By now, we’ve all seen Twitter Bootstrap – it’s a great CSS and Javascript library open sourced by Twitter that makes it easy to produce a very polished looking site, with fantastic support for layout, navigation, typography, and much more. Twitter Bootstrap is based on Less.js, the popular dynamic CSS scripting language written by Alexis Sellier or @cloudhead. Less.js, like Node.js, is implemented completely with Javascript. While Less is based on Javascript and not Ruby, some great work has been done just in the last couple of months to make it easy to set up Twitter Bootstrap in your Rails 3.1 app using a variety of different approaches.
![]() |
Twitter Bootstrap is a great way to quickly build a very polished web site. |
Twitter Bootstrap basics
On the surface, Twitter Bootstrap is simply a single CSS file, bootstrap.css, that provides all of the style and layout support, along with a few Javascript files that implement various dynamic features. This means the fastest and simplest way to get started using it is just to copy bootstrap.css into your Rails 3.1 app/assets/stylesheets folder like this:$ git clone https://github.com/twitter/bootstrap.git
$ cp bootstrap/bootstrap.css path/to/app/assets/stylesheets/.
…and you’re off to the races! Use their online demo page as a guide to get started; you can even use “Inspect Element” or “View Source” right on that page to get a sense of what styles they’re using for each feature.
However, as I mentioned above, Twitter Bootstrap is based on the Less.js framework, and the bootstrap.css file is a actually a compiled version of the Less code contained in a series of “.less” code files:
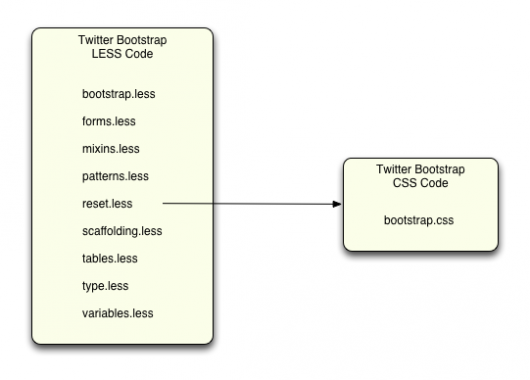
Less-rails-bootstrap
Fortunately, Ken Collins did some great work during the past few months to solve this problem; he wrote a new gem called less-rails-bootstrap that adds support for Less into the Rails 3.1 asset pipeline. You can read his blog post or check out his Github readme page for the details. Another gem called twitter-bootstrap-rails by Seyhun Akyürek uses the same approach, although at first glance twitter-bootstrap-rails doesn’t appear to have any tests in it, while Ken’s less-rails-bootstrap does have an effective MiniTest::Spec test suite. I’ll repeat the setup steps from Ken’s blog post here; first just add less-rails-bootstrap (or twitter-bootstrap-rails) to your Gemfile in the :assets group:group :assets do
gem 'less-rails-bootstrap'
end
And install it using “bundle install.” Then all you need to do is require the Twitter CSS code from your app/assets/stylesheets/application.css file like this:
/*
*= require twitter/bootstrap
*/
As Ken explains, now you’re free to override and manipulate the Twitter Bootstrap Less code directly; for example if you add this code to a new file with a .css.less extension:
@import "twitter/bootstrap";
#foo {
.border-radius(4px);
}
… you now have a cross-browser compatible CSS style that will apply a rounded border with a radius of 4 pixels. But how does this actually work? Let’s take a closer look:
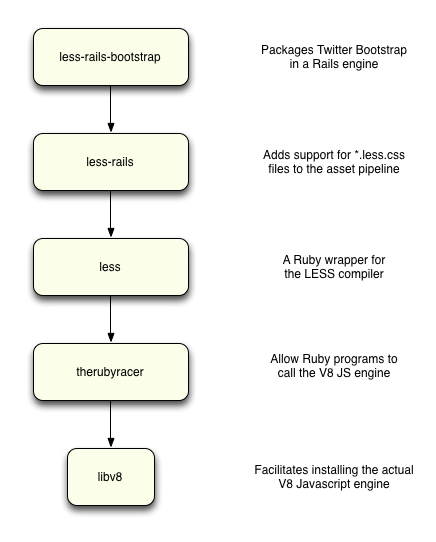
- less-rails-bootstrap: Ken’s gem actually includes the Twitter Bootstrap Less code files (in the vendor/assets folder) and provides a Rails engine to make them accessible to your app. More on this in a minute…
- less-rails: Ken wrote also wrote this gem, based on an earlier version by Karst Hammer, that helps integrate Less with the Rails 3.1 asset pipeline, providing extensions that Rails developers would expect. See Ken’s clarification on this in the comments.
