Ever struggled with managing different configurations in your Node.js applications? Ever wanted a centralized and organized way to handle secrets like API keys and database credentials? Look no further! Environment variables are here to save the day. In this article, we’ll dive into the world of environment variables in Node.js, exploring their benefits, use cases, and best practices for managing them effectively.
We’ll guide you through setting up, accessing, and organizing environment variables, as well as using them across different environments and integrating them into npm scripts. By the end of this post, you’ll have a solid understanding of how environment variables can make your life as a Node.js developer easier.
Understanding Environment Variables in Node.js
Environment variables in Node.js serve as a source of configuration and secrets, allowing for easier debugging and maintenance of your applications. By externalizing app-specific settings, environment variables provide a single source of truth, making it simple to:
- run your application on different machines or environments without having to modify the code itself
- keep sensitive information, such as API keys or database credentials, separate from your code
- customize the behavior of your application based on the environment it’s running in
Using environment variables can greatly improve the flexibility and security of your Node.js applications.
What are environment variables?
Environment variables are named values that can be used to configure applications and processes, allowing for easy customization and portability. In the context of Node.js, environment variables provide a way to store and manage configurations, such as API keys, database credentials, and other settings, outside of your code.
Accessing these variables throughout your application code becomes straightforward with the process.env
property, allowing you to tailor your app’s functioning according to various environments.
Why use environment variables in Node.js?
Using environment variables in Node.js offers several advantages, including:
- improved security, by storing sensitive information outside of the codebase
- adaptability in configuring the application for various environments
- scalability in managing configuration settings
- easier collaboration with other developers or teams
Storing your configuration settings in environment variables lets you operate your application universally, eliminating the need to alter the code or rebuild it. This enhances the application’s encapsulation and maintainability.
Common use cases for environment variables
Environment variables are extremely versatile and can be used in a variety of scenarios. Some common use cases include configuring API keys, database credentials, and adjusting application behavior based on all the environment variables.
Environment-specific behavior can be particularly useful, allowing you to activate or deactivate certain features or adapt your application’s behavior according to the environment it’s running in, such as development, testing, or production.
Accessing Environment Variables in Node.js
Accessing environment variables in Node.js is simple and straightforward, thanks to the process.env
object. This global object contains key–value pairs of environment variables, with the values stored as strings.
The process.env object
The process.env
object is a global object that holds all environment variables as key–value pairs, with values stored as strings. When the Node.js process starts, it reads the environment variables set by the parent process or the operating system and stores them in the process.env
object. This makes it easy to access and use these variables throughout your application code.
Reading an environment variable
To read an environment variable in Node.js, simply access the corresponding property on the process.env
object. For example, suppose you have an environment variable named MY_VARIABLE
. In that case, you can access its value using process.env.MY_VARIABLE
. Keep in mind that the values read from environment variables are always strings, so if you need a different type, you’ll have to convert the value to the appropriate type.
Handling non-string types
Since environment variables are stored as strings, you might need to parse or convert them to their intended data type. For example, you can use JSON.parse
for objects or parseInt
for numbers. Appropriate management of non-string types ensures your application functions as intended, preventing potential problems related to data type inconsistencies.
Setting Environment Variables in Node.js
Setting environment variables in Node.js can be done within a script or from the command line, allowing for easy customization and configuration of your application. We’ll delve into both methods in this section and provide examples to help you commence.
Setting environment variables within a script
To set an environment variable within a Node.js script, you can assign a value to a property on the process.env
object. For example, if you want to set an environment variable named MY_VARIABLE
with the value my_value
, you can use the syntax process.env.MY_VARIABLE = 'my_value'
. This facilitates effortless tailoring of your application’s behavior and configurations right within your code.
Setting environment variables from the command line
Another way to set environment variables in Node.js is from the command line. On Linux and macOS, you can use the export
command, while on Windows, you can use the set
command.
For example, to set the MY_VARIABLE
environment variable to my_value
, you can run export MY_VARIABLE='my_value'
on Linux and macOS or set MY_VARIABLE=my_value
on Windows. This approach allows you to set environment variables before running your Node.js script, making it easy to customize your application’s behavior and configurations.
