jQuery Code Snippet to disable the mouse right click as often used with websites who want to add some low level security. jQuery can do this with a bind to the contextmenu event. I reccommend only using this function if you have to as it is generally bad practice.
$(function() {
$(this).bind("contextmenu", function(e) {
e.preventDefault();
});
});
Frequently Asked Questions (FAQs) about Disabling Mouse Clicks Using jQuery
How can I disable the right-click function using jQuery?
Disabling the right-click function using jQuery is quite simple. You need to use the ‘contextmenu’ event in jQuery, which is triggered when the right mouse button is clicked. Here is a simple code snippet that disables the right-click function:$(document).ready(function(){
$(document).bind("contextmenu",function(e){
return false;
});
});
This code binds the ‘contextmenu’ event to the document and returns false when the event is triggered, effectively disabling the right-click function.
Can I disable the right-click function for specific elements only?
Yes, you can disable the right-click function for specific elements only. Instead of binding the ‘contextmenu’ event to the document, you can bind it to a specific element. For example, to disable right-click on images, you can use the following code:$('img').bind('contextmenu', function(e) {
return false;
});
This code binds the ‘contextmenu’ event to all ‘img’ elements and returns false when the event is triggered, effectively disabling the right-click function on images.
Is it possible to display a custom message when the right-click function is disabled?
Yes, it is possible to display a custom message when the right-click function is disabled. You can use the ‘alert’ function in JavaScript to display a custom message. Here is an example:$(document).ready(function(){
$(document).bind("contextmenu",function(e){
alert("Right-click is disabled");
return false;
});
});
This code displays an alert message saying “Right-click is disabled” when the right-click function is triggered.
Can I disable other mouse events using jQuery?
Yes, you can disable other mouse events using jQuery. For example, to disable the double-click event, you can use the ‘dblclick’ event in jQuery. Here is an example:$(document).ready(function(){
$(document).bind("dblclick",function(e){
return false;
});
});
This code binds the ‘dblclick’ event to the document and returns false when the event is triggered, effectively disabling the double-click function.
Is it a good practice to disable the right-click function?
Disabling the right-click function can be useful in some cases, such as preventing users from downloading images or copying text. However, it can also be annoying for users who are used to using the right-click function for other purposes, such as opening links in new tabs. Therefore, it is important to consider the user experience before deciding to disable the right-click function.
Can users bypass the right-click function being disabled?
Yes, users can bypass the right-click function being disabled by using keyboard shortcuts or by disabling JavaScript in their browser. Therefore, while disabling the right-click function can deter casual users, it is not a foolproof method of preventing users from copying content or downloading images.
Can I disable the right-click function for specific browsers only?
Disabling the right-click function using jQuery will affect all browsers that support jQuery. However, you can use JavaScript to detect the user’s browser and disable the right-click function for specific browsers only. This requires more advanced JavaScript knowledge and is beyond the scope of this article.
Can I disable the right-click function on mobile devices?
The right-click function is not commonly used on mobile devices, as most mobile devices use touch events instead of mouse events. However, you can use jQuery to disable touch events in a similar way to disabling mouse events. This requires more advanced jQuery knowledge and is beyond the scope of this article.
Can I disable the right-click function without using jQuery?
Yes, you can disable the right-click function without using jQuery by using plain JavaScript. Here is an example:document.oncontextmenu = function(e) {
e.preventDefault();
};
This code sets the ‘oncontextmenu’ event handler of the document to a function that prevents the default action of the event, effectively disabling the right-click function.
Can I enable the right-click function after it has been disabled?
Yes, you can enable the right-click function after it has been disabled by unbinding the ‘contextmenu’ event. Here is an example:$(document).unbind('contextmenu');
This code unbinds the ‘contextmenu’ event from the document, effectively enabling the right-click function.
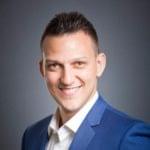
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.