- less.rb: This gem, written by Charles Lowell, is a thin wrapper around the Less language compiler, allowing your Rails app to call it.
- therubyracer: Also written by Charles Lowell, this gem provides Ruby programs, in this case your Rails 3.1 app, the ability to call the V8 Javascript engine.
- libv8: This gem makes it easy to install or compile from source the actual V8 Javascript library, which itself is implemented mostly in C.
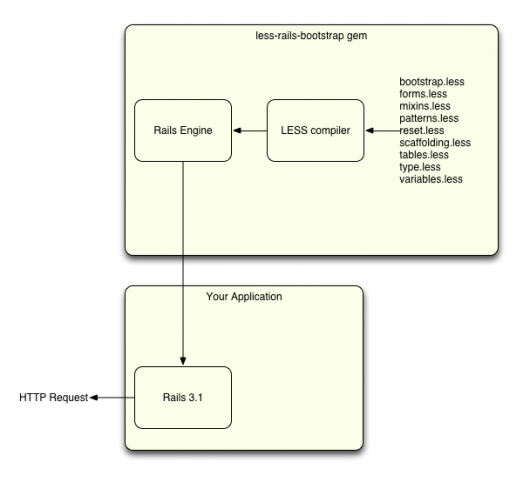
$ cd `bundle show less-rails-bootstrap`
$ find vendor
vendor
vendor/assets
vendor/assets/javascripts
vendor/assets/javascripts/twitter
vendor/assets/javascripts/twitter/bootstrap
vendor/assets/javascripts/twitter/bootstrap/alerts.js
vendor/assets/javascripts/twitter/bootstrap/buttons.js
vendor/assets/javascripts/twitter/bootstrap/dropdown.js
vendor/assets/javascripts/twitter/bootstrap/modal.js
vendor/assets/javascripts/twitter/bootstrap/popover.js
vendor/assets/javascripts/twitter/bootstrap/scrollspy.js
vendor/assets/javascripts/twitter/bootstrap/tabs.js
vendor/assets/javascripts/twitter/bootstrap/twipsy.js
vendor/assets/javascripts/twitter/bootstrap.js
vendor/assets/stylesheets
vendor/assets/stylesheets/twitter
vendor/assets/stylesheets/twitter/bootstrap.css.less
vendor/frameworks
vendor/frameworks/twitter
vendor/frameworks/twitter/bootstrap
vendor/frameworks/twitter/bootstrap/bootstrap.less
vendor/frameworks/twitter/bootstrap/forms.less
vendor/frameworks/twitter/bootstrap/mixins.less
vendor/frameworks/twitter/bootstrap/patterns.less
vendor/frameworks/twitter/bootstrap/reset.less
vendor/frameworks/twitter/bootstrap/scaffolding.less
vendor/frameworks/twitter/bootstrap/tables.less
vendor/frameworks/twitter/bootstrap/type.less
vendor/frameworks/twitter/bootstrap/variables.less
vendor/frameworks/twitter/bootstrap.less
Sass-twitter-bootstrap
While Ken’s done a great job with less-rails-bootstrap, you may prefer to use Sass instead of Less, because it’s already supported by Rails 3.1 out of the box, or because your application already contains a large amount of Sass code, or possibly just because you’re more familiar with it. At first glance, this seems to be a serious problem for Twitter Bootstrap: it’s not appropriate for a large portion of the Rails community that prefers Sass, since it was implemented with the Javascript-centric Less.js technology. Don’t worry – some other great developers (John Long, Jeremy Hinegardner and others) ran into this dilemma already and came up with a solution: they translated Twitter Bootstrap’s Less code into Sass, and released that as a separate library on Github, called Sass-twitter-bootstrap. Sass-twitter-bootstrap is not a gem, but instead is just a github repo containing Twitter’s translated code. To use it in your Rails 3.1 app, just clone the repo and copy the bootstrap css file into your app, like this:$ git clone https://github.com/jlong/sass-twitter-bootstrap.git
$ cp sass-twitter-bootstrap/bootstrap.css path/to/app/assets/stylesheets/.
Of course, this isn’t really any different than copying the bootstrap.css file directly from the Twitter Bootstrap repo; instead what you should do is copy the Sass source files right into your application like this:
$ rm path/to/app/assets/stylesheets/bootstrap.css
$ cp -r sass-twitter-bootstrap/lib path/to/app/assets/stylesheets/twitter
And now since the Rails 3.1 asset pipeline supports Sass out of the box, we’re good to go… almost! If you run your app now, you’ll see an error:
ActionView::Template::Error (Undefined variable: "$baseline".
