Recently, I had to work on a website heavily using tilted angles as part of its design guidelines. By “tilted angles”, I mean these sections whose top or bottom edge is not completely horizontal and rather a bit inclined.
There are quite a few ways to implement this. You could have base 64 encoded images applied as a background, but it makes it hard to customise (color, angle, etc.).
Another way would be to skew and/or rotate an absolutely positioned pseudo-element, but if there is one thing I don’t want to deal with, it’s the skew transform. God, no.
When using Sass, you could use the Angled Edges library that encodes dynamically generated SVG. It works super well, however it requires a fixed width and height expressed in pixels, which kind of bothered me.
I also really wanted to see if and how I could be able to implement this. I ended up with a solution that I am really proud of, even if it might be a bit over-engineered for simple scenarios.
What’s the Idea?
My idea was to apply a half transparent, half solid gradient to an absolutely-positioned pseudo-element. The angle of the gradient defines the tilt angle.
background-image: linear-gradient($angle, $color 50%, transparent 50%);
This is packaged in a mixin that applies the background color to the container, and generates a pseudo-element with the correct gradient based on the given angle. Simple enough. You’d use it like this:
.container {
@include tilted($angle: 3deg, $color: rgb(255, 255, 255));
}
The main problem I faced with this was to figure which height the pseudo-element should have. At first, I made it an argument of the mixin, but I ended up going trial-and-error at every new angle to figure out what would be the best height for the pseudo. Not ideal.
And just when I was about to table flip the whole thing, I decided to leave the laptop away, took a pen and paper and started doodling to figure out the formula behind it. It took me a while (and a few Google searches) to remember trigonometry I learnt in high-school, but eventually I made it.
To avoid having to guess or come up with good approximations, the height of the pseudo-element is computed from the given angle. This is, of course, all done with a bit of Sass and a lot of geometry. Let’s go.
Computing the Pseudo-element’s Height
Trust me when I’m telling you it won’t be too hard. The first thing we know is that we have a full-width pseudo-element. The gradient line will literally be the pseudo’s diagonal, so we end up with a rectangle triangle.
Let’s name it ABC, where C
is the right angle, B
is the known angle ($angle
argument) and A
is therefore C - B
. As shown on this diagram, we try to figure out what b
is.
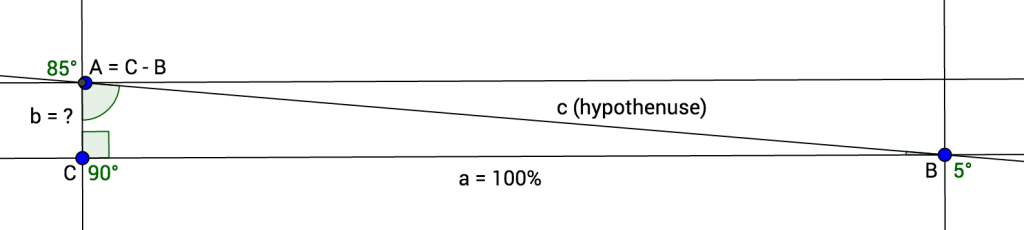
To do that, we need to find the value of c
(the gradient line, aka the hypothenuse), which is the length of a
(the bottom side, 100%) divided by sine of the A
angle (with B = 5°
, A
would be 85° for instance).
c = a / sin(C - B)
From there, we have to use Pythagoras theorem:
The square of the hypotenuse (the side opposite the right angle) is equal to the sum of the squares of the other two sides.
Therefore, the square of one of the other side equals the square of the hypothenuse minus the square of the third side. So the square of b
equals the square of c
minus the square of a
.
b² = c² - a²
Finally, the length of b
equals the squared root of the square of c
minus the square of a
.
b = √(c² - a²)
That’s it. We can now build a small Sass function computing the height of the pseudo-element based on a given angle.
@function get-tilted-height($angle) {
$a: (100% / 1%);
$A: (90deg - $angle);
$c: ($a / sin($A));
$b: sqrt(pow($c, 2) - pow($a, 2));
@return (abs($b) * 1%);
}
Note: the pow()
, sqrt()
and sin()
functions can either come from Sassy-Math, Compass or custom sources.
Building the Tilted Mixin
We’ve done the hardest part, trust me! The last thing to do is build the actual tilted()
mixin. It accepts an angle and a color as argument and generates a pseudo-element.
@mixin tilted($angle, $color) {
$height: get-tilted-height($angle);
position: relative;
background-color: $color;
&::before {
content: '';
padding-top: $height;
position: absolute;
left: 0;
right: 0;
bottom: 100%;
background-image: linear-gradient($angle, $color 50%, transparent 50%);
}
}
A few things to note here: the mixin applies position: relative
to the container to define a position context for the pseudo-element. When using this mixin on absolute or fixed elements, it might be worth considering removing this declaration from the mixin.