Working with .env Files
Managing environment variables directly within your code or from the command line can become cumbersome, especially for large applications with numerous variables. That’s where .env
files come into play. In this section, we’ll explore the advantages of using .env
files, the procedure for installing and setting up the dotenv package, and the method for loading custom environment variables from .env
files into your Node.js application.
Why use .env files?
.env
files provide a convenient way to store and manage environment variables for your Node.js application, keeping them organized and easily accessible. By storing environment variables in a .env
file, you can keep your application’s configuration settings and sensitive information, such as API keys and database credentials, separate from your codebase. This helps keep your code clean and maintainable while ensuring sensitive data is not accidentally exposed to the public.
Installing and configuring the dotenv package
To load environment variables from a .env
file, you’ll need to install and configure the dotenv package. The dotenv package is an npm package that enables you to read environment variables from a .env
file into your Node.js app.
To install the dotenv package, run npm install dotenv
in your project directory. Then, create a .env
file in the root directory of your application and add your environment variables, one per line.
Loading custom environment variables from .env files
Once you’ve installed and configured the dotenv package, you can load custom environment variables from your .env
file into your Node.js application. The dotenv package provides a config()
function that reads the environment variables from your .env
file and makes them available in your application through the process.env
object.
This enables smooth access to, and utilization of, custom environment variables throughout your application, easing configuration management and enhancing the maintainability of your code by using process global variable.
Managing Default and Required Environment Variables
In some cases, you might want to set default values for certain environment variables or ensure that specific variables are set before your application runs. In this section, we’ll discuss how to manage default and required environment variables in Node.js, including setting default values and handling cases where required variables are missing.
Setting default values for environment variables
Setting default values for environment variables can be done using the OR operator, like so: process.env.MY_VARIABLE || 'default_value'
. However, be cautious when using default values, as they can lead to unexpected behavior and make debugging more difficult.
If your application relies on a specific configuration, it’s generally better to ensure that the environment variables are properly set before running your application.
Handling required environment variables without default values
For required environment variables without default values, you can use methods like assert.ok()
or similar to ensure they’re properly set before continuing your application’s execution. Managing instances where required environment variables are absent helps prevent errors and guarantees the correct functioning of your application, even if certain variables aren’t supplied.
Best Practices for Environment Variables in Node.js
Now that we’ve covered the ins and outs of environment variables in Node.js, it’s essential to follow best practices to ensure your application is secure, maintainable, and efficient.
This section, we’ll outline some of the optimal practices for managing environment variables in Node.js, including strategies to avoid hardcoding values, maintaining the security of sensitive data, and arranging variables in a config module.
Avoiding hardcoding values
Hardcoding values in your Node.js application can lead to difficulties in managing and maintaining your code. By using environment variables to store and manage configurations and settings, you can keep your code clean, maintainable, and easily adjustable for different environments and use cases. This helps ensure your application is easy to update, debug, and deploy, making your development process more efficient and effective.
Keeping sensitive data secure
Sensitive data, such as API keys and database credentials, should be stored in environment variables to keep them secure and separate from your codebase. This ensures that sensitive data isn’t accidentally exposed through your source code or version control system, helping to protect your application and user data from unauthorized access.
Organizing environment variables in a config module
By organizing your environment variables in a config module, you can make them easily accessible and maintainable throughout your Node.js application. This allows you to centralize your application’s configuration settings, making it easier to update and manage them as your application evolves.
Additionally, a config module can help ensure that your environment variables are used consistently across your application, reducing the risk of configuration errors and making your code more maintainable.
Summary
In this article, we’ve explored the world of environment variables in Node.js, covering their benefits, use cases, and best practices for managing them effectively. We’ve seen how environment variables can help improve the security, maintainability, and flexibility of your Node.js applications, making it easier to run your app in different environments without having to modify the code itself.
As you continue your journey with Node.js, remember the importance of managing your application configurations and settings using environment variables. By following the best practices outlined above, you’ll be well on your way to building secure, maintainable, and efficient Node.js applications that can be easily deployed and run in a variety of environments.
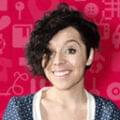
Dianne is SitePoint's newsletter editor. She especiallly loves learning about JavaScript, CSS and frontend technologies.