How to declare switch statements using JavaScript. Pretty handy to know it will save you lots of time when executing different code depending the value of variable.
var jsLang = 'jquery';
switch (jsLang) {
case 'jquery':
alert('jQuery Wins!');
break;
case 'prototype':
alert('prototype Wins!');
break;
case 'mootools':
alert('mootools Wins!');
break;
case 'dojo':
alert('dojo Wins!');
break;
default:
alert('Nobody Wins!');
}
//outputs "jQuery Wins!"
You can also fall through to match multiple cases by omitting the breaks like so:
var jsLang = 'prototype';
switch (jsLang) {
case 'jquery':
alert('jQuery sucks!');
break;
case 'prototype':
alert('prototype sucks!');
case 'mootools':
alert('mootools sucks!');
case 'dojo':
alert('dojo sucks!');
break;
default:
alert('Nobody sucks!');
}
//outputs "prototype sucks! mootools sucks! dojo sucks!"
FAQs on Implementing and Utilizing jQuery Switch Statements in JavaScript
What is a jQuery Switch Statement?
A jQuery switch statement is a control flow statement that allows JavaScript to execute one block of code from many possibilities. It is a part of conditional statements in JavaScript, which also includes if-else and else if statements. The switch statement evaluates an expression and compares it with multiple cases. Once the match is found, the block of code associated with that case is executed.
How does a jQuery Switch Statement work?
The jQuery switch statement works by evaluating an expression once and then comparing the result with the values of different cases. If a match is found, the associated block of code is executed. If no match is found, the default case is executed. The break keyword is used to prevent the code from running into the next case unintentionally. It breaks out of the switch block once the matched case is executed.
How to use a jQuery Switch Statement?
To use a jQuery switch statement, you need to follow these steps:
1. Start with the switch keyword followed by an expression in parentheses.
2. Then, write the case keyword followed by the value you want to match with the expression and a colon.
3. Write the code you want to execute if the case matches the expression.
4. Use the break keyword to prevent the code from running into the next case.
5. Repeat steps 2-4 for as many cases as you want.
6. Finally, you can use the default keyword to specify the code to be executed if no case matches the expression.
What is the syntax of a jQuery Switch Statement?
The syntax of a jQuery switch statement is as follows:switch(expression) {
case value1:
// code to be executed if expression equals value1
break;
case value2:
// code to be executed if expression equals value2
break;
...
default:
// code to be executed if expression doesn't match any cases
}
The expression is evaluated once. The value of the expression is compared with the values of each case. If there is a match, the associated block of code is executed.
Can I use a jQuery Switch Statement without a break statement?
Yes, you can use a jQuery switch statement without a break statement. However, it’s not recommended because it can lead to code running into the next case unintentionally, which is known as fall-through. The break statement is used to prevent this from happening. It breaks out of the switch block once the matched case is executed.
What is the difference between a jQuery Switch Statement and an If-Else Statement?
The main difference between a jQuery switch statement and an if-else statement is how they make decisions. The switch statement evaluates an expression once and compares it with multiple cases, executing the block of code associated with the matching case. On the other hand, the if-else statement evaluates a condition and executes a block of code if the condition is true, otherwise, it executes another block of code.
Can I use a jQuery Switch Statement with strings?
Yes, you can use a jQuery switch statement with strings. The expression in the switch statement can be of any data type, including strings. The values of the cases must also be of the same data type as the expression.
How to use a jQuery Switch Statement with multiple cases?
To use a jQuery switch statement with multiple cases, you need to write multiple case statements within the switch block. Each case statement should have a unique value to match with the expression. If the expression matches the value of a case, the associated block of code is executed.
Can I use a jQuery Switch Statement without a default case?
Yes, you can use a jQuery switch statement without a default case. However, it’s recommended to include a default case as it will be executed if no case matches the expression. It acts as a fallback option.
How to use a jQuery Switch Statement with a default case?
To use a jQuery switch statement with a default case, you need to write the default keyword followed by a colon and the code you want to execute if no case matches the expression. The default case doesn’t need a break statement as it is always the last case in the switch block.
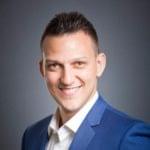
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.