Chances are that you want to set some kind of minimum requirements on your WooCommerce store before your customers checkout. What follows is a guide on how to set these requirements and restrictions, without needing to use any plugins at all:
- Setting A Minimum Weight Requirement Per Order
- Setting A Minimum Number Of Products Required Per Order
- Setting A Minimum Quantity Per Product
- Setting A Minimum Dollar Amount Per Order
Methods Used In This Article
There is always more than one way of setting minimum requirements in WooCommerce; the results might even be identical. But I consider the methods described below to be the right (or better) way of doing it. Any suggestions on how you accomplish these tasks or further improvements are always welcome and received well.
The following code has been tested in the latest versions available for WordPress (3.9.1) and WooCommerce (2.1.12). We’ll be using the dummy data provided for WooCommerce when you install the plugin. The code should go in your theme’s functions.php file and is heavily commented so it’s easier to follow and/or modify if needed.
We are going to be using the woocommerce_check_cart_items
action provided by WooCommerce to run our functions and execute our code. Visit the following link for a complete list of WooCommerce actions and filters, also known as hooks.
Setting A Minimum Weight Requirement Per Order
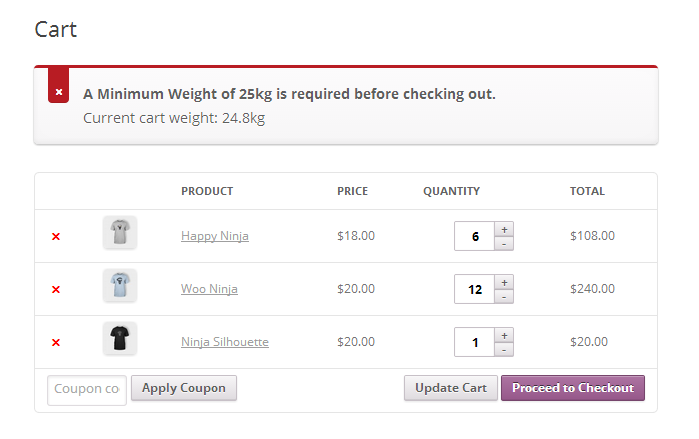
It is often useful to restrict your customer(s) from completing the checkout process without having met a minimum weight requirement. Minium weight requirements can help make your shipping costs more manageable, and the shipping process more streamlined. Don’t forget to change the minimum weight requirement to whatever works best for you, and keep in mind that weight is calculated in whatever weight unit you have set under WooCommerce -> Settings -> Products.
// Set a minimum weight requirement before checking out
add_action( 'woocommerce_check_cart_items', 'spyr_set_weight_requirements' );
function spyr_set_weight_requirements() {
// Only run in the Cart or Checkout pages
if( is_cart() || is_checkout() ) {
global $woocommerce;
// Set the minimum weight before checking out
$minimum_weight = 25;
// Get the Cart's content total weight
$cart_contents_weight = WC()->cart->cart_contents_weight;
// Compare values and add an error is Cart's total weight
// happens to be less than the minimum required before checking out.
// Will display a message along the lines of
// A Minimum Weight of 25kg is required before checking out. (Cont. below)
// Current cart weight: 12.5kg
if( $cart_contents_weight < $minimum_weight ) {
// Display our error message
wc_add_notice( sprintf('<strong>A Minimum Weight of %s%s is required before checking out.</strong>'
. '<br />Current cart weight: %s%s',
$minimum_weight,
get_option( 'woocommerce_weight_unit' ),
$cart_contents_weight,
get_option( 'woocommerce_weight_unit' ),
get_permalink( wc_get_page_id( 'shop' ) )
),
'error' );
}
}
}
Setting A Minimum Number Of Products Required Per Order
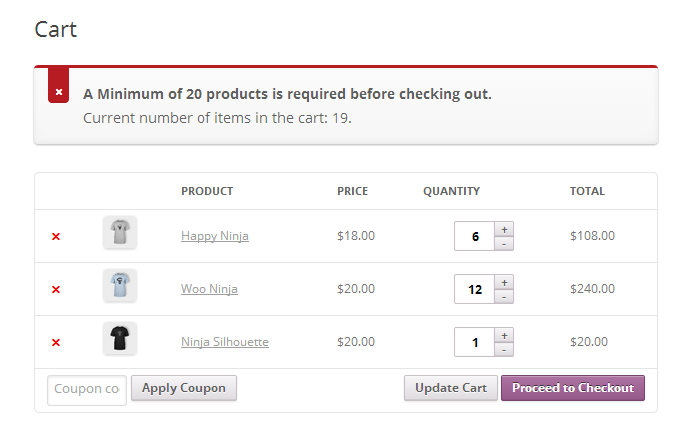
Another valid scenario is setting a minimum number of products that have to be ordered at a time before allowing the customer to fully pay for his order and shipped. Change ’20’ to whatever works best for your needs. As in the previous example, you want to make sure that you only run this code on the cart and checkout pages. This is we use is_cart() and is_checkout(), which return ‘true’ whenever we are on those two specific pages. Learn more about WooCommerce conditionals Tags.