The mixin applies the background color to the container itself as well as pseudo’s gradient, as they have to be synced anyway.
Finally, the pseudo-element’s height has to be conveyed through padding-top
(or padding-bottom
for that matter) instead of height
. Since the height is expressed in percentages based on the parent’s width, we cannot rely on height
(as it computes from the parent’s height).
Final Thoughts and Going Further
For this article, I’ve chosen to go with a simple version of the mixin, which might lack flexibility and could, in theory, present the following problems:
- It is not possible to use it on an element already making use of its
::before
pseudo-element. This could be solved by adding an optional parameter to specify the pseudo-element, defaulting tobefore
. - It is not possible to display a tilted edge at the bottom of the container as
bottom: 0
is currently hard-coded in the mixin core. This could be solved by making it possible to pass an extra position to the mixin.
Also, my existing version uses Sass-based math functions as it was in a Jekyll project, not allowing me to extend the Sass layer. If using node-sass, you could easily pass these functions from JavaScript to Sass through Eyeglass or Sassport, which would be definitely much better.
I hope you liked it! If you can think of anything to improve it, please feel free to share in the comments. :)
See the Pen Tilted Angles in CSS by SitePoint (@SitePoint) on CodePen.
Frequently Asked Questions (FAQs) about Tilted Angles in Sass
How can I use the Sass sqrt function to calculate tilted angles?
The Sass sqrt function is a powerful tool that can be used to calculate tilted angles. To use it, you need to pass the value you want to find the square root of as an argument to the function. For example, if you want to find the square root of 2, you would write sqrt(2)
. This will return the square root of 2, which is approximately 1.414. You can then use this value in your calculations to determine the angle of tilt. Remember, the sqrt function only works with numbers, so make sure to convert any string values to numbers before using them in the function.
What is the purpose of using tilted angles in Sass?
Tilted angles in Sass are used to create dynamic and visually appealing designs. They can be used to rotate elements, create skewed effects, or even to create complex geometric shapes. By using tilted angles, you can add a unique and interesting visual element to your website or application. They can also be used to create a sense of movement or dynamism, making your design more engaging and interactive.
Can I use other mathematical functions in Sass besides sqrt?
Yes, Sass supports a variety of mathematical functions, including addition, subtraction, multiplication, division, and modulus. These functions can be used to perform calculations and manipulate values in your stylesheets. For example, you can use the addition function to increase the size of an element, or the division function to divide a value into equal parts. This makes Sass a powerful tool for creating complex and dynamic designs.
How can I convert string values to numbers in Sass?
In Sass, you can convert string values to numbers using the unitless()
function. This function removes the unit from a number, effectively converting it into a unitless number. For example, if you have a string value of “10px”, you can convert it to a number by writing unitless("10px")
. This will return the number 10, which you can then use in your calculations.
What are some common use cases for the Sass sqrt function?
The Sass sqrt function is commonly used in calculations involving geometry, such as calculating the length of the diagonal of a square or the radius of a circle. It can also be used to calculate the distance between two points in a coordinate system. In addition, the sqrt function can be used in animations and transitions to create smooth and natural movements.
How can I use the Sass sqrt function to create a diagonal line?
To create a diagonal line using the Sass sqrt function, you first need to calculate the length of the diagonal. This can be done using the Pythagorean theorem, which states that the square of the length of the diagonal is equal to the sum of the squares of the lengths of the sides. You can then use the sqrt function to find the square root of this sum, which will give you the length of the diagonal. You can then use this value to set the width and height of the line, and rotate it to the desired angle.
Can I use the Sass sqrt function with variables?
Yes, you can use the Sass sqrt function with variables. This allows you to create dynamic and flexible stylesheets that can easily be updated and modified. For example, you could define a variable for the length of a side of a square, and then use the sqrt function to calculate the length of the diagonal. This would allow you to easily change the size of the square and have the length of the diagonal update automatically.
How can I use the Sass sqrt function to create a circle?
To create a circle using the Sass sqrt function, you first need to calculate the radius of the circle. This can be done by dividing the diameter of the circle by 2. You can then use the sqrt function to find the square root of the radius, which will give you the length of the radius. You can then use this value to set the width and height of the circle, and use the border-radius property to make the circle round.
What is the syntax for the Sass sqrt function?
The syntax for the Sass sqrt function is sqrt($value)
, where $value
is the value you want to find the square root of. The function will return the square root of the value. Remember, the value must be a number, so make sure to convert any string values to numbers before using them in the function.
Can I use the Sass sqrt function in combination with other functions?
Yes, you can use the Sass sqrt function in combination with other functions. This allows you to create complex calculations and manipulations. For example, you could use the sqrt function in combination with the pow function to calculate the length of the diagonal of a rectangle. This would involve squaring the lengths of the sides, adding them together, and then finding the square root of the sum.
Non-binary trans accessibility & diversity advocate, frontend developer, author. Real life cat. She/they.