(in /path/to/app/assets/stylesheets/twitter/forms.scss))
Thanks to Brent Collier, there’s a simple solution for this problem: the code in application.css by default uses “require_tree” to include all of the code under app/assets/stylesheets:
/*
* This is a manifest file that'll automatically include all the stylesheets available in this directory
* and any sub-directories. You're free to add application-wide styles to this file and they'll appear at
* the top of the compiled file, but it's generally better to create a new file per style scope.
*= require_self
*= require_tree .
*/
As Brent explains, the problem with this is that Twitter Bootstrap’s Less code (and the translated Sass version) was designed to be included once using the bootstrap.scss file, which in turns includes all of the other files. Brent’s solution works perfectly: just remove “require_tree” and require bootstrap.scss directly:
/*
* This is a manifest file that'll automatically include all the stylesheets available in this directory
* and any sub-directories. You're free to add application-wide styles to this file and they'll appear at
* the top of the compiled file, but it's generally better to create a new file per style scope.
*= require_self
*= require twitter/bootstrap
*/
Conceptually, here’s what this setup looks like:
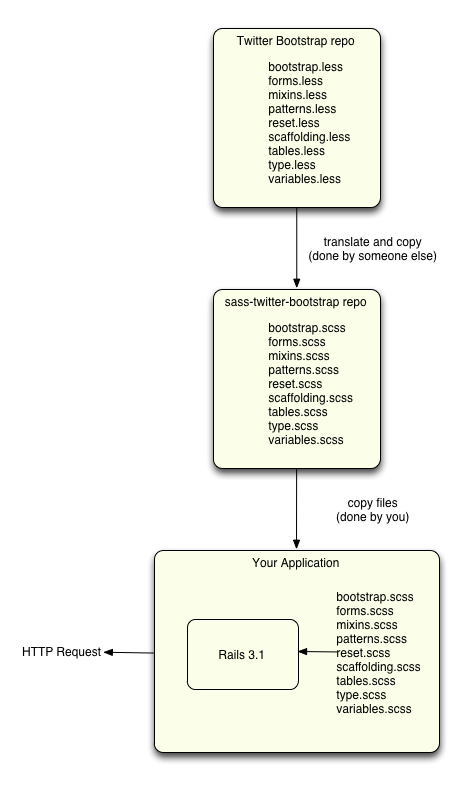
Bootstrap-sass and bootstrap-rails
Like everything in the Rails world, there are always other good solutions out there you can take a look at, in this case the bootstrap-sass gem by Thomas McDonald and the bootstrap-rails gem by AnjlLab. Both of these gems combine the Sass translation approach with a Rails engine to avoid the manual copy maintenance headache. Using bootstrap-sass (or bootstrap-rails) is a simple as adding it to your Gemfile:group :assets do
gem 'bootstrap-sass'
end
Run “bundle install” and then add a require statement to app/assets/stylesheets/application.css:
/*
*= require bootstrap
*/
Similar to less-rails-bootstrap, this works by having Rails 3.1 load the Sass code from a Rails engine inside the gem:
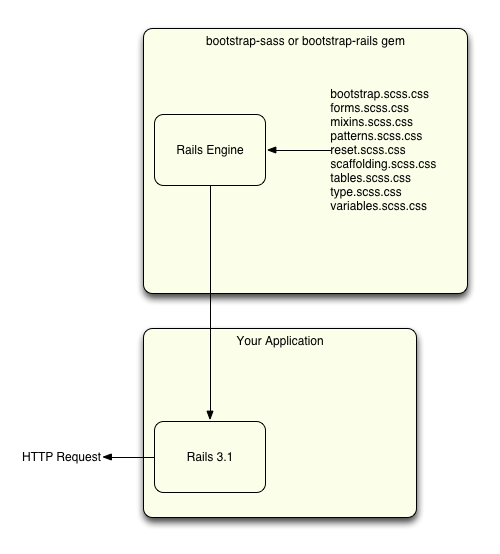
Other options
Amazingly, there are even more options out there for integrating Rails 3.1 and Twitter Bootstrap that I don’t have time to cover today:- compass-twitter-bootstrap is similar to bootstrap-sass and bootstrap-rails, using a Rails engine to provide a translated version of the Twitter Bootstrap code, but is geared instead to the Compass CSS framework.
- twitter_bootstrap_form_for implements a custom Form Builder object, the object yielded by form_for in your view, designed specifically for Twitter Bootstrap.