// Set a minimum number of products requirement before checking out
add_action( 'woocommerce_check_cart_items', 'spyr_set_min_num_products' );
function spyr_set_min_num_products() {
// Only run in the Cart or Checkout pages
if( is_cart() || is_checkout() ) {
global $woocommerce;
// Set the minimum number of products before checking out
$minimum_num_products = 20;
// Get the Cart's total number of products
$cart_num_products = WC()->cart->cart_contents_count;
// Compare values and add an error is Cart's total number of products
// happens to be less than the minimum required before checking out.
// Will display a message along the lines of
// A Minimum of 20 products is required before checking out. (Cont. below)
// Current number of items in the cart: 6
if( $cart_num_products < $minimum_num_products ) {
// Display our error message
wc_add_notice( sprintf( '<strong>A Minimum of %s products is required before checking out.</strong>'
. '<br />Current number of items in the cart: %s.',
$minimum_num_products,
$cart_num_products ),
'error' );
}
}
}
Setting A Minimum Quantity Per Product
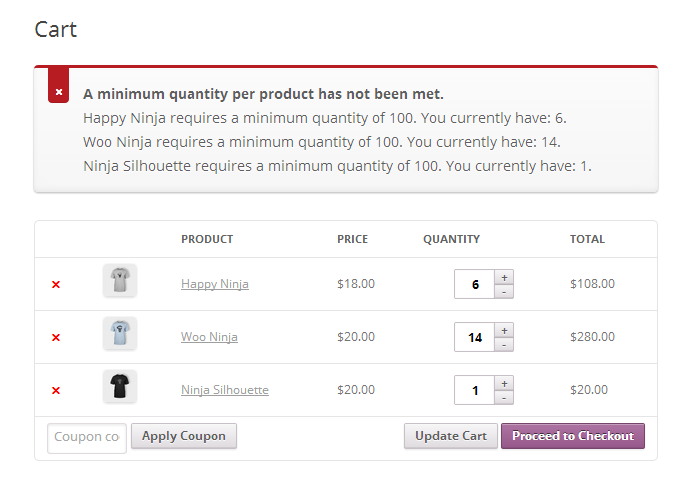
Setting a minimum quantity per product is a common requirement for WooCommerce stores, specially if you are selling wholesale. Setting a minimum quantity will restrict your customer(s) from purchasing a specific product in lesser quantities. For us to check for the minimum quantities, we need to loop through every single product in the cart and check it against our minimum quantity per product requirements set in place.
To set these restrictions, you need to create an array which holds your rules/restrictions inside another array. Be careful when editing this array, and make sure that all code is entered accurately in order to prevent errors and unexpected results. The format you need to use is as follows:
// Product Id and Min. Quantities per Product
// id = Product ID
// min = Minimum quantity
$product_min_qty = array(
array( 'id' => 47, 'min' => 100 ),
array( 'id' => 37, 'min' => 100 ),
array( 'id' => 34, 'min' => 100 ),
array( 'id' => 31, 'min' => 100 ),
);
Here is where the magic happens.
