//selector for injected iframe
var iframeSrc = $('#iframe').attr('src');
Frequently Asked Questions (FAQs) about Injecting iFrame with jQuery
What is the purpose of injecting an iFrame with jQuery?
Injecting an iFrame with jQuery is a common practice in web development. It allows developers to dynamically load content from another webpage or website into a current webpage without requiring a page refresh. This can be particularly useful for loading multimedia content, such as videos, or other interactive elements that are hosted on different servers or domains. It can also be used to create more complex web applications that require the loading of different components or modules.
How can I change the source of an iFrame using jQuery?
Changing the source of an iFrame using jQuery is quite straightforward. You can use the attr() method to change the ‘src’ attribute of the iFrame. Here’s a simple example:$('iframe').attr('src', 'new_source_url');
In this example, ‘new_source_url’ should be replaced with the URL of the new source you want to load in the iFrame.
How can I get the current source of an iFrame using jQuery?
You can get the current source of an iFrame using the attr() method in jQuery. Here’s how you can do it:var currentSrc = $('iframe').attr('src');
In this example, the variable ‘currentSrc’ will hold the current source URL of the iFrame.
Can I inject an iFrame into a specific part of my webpage using jQuery?
Yes, you can inject an iFrame into a specific part of your webpage using jQuery. You can use the append() or prepend() methods to add the iFrame to a specific element on your page. Here’s an example:$('#myElement').append('<iframe src="my_source_url"></iframe>');
In this example, an iFrame with the source ‘my_source_url’ is added to the end of the element with the id ‘myElement’.
How can I handle errors when loading an iFrame with jQuery?
You can handle errors when loading an iFrame with jQuery using the error() method. This method triggers a function when an error occurs while loading the iFrame. Here’s an example:$('iframe').error(function() {
alert('An error occurred while loading the iFrame.');
});
In this example, an alert is displayed when an error occurs while loading the iFrame.
Can I load an iFrame with jQuery when a user clicks a button?
Yes, you can load an iFrame with jQuery when a user clicks a button. You can use the click() method to trigger the loading of the iFrame when the button is clicked. Here’s an example:$('#myButton').click(function() {
$('iframe').attr('src', 'my_source_url');
});
In this example, the source of the iFrame is changed to ‘my_source_url’ when the button with the id ‘myButton’ is clicked.
How can I check if an iFrame has finished loading with jQuery?
You can check if an iFrame has finished loading with jQuery using the load() method. This method triggers a function when the iFrame has finished loading. Here’s an example:$('iframe').load(function() {
alert('The iFrame has finished loading.');
});
In this example, an alert is displayed when the iFrame has finished loading.
Can I inject multiple iFrames with jQuery?
Yes, you can inject multiple iFrames with jQuery. You can use the each() method to iterate over a set of elements and inject an iFrame into each one. Here’s an example:$('.myElements').each(function() {
$(this).append('<iframe src="my_source_url"></iframe>');
});
In this example, an iFrame with the source ‘my_source_url’ is added to each element with the class ‘myElements’.
Can I inject an iFrame with jQuery on page load?
Yes, you can inject an iFrame with jQuery on page load. You can use the ready() method to trigger the injection of the iFrame when the page has finished loading. Here’s an example:$(document).ready(function() {
$('body').append('<iframe src="my_source_url"></iframe>');
});
In this example, an iFrame with the source ‘my_source_url’ is added to the body of the page when the page has finished loading.
Can I inject an iFrame with jQuery into a specific position in my webpage?
Yes, you can inject an iFrame with jQuery into a specific position in your webpage. You can use the insertBefore() or insertAfter() methods to add the iFrame before or after a specific element on your page. Here’s an example:$('<iframe src="my_source_url"></iframe>').insertBefore('#myElement');
In this example, an iFrame with the source ‘my_source_url’ is added before the element with the id ‘myElement’.
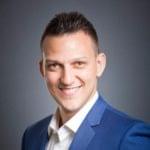
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.