- css-bootstrap-rails is similar to bootstrap-sass and bootstrap-rails, but uses a Rails engine to include only bootstrap.css without any Less or Sass files.
Six of one, half dozen of the other
All of these approaches will work equally well:- Using the original Less code via a Rails engine (less-rails-bootstrap or twitter-bootstrap-rails)
- Copying in translated Sass code directly into your application (sass-twitter-bootstrap), or
- Using a translated Sass version via a Rails engine (bootstrap-sass or bootstrap-rails).
Frequently Asked Questions (FAQs) about Bootstrap, Less, Sass, and Rails 3.1
How do I install Bootstrap in Rails 3.1?
To install Bootstrap in Rails 3.1, you need to add the Bootstrap gem to your Gemfile. Open your Gemfile and add the following line: gem 'bootstrap-sass', '~> 3.3.6'
. Then, run bundle install
to install the gem. After that, you need to import Bootstrap styles in your application.css
file. Rename this file to application.scss
and then import Bootstrap styles by adding @import "bootstrap";
at the beginning of the file.
What is the difference between Less and Sass?
Less and Sass are both CSS pre-processors, which means they extend the default capabilities of CSS. The main difference between them is the way they handle variables, mixins, and functions. Less uses ‘@’ for variables and ‘.’ for mixins, while Sass uses ‘ for variables and ‘@mixin’ for mixins. Sass also supports control directives for libraries, while Less does not.
How do I use Less with Rails 3.1?
To use Less with Rails 3.1, you need to add the Less Rails gem to your Gemfile. Open your Gemfile and add the following line: gem 'less-rails'
. Then, run bundle install
to install the gem. After that, you can start using Less in your Rails application by creating .less
files in your stylesheets directory.
How do I use Sass with Rails 3.1?
To use Sass with Rails 3.1, you need to add the Sass Rails gem to your Gemfile. Open your Gemfile and add the following line: gem 'sass-rails', '~> 3.1'
. Then, run bundle install
to install the gem. After that, you can start using Sass in your Rails application by creating .scss
files in your stylesheets directory.
How do I customize Bootstrap in Rails 3.1?
To customize Bootstrap in Rails 3.1, you need to override Bootstrap’s default variables. You can do this by creating a new .scss
file in your stylesheets directory and importing it after Bootstrap in your application.scss
file. In this new file, you can redefine any of Bootstrap’s default variables to customize its styles.
How do I update Bootstrap in Rails 3.1?
To update Bootstrap in Rails 3.1, you need to update the Bootstrap gem in your Gemfile. Open your Gemfile and change the version number of the Bootstrap gem to the latest version. Then, run bundle update bootstrap-sass
to update the gem. After that, you need to restart your Rails server for the changes to take effect.
How do I use Bootstrap components in Rails 3.1?
To use Bootstrap components in Rails 3.1, you need to import Bootstrap’s JavaScript file in your application.js
file. Add the following line at the end of the file: //= require bootstrap
. After that, you can start using Bootstrap components in your Rails views by adding the appropriate HTML and classes.
How do I troubleshoot issues with Bootstrap in Rails 3.1?
If you’re having issues with Bootstrap in Rails 3.1, there are a few things you can check. First, make sure you’ve correctly installed the Bootstrap gem and imported its styles and JavaScript. Second, check your console for any error messages. These messages can often provide clues about what’s going wrong. Finally, make sure your HTML is correctly structured and you’re using the right classes for Bootstrap components.
How do I use Bootstrap with the asset pipeline in Rails 3.1?
To use Bootstrap with the asset pipeline in Rails 3.1, you need to import Bootstrap’s styles and JavaScript in your asset pipeline. You can do this by adding @import "bootstrap";
to your application.scss
file and //= require bootstrap
to your application.js
file. After that, Rails will automatically include Bootstrap’s styles and JavaScript when compiling your assets.
How do I use Bootstrap with Turbolinks in Rails 3.1?
Turbolinks can cause issues with Bootstrap’s JavaScript in Rails 3.1. To solve this, you need to listen for the turbolinks:load
event and initialize your Bootstrap components in its callback. You can do this by adding the following JavaScript code to your application.js
file: $(document).on('turbolinks:load', function() { // Initialize your Bootstrap components here });
.
Pat Shaughnessy writes a blog about Ruby development and recently self-published an eBook called Ruby Under a Microscope. When he's not at the keyboard, Pat enjoys spending time with his wife and two kids. Pat is also a fluent Spanish speaker and travels frequently to Spain to visit his wife's family.