// Set minimum quantity per product before checking out
add_action( 'woocommerce_check_cart_items', 'spyr_set_min_qty_per_product' );
function spyr_set_min_qty_per_product() {
// Only run in the Cart or Checkout pages
if( is_cart() || is_checkout() ) {
global $woocommerce;
// Product Id and Min. Quantities per Product
$product_min_qty = array(
array( 'id' => 47, 'min' => 100 ),
array( 'id' => 37, 'min' => 100 ),
array( 'id' => 34, 'min' => 100 ),
array( 'id' => 31, 'min' => 100 ),
);
// Will increment
$i = 0;
// Will hold information about products that have not
// met the minimum order quantity
$bad_products = array();
// Loop through the products in the Cart
foreach( $woocommerce->cart->cart_contents as $product_in_cart ) {
// Loop through our minimum order quantities per product
foreach( $product_min_qty as $product_to_test ) {
// If we can match the product ID to the ID set on the minimum required array
if( $product_to_test['id'] == $product_in_cart['product_id'] ) {
// If the quantity required is less than than the quantity in the cart now
if( $product_in_cart['quantity'] < $product_to_test['min'] ) {
// Get the product ID
$bad_products[$i]['id'] = $product_in_cart['product_id'];
// Get the Product quantity already in the cart for this product
$bad_products[$i]['in_cart'] = $product_in_cart['quantity'];
// Get the minimum required for this product
$bad_products[$i]['min_req'] = $product_to_test['min'];
}
}
}
// Increment $i
$i++;
}
// Time to build our error message to inform the customer
// About the minimum quantity per order.
if( is_array( $bad_products) && count( $bad_products ) > 1 ) {
// Lets begin building our message
$message = '<strong>A minimum quantity per product has not been met.</strong><br />';
foreach( $bad_products as $bad_product ) {
// Append to the current message
$message .= get_the_title( $bad_product['id'] ) .' requires a minimum quantity of '
. $bad_product['min_req']
.'. You currently have: '. $bad_product['in_cart'] .'.<br />';
}
wc_add_notice( $message, 'error' );
}
}
}
Setting A Minimum Dollar Amount Per Order
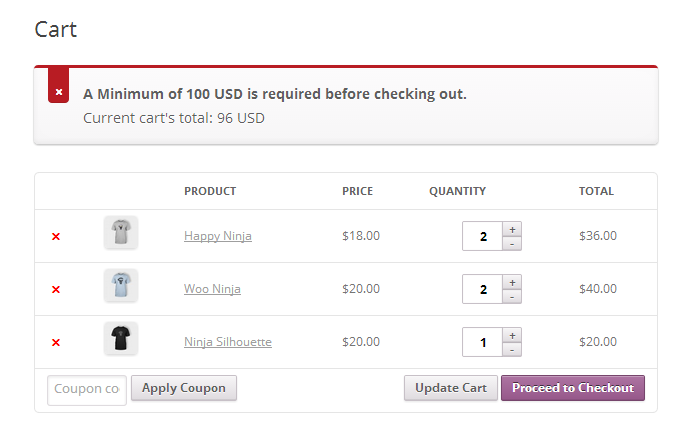
Last but not least, you may want to set a minimum dollar amount that must be spent before your customer is allowed to checkout. Please note that we are calculating the total value using the subtotal, before shipping and taxes are added to the final order total.
// Set a minimum dollar amount per order
add_action( 'woocommerce_check_cart_items', 'spyr_set_min_total' );
function spyr_set_min_total() {
// Only run in the Cart or Checkout pages
if( is_cart() || is_checkout() ) {
global $woocommerce;
// Set minimum cart total
$minimum_cart_total = 10;
// Total we are going to be using for the Math
// This is before taxes and shipping charges
$total = WC()->cart->subtotal;
// Compare values and add an error is Cart's total
// happens to be less than the minimum required before checking out.
