Simple jQuery code snippet to find the index of an array item given the item value. It’s a bit like the .NET Array.FindIndex Method but use the in Array function.
//syntax
jQuery.inArray( value, array )
//example
jQuery.inArray("Sam", array)
Frequently Asked Questions about jQuery’s Find Index and Array Item
How does the findIndex() method work in JavaScript?
The findIndex() method in JavaScript is a built-in function that is used to find the index of the first element in an array that satisfies a provided testing function. It executes the function once for each index in the array until it finds one where the function returns a truthy value. If such an element is found, findIndex() immediately returns the index of that element. Otherwise, it returns -1.
What is the difference between findIndex() and indexOf() in JavaScript?
Both findIndex() and indexOf() are used to find the index of an element in an array. However, there are some key differences. The indexOf() method returns the first index at which a given element can be found in the array, or -1 if it is not present. It compares elements using strict equality (the same method used by the ===, or triple-equals, operator). On the other hand, findIndex() executes a callback function on each element in the array until the callback returns a truthy value, then it returns the index of that element. If no such element is found, it returns -1.
Can I use findIndex() with jQuery?
Yes, you can use findIndex() with jQuery. However, it’s important to note that jQuery does not have a built-in findIndex() method. Instead, you can use the $.inArray() method, which is similar to JavaScript’s indexOf() method. If you want to use findIndex() with jQuery, you would need to convert your jQuery object to a JavaScript array first.
How can I use findIndex() with an array of objects?
You can use findIndex() with an array of objects by providing a callback function that checks for a certain condition. The callback function should return true for the object you’re looking for and false for all other objects. Here’s an example:let array = [{id: 1, name: 'John'}, {id: 2, name: 'Jane'}, {id: 3, name: 'Joe'}];
let index = array.findIndex(obj => obj.id === 2);
console.log(index); // Outputs: 1
What happens if there are multiple elements that satisfy the testing function in findIndex()?
If there are multiple elements that satisfy the testing function in findIndex(), the method will return the index of the first element that satisfies the function. It will not continue checking the remaining elements in the array.
Can I use findIndex() on a string?
No, you cannot use findIndex() on a string. The findIndex() method is only available on Array objects. If you want to find the index of a character or substring in a string, you can use the indexOf() or lastIndexOf() methods.
Is findIndex() supported in all browsers?
The findIndex() method is not supported in Internet Explorer. However, it is supported in all modern browsers, including Chrome, Firefox, Safari, and Edge.
Can I use findIndex() with a multidimensional array?
Yes, you can use findIndex() with a multidimensional array. However, you would need to provide a callback function that can handle the nested arrays. The callback function would need to iterate over the sub-arrays and check for the condition you’re looking for.
How can I use findIndex() to find the last element that satisfies a condition?
The findIndex() method only returns the index of the first element that satisfies the provided testing function. If you want to find the last element that satisfies a condition, you would need to reverse the array first using the reverse() method, and then call findIndex().
Can I use findIndex() with a typed array?
Yes, you can use findIndex() with a typed array. A typed array is a JavaScript array-like object and provides a mechanism for accessing raw binary data. Just like with a regular array, you can use findIndex() to find the index of the first element in a typed array that satisfies a provided testing function.
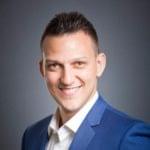
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.