- The jqXHR (jQuery XMLHttpRequest) replaces the browser native XMLHttpRequest object.
- jQuery wraps the browser native XMLHttpRequest object with a superset API.
- The jQuery XMLHttpRequest (jqXHR) object is returned by the $.ajax() function.
- The jqXHR object simulates native XHR functionality where possible.
So what does it do? …
- It handles the HTTP request headers (Last-Modified, etag, Content-Type, MIME types etc…).
- It handles the callbacks of the request (including promise callbacks .done(), .fail() etc…)
- It handles any prefilters set for the request.
- It handles any timeouts set for the request.
- It handles any cross domain calls (including jsonp).
In the jQuery source code it is even commented as Fake xhr
// Fake xhr
jqXHR = {
...
}
jqZHR implements a Promise Interface
The jqXHR objects returned by $.ajax() implement the Promise interface. The object has all the properties, methods, and behavior of a Promise. Read more on deferred.promise().jqXHR Backward Compatibility with XMLHttpRequest
For backward compatibility with XMLHttpRequest, a jqXHR object will expose the following properties and methods: readyState, status, statusText, responseXML and/or responseText (with xml and/or text response, respectively), getAllResponseHeaders(), getResponseHeader(), abort() and setRequestHeader(). Since success, error, complete and statusCode cover all the requirements, jqXHR doesn’t provide any support for onreadystatechange.// Attach deferreds
deferred.promise( jqXHR ).complete = completeDeferred.add;
jqXHR.success = jqXHR.done;
jqXHR.error = jqXHR.fail;
Background info on XHR request
XMLHttpRequest (XHR) is an API available in web browser scripting languages such as JavaScript. It is used to send HTTP or HTTPS requests directly to a web server and load the server response data directly back into the script.
- XMLHttpRequest is a JavaScript object that was designed by Microsoft and adopted by Mozilla, Apple, and Google.
- Despite its name, XMLHttpRequest can be used to retrieve any type of data, not just XML, and it supports protocols other than HTTP (including file and ftp).
- XMLHttpRequest is subject to the browser’s same origin policy in that, for security reasons, requests will only succeed if they are made to the same server that served the original web page.
- The concept behind the XMLHttpRequest object was originally created by the developers of Outlook Web Access (by Microsoft) for Microsoft Exchange Server 2000.
Further Reading
- Taking a closer look at the jqxhr object
- Sharpkit.jQuery
- Latest jQuery Source Code
- Wikapedia XMLHttpRequest
- Mozilla XMLHttpRequest
Frequently Asked Questions (FAQs) about jQuery’s jqXHR Object
What is the main difference between jqXHR and the traditional AJAX request?
The jqXHR object is a superset of the browser’s native XMLHttpRequest object. It is returned by jQuery’s $.ajax() method and includes all properties, methods, and behaviors of the native XMLHttpRequest with additional features. The jqXHR object provides a consistent interface to manage AJAX requests and responses, including error handling, which is not available in the traditional AJAX request.
How can I handle errors using jqXHR?
The jqXHR object provides several methods to handle errors. The most common one is the .fail() method. This method is called when the request fails. It takes a function as an argument, which will be executed when the request fails. The function can take three parameters: jqXHR object, textStatus, and errorThrown, which provide details about the error.
Can I cancel a request using jqXHR?
Yes, you can cancel a request using the .abort() method of the jqXHR object. This method cancels the request if it has not yet completed. It’s important to note that not all requests can be cancelled. For example, requests that have already been sent to the server cannot be cancelled.
How can I get the status code of a request using jqXHR?
The jqXHR object provides the .status property, which contains the status code of the request. This property is useful to determine the result of the request. For example, a status code of 200 means the request was successful, while a status code of 404 means the requested resource was not found.
Can I use jqXHR with JSONP?
Yes, you can use jqXHR with JSONP. However, due to browser restrictions, error handling for JSONP requests is limited. The .fail() method will not be called if the request fails. Instead, you can use the .always() method, which is called regardless of whether the request succeeds or fails.
How can I chain multiple AJAX requests using jqXHR?
The jqXHR object returns a Promise, which can be used to chain multiple AJAX requests. You can use the .then() method of the Promise to chain the requests. The .then() method takes two functions as arguments: a function to be called when the request succeeds, and a function to be called when the request fails.
Can I use jqXHR with synchronous requests?
While it’s possible to use jqXHR with synchronous requests by setting the async option to false, it’s generally not recommended. Synchronous requests block the browser until the request completes, which can lead to a poor user experience. Instead, consider using asynchronous requests and the Promise interface provided by jqXHR.
How can I get the response data using jqXHR?
The jqXHR object provides the .responseText and .responseXML properties, which contain the response data as a string and as an XML document, respectively. You can also use the .done() method, which is called when the request succeeds. The function passed to the .done() method receives the response data as its first argument.
Can I set custom headers using jqXHR?
Yes, you can set custom headers using the .setRequestHeader() method of the jqXHR object. This method takes two arguments: the name of the header and the value to set. Note that due to browser restrictions, not all headers can be set.
How can I monitor the progress of a request using jqXHR?
The jqXHR object provides the .progress() method, which is called periodically while the request is being processed. This method takes a function as an argument, which receives an event object containing information about the progress of the request.
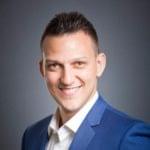
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.