.
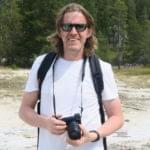
Network admin, freelance web developer and editor at SitePoint.