Quick jQuery snippet to reverse each to loop over elements backwards. Example shows effect on loop over a list backwards.
$($("li").get().reverse()).each(function() { /* ... */ });
Frequently Asked Questions (FAQs) about jQuery .each() Reverse
How can I reverse the order of elements in an array using jQuery .each()?
To reverse the order of elements in an array using jQuery .each(), you can use the .get() method to convert the jQuery object into a true JavaScript array. Then, you can use the .reverse() method to reverse the order of the elements. Here’s an example:var arr = $('div').get();
arr.reverse();
$.each(arr, function(i, el){
console.log(el);
});
In this example, ‘div’ is the selector that selects all div elements on the page. The .get() method is used to convert the jQuery object into a true JavaScript array. The .reverse() method is then used to reverse the order of the elements in the array. Finally, the .each() method is used to iterate over the reversed array.
What is the difference between .each() and .reverse() in jQuery?
The .each() and .reverse() methods in jQuery serve different purposes. The .each() method is used to iterate over a set of elements in the jQuery object. It executes a function for each matched element. On the other hand, the .reverse() method is used to reverse the order of the elements in an array. However, it’s important to note that .reverse() is not a jQuery method, but a JavaScript method. Therefore, to use .reverse() on a jQuery object, you first need to convert it into a true JavaScript array using the .get() method.
Can I use .each() method to iterate over an array in reverse order?
Yes, you can use the .each() method to iterate over an array in reverse order. However, the .each() method itself does not provide a way to reverse the order of iteration. To achieve this, you need to first reverse the order of the elements in the array using the .reverse() method, and then use the .each() method to iterate over the reversed array. Here’s an example:var arr = [1, 2, 3, 4, 5];
arr.reverse();
$.each(arr, function(i, el){
console.log(el);
});
In this example, the .reverse() method is used to reverse the order of the elements in the array, and then the .each() method is used to iterate over the reversed array.
How can I reverse the order of DOM elements using jQuery?
To reverse the order of DOM elements using jQuery, you can use the .get() method to convert the jQuery object into a true JavaScript array. Then, you can use the .reverse() method to reverse the order of the elements. Finally, you can use the .each() method to iterate over the reversed array and the .appendTo() method to rearrange the DOM elements in the reversed order. Here’s an example:var arr = $('div').get();
arr.reverse();
$.each(arr, function(i, el){
$(el).appendTo('body');
});
In this example, ‘div’ is the selector that selects all div elements on the page. The .get() method is used to convert the jQuery object into a true JavaScript array. The .reverse() method is then used to reverse the order of the elements in the array. Finally, the .each() method is used to iterate over the reversed array, and the .appendTo() method is used to rearrange the DOM elements in the reversed order.
Why is .reverse() not working on my jQuery object?
The .reverse() method is a JavaScript method, not a jQuery method. Therefore, it works on true JavaScript arrays, not on jQuery objects. If you try to use the .reverse() method on a jQuery object, it will not work and will throw an error. To use the .reverse() method on a jQuery object, you first need to convert it into a true JavaScript array using the .get() method. Here’s an example:var arr = $('div').get();
arr.reverse();
In this example, ‘div’ is the selector that selects all div elements on the page. The .get() method is used to convert the jQuery object into a true JavaScript array. Then, the .reverse() method is used to reverse the order of the elements in the array.
Can I use .each() method to iterate over a reversed array without modifying the original array?
Yes, you can use the .each() method to iterate over a reversed array without modifying the original array. To achieve this, you can use the .slice() method to create a copy of the array, and then use the .reverse() method to reverse the order of the elements in the copied array. Here’s an example:var arr = [1, 2, 3, 4, 5];
var reversedArr = arr.slice().reverse();
$.each(reversedArr, function(i, el){
console.log(el);
});
In this example, the .slice() method is used to create a copy of the array. The .reverse() method is then used to reverse the order of the elements in the copied array. Finally, the .each() method is used to iterate over the reversed array.
How can I use .each() method to iterate over a reversed array in a single line of code?
To use the .each() method to iterate over a reversed array in a single line of code, you can chain the .get(), .reverse(), and .each() methods together. Here’s an example:$.each($('div').get().reverse(), function(i, el){ console.log(el); });
In this example, ‘div’ is the selector that selects all div elements on the page. The .get() method is used to convert the jQuery object into a true JavaScript array. The .reverse() method is then used to reverse the order of the elements in the array. Finally, the .each() method is used to iterate over the reversed array.
Can I use .each() method to iterate over a reversed array of DOM elements?
Yes, you can use the .each() method to iterate over a reversed array of DOM elements. To achieve this, you can use the .get() method to convert the jQuery object into a true JavaScript array, the .reverse() method to reverse the order of the elements in the array, and the .each() method to iterate over the reversed array. Here’s an example:var arr = $('div').get();
arr.reverse();
$.each(arr, function(i, el){
console.log(el);
});
In this example, ‘div’ is the selector that selects all div elements on the page. The .get() method is used to convert the jQuery object into a true JavaScript array. The .reverse() method is then used to reverse the order of the elements in the array. Finally, the .each() method is used to iterate over the reversed array.
How can I use .each() method to iterate over a reversed array and perform a specific action for each element?
To use the .each() method to iterate over a reversed array and perform a specific action for each element, you can pass a function to the .each() method. This function will be executed for each element in the reversed array. Here’s an example:var arr = [1, 2, 3, 4, 5];
arr.reverse();
$.each(arr, function(i, el){
console.log('Element ' + i + ' is ' + el);
});
In this example, the .reverse() method is used to reverse the order of the elements in the array. Then, the .each() method is used to iterate over the reversed array. The function passed to the .each() method takes two parameters: the index of the current element and the current element itself. This function is executed for each element in the reversed array, and it logs a message to the console that includes the index and value of the current element.
Can I use .each() method to iterate over a reversed array of objects?
Yes, you can use the .each() method to iterate over a reversed array of objects. To achieve this, you can use the .reverse() method to reverse the order of the elements in the array, and the .each() method to iterate over the reversed array. Here’s an example:var arr = [{name: 'John'}, {name: 'Jane'}, {name: 'Joe'}];
arr.reverse();
$.each(arr, function(i, el){
console.log(el.name);
});
In this example, the .reverse() method is used to reverse the order of the elements in the array of objects. Then, the .each() method is used to iterate over the reversed array. The function passed to the .each() method takes two parameters: the index of the current element and the current element itself. This function is executed for each element in the reversed array, and it logs the name property of the current object to the console.
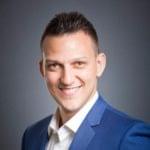
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.