// Will display a message along the lines of
// A Minimum of 10 USD is required before checking out. (Cont. below)
// Current cart total: 6 USD
if( $total <= $minimum_cart_total ) {
// Display our error message
wc_add_notice( sprintf( '<strong>A Minimum of %s %s is required before checking out.</strong>'
.'<br />Current cart\'s total: %s %s',
$minimum_cart_total,
get_option( 'woocommerce_currency'),
$total,
get_option( 'woocommerce_currency') ),
'error' );
}
}
}
Conclusion
Are you can see, WooCommerce allows you to use actions and filters to change the normal checkout process. All stores are different and being able to set these restrictions when needed is crucial. For us developers, who need to accomplish tasks like these, knowing how to do it is vital.
Thanks for reading, comments or suggestions on how to improve the code are always welcome. If you have ideas for a specific WooCommerce modification, don’t be shy and post a link to it in the comments for discussion.
Frequently Asked Questions on Minimum Checkout Requirements in WooCommerce
How Can I Set a Minimum Quantity for a Specific Product in WooCommerce?
To set a minimum quantity for a specific product in WooCommerce, you need to navigate to the product data section of the product you want to set a minimum quantity for. Under the ‘Inventory’ tab, you will find an option to set the ‘Minimum Quantity’. Enter the desired number and save your changes. This will ensure that customers must purchase at least this quantity of the product to proceed with checkout.
Can I Set a Maximum Quantity for Products in WooCommerce?
Yes, you can set a maximum quantity for products in WooCommerce. Similar to setting a minimum quantity, you can do this from the product data section under the ‘Inventory’ tab. There, you will find an option to set the ‘Maximum Quantity’. Enter the desired number and save your changes. This will limit the number of each product a customer can purchase in a single order.
Is it Possible to Limit the Total Quantity of Products in a Single Order?
Yes, it is possible to limit the total quantity of products in a single order in WooCommerce. This can be done by using a plugin like ‘MinMax Quantity for WooCommerce’. Once installed and activated, you can set a minimum and maximum limit for the total quantity of products in a single order from the plugin’s settings.
How Can I Update Product Stock Programmatically in WooCommerce?
Updating product stock programmatically in WooCommerce can be done by using WooCommerce’s built-in functions. You can use the wc_update_product_stock()
function to update the stock quantity of a product. This function takes two parameters: the product ID and the new stock quantity.
Can I Set a Minimum Order Amount in WooCommerce?
Yes, you can set a minimum order amount in WooCommerce. This can be done by using a plugin like ‘WooCommerce Min/Max Quantities’. Once installed and activated, you can set a minimum order amount from the plugin’s settings. This will require customers to reach this minimum order amount before they can proceed with checkout.
How Can I Set Quantity Increments for Products in WooCommerce?
Setting quantity increments for products in WooCommerce can be done by using a plugin like ‘WooCommerce Quantity Increment’. Once installed and activated, you can set quantity increments for each product from the product data section under the ‘Inventory’ tab.
Can I Set Different Minimum and Maximum Quantities for Different Products?
Yes, you can set different minimum and maximum quantities for different products in WooCommerce. This can be done from the product data section of each product. Under the ‘Inventory’ tab, you can set the ‘Minimum Quantity’ and ‘Maximum Quantity’ for each product individually.
Is it Possible to Set a Minimum and Maximum Quantity for Variations of a Product?
Yes, it is possible to set a minimum and maximum quantity for variations of a product in WooCommerce. This can be done from the variations section of the product data. For each variation, you can set a ‘Minimum Quantity’ and ‘Maximum Quantity’.
How Can I Display the Minimum and Maximum Quantity Requirements on the Product Page?
Displaying the minimum and maximum quantity requirements on the product page can be done by using a plugin like ‘WooCommerce Min/Max Quantities’. This plugin adds a notice on the product page displaying the minimum and maximum quantity requirements for the product.
Can I Set a Minimum and Maximum Quantity for Specific Categories of Products?
Yes, you can set a minimum and maximum quantity for specific categories of products in WooCommerce. This can be done by using a plugin like ‘WooCommerce Min/Max Quantities’. Once installed and activated, you can set minimum and maximum quantities for each product category from the plugin’s settings.
Yojance Rabelo has been using WordPress to build all kinds of Websites since July 2006. In his free time he likes to try out new technologies, mainly PHP and JavaScript based, and also playing with his EV3RSTORM, a Lego MINDSTORMS robot. He maintains his own blog among other sites about various topics.