Finding whether a string contains another string can be achieved not using jQuery but plain ole JavaScript! Here is how you do it.
if (str.indexOf("Yes") >= 0)
Note that this is case-sensitive. If you want a case-insensitive search, you can write
if (str.toLowerCase().indexOf("yes") >= 0)
//OR
if (/yes/i.test(str))
//OR
//You could use search or match for this.
str.search( 'Yes' )
Another example to check if a string contains another string.
if (v.indexOf("http:") == -1)
{
//string doesn't contain http
}
This will return the position of the match, or -1 if it isn’t found.
Frequently Asked Questions (FAQs) about jQuery String Functions
How can I check if a string contains a specific substring in jQuery?
In jQuery, you can use the indexOf()
method to check if a string contains a specific substring. This method returns the position of the first occurrence of a specified value in a string. If the value is not found, it returns -1. Here’s an example:var str = "Hello world!";
var n = str.indexOf("world");
In this example, n
will be 6 because “world” starts at position 6 in the string “Hello world!”.
What is the difference between indexOf()
and lastIndexOf()
in jQuery?
Both indexOf()
and lastIndexOf()
are used to find the position of a substring in a string. The difference lies in the direction they search in. indexOf()
starts searching from the beginning of the string and returns the position of the first occurrence of the specified substring. On the other hand, lastIndexOf()
starts searching from the end of the string and returns the position of the last occurrence of the specified substring.
How can I replace a substring in a string using jQuery?
You can use the replace()
method in jQuery to replace a substring in a string. This method searches a string for a specified value, or a regular expression, and returns a new string where the specified values are replaced. Here’s an example:var str = "Hello world!";
var newStr = str.replace("world", "jQuery");
In this example, newStr
will be “Hello jQuery!”.
How can I convert a string to lowercase or uppercase in jQuery?
You can use the toLowerCase()
and toUpperCase()
methods in jQuery to convert a string to lowercase or uppercase respectively. Here’s an example:var str = "Hello World!";
var lowerCaseStr = str.toLowerCase();
var upperCaseStr = str.toUpperCase();
In this example, lowerCaseStr
will be “hello world!” and upperCaseStr
will be “HELLO WORLD!”.
How can I split a string into an array of substrings in jQuery?
You can use the split()
method in jQuery to split a string into an array of substrings. This method splits a string into an array of substrings based on a specified separator. Here’s an example:var str = "Hello world!";
var arr = str.split(" ");
In this example, arr
will be [“Hello”, “world!”].
How can I join an array of strings into a single string in jQuery?
You can use the join()
method in jQuery to join an array of strings into a single string. This method joins all elements of an array into a string. Here’s an example:var arr = ["Hello", "world!"];
var str = arr.join(" ");
In this example, str
will be “Hello world!”.
How can I extract a part of a string in jQuery?
You can use the substring()
method in jQuery to extract a part of a string. This method extracts the characters from a string, between two specified indices, and returns the new sub string. Here’s an example:var str = "Hello world!";
var subStr = str.substring(0, 5);
In this example, subStr
will be “Hello”.
How can I remove whitespace from both ends of a string in jQuery?
You can use the trim()
method in jQuery to remove whitespace from both ends of a string. This method does not change the original string. Here’s an example:var str = " Hello world! ";
var trimmedStr = str.trim();
In this example, trimmedStr
will be “Hello world!”.
How can I find the length of a string in jQuery?
You can use the length
property in jQuery to find the length of a string. This property returns the number of characters in a string. Here’s an example:var str = "Hello world!";
var len = str.length;
In this example, len
will be 12.
How can I concatenate two strings in jQuery?
You can use the concat()
method in jQuery to concatenate two strings. This method joins two or more strings into one string. Here’s an example:var str1 = "Hello";
var str2 = "world!";
var str = str1.concat(" ", str2);
In this example, str
will be “Hello world!”.
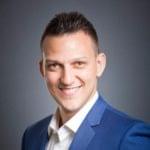
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.