jQuery code snippet to detect if a user is viewing the website using a mobile device, specifically an iPhone iPod or iPad. You may also wish to detect the mobile browser type.
jQuery(document).ready(function($){
var deviceAgent = navigator.userAgent.toLowerCase();
var agentID = deviceAgent.match(/(iphone|ipod|ipad)/);
if (agentID) {
// mobile code here
}
});
Frequently Asked Questions on Detecting Mobile Devices with jQuery
How can I use jQuery to detect a mobile device?
jQuery is a powerful JavaScript library that can be used to detect mobile devices. The first step is to include the jQuery library in your HTML file. You can do this by adding a script tag with the source attribute pointing to the jQuery library. Once you have jQuery included in your project, you can use the navigator.userAgent
property to detect the user’s device. This property returns a string that contains information about the name, version, and platform of the browser. By using jQuery’s indexOf()
function, you can check if this string contains certain keywords that are associated with mobile devices, such as ‘Mobile’, ‘iPhone’, ‘Android’, etc.
Can I use jQuery to differentiate between different types of mobile devices?
Yes, you can use jQuery to differentiate between different types of mobile devices. The navigator.userAgent
property not only allows you to detect if a user is on a mobile device, but it also provides information about the specific type of device. For example, if you want to check if a user is on an iPhone, you can look for the keyword ‘iPhone’ in the navigator.userAgent
string. Similarly, you can check for ‘iPad’, ‘Android’, etc., to detect other types of devices.
What are the limitations of using jQuery for mobile device detection?
While jQuery provides a simple and convenient way to detect mobile devices, it does have some limitations. The main limitation is that it relies on the navigator.userAgent
property, which can be easily spoofed or manipulated by the user. This means that it may not always provide accurate results. Additionally, jQuery’s device detection capabilities are limited to the information provided by the navigator.userAgent
property, which may not include all types of mobile devices.
Can I use jQuery to detect the orientation of a mobile device?
Yes, you can use jQuery to detect the orientation of a mobile device. The window.orientation
property provides information about the orientation of the device. You can use jQuery’s resize()
function to listen for changes in the window size, which will be triggered when the device’s orientation changes. By checking the value of the window.orientation
property, you can determine if the device is in portrait or landscape mode.
How can I use jQuery to optimize my website for mobile devices?
jQuery can be used to optimize your website for mobile devices in several ways. First, you can use it to detect if a user is on a mobile device and then serve a mobile-optimized version of your website. You can also use it to detect the orientation of the device and adjust the layout of your website accordingly. Additionally, jQuery provides a number of plugins, such as jQuery Mobile, that are specifically designed to enhance the user experience on mobile devices.
Is jQuery the only way to detect mobile devices?
No, jQuery is not the only way to detect mobile devices. There are several other methods available, such as CSS media queries and server-side device detection. Each method has its own advantages and disadvantages, and the best method to use depends on your specific needs and requirements.
How reliable is jQuery for mobile device detection?
While jQuery provides a simple and convenient way to detect mobile devices, it is not always 100% reliable. This is because it relies on the navigator.userAgent
property, which can be easily spoofed or manipulated by the user. Therefore, while jQuery can be a useful tool for mobile device detection, it should not be relied upon as the sole method of detection.
Can I use jQuery to detect specific features of a mobile device?
jQuery does not provide a built-in way to detect specific features of a mobile device, such as the presence of a touchscreen or the device’s screen resolution. However, there are several jQuery plugins available that can provide this functionality. Additionally, you can use the navigator.userAgent
property to detect certain features that are associated with specific types of devices.
How can I use jQuery to detect a mobile device’s operating system?
You can use the navigator.userAgent
property to detect a mobile device’s operating system. This property returns a string that contains information about the name, version, and platform of the browser. By checking for certain keywords in this string, you can determine the device’s operating system. For example, you can check for ‘iPhone OS’ to detect iOS devices, or ‘Android’ to detect Android devices.
Can I use jQuery to detect a mobile device’s browser?
Yes, you can use jQuery to detect a mobile device’s browser. The navigator.userAgent
property provides information about the name and version of the browser. By checking for certain keywords in this string, you can determine the device’s browser. For example, you can check for ‘Safari’ to detect Safari browsers, or ‘Chrome’ to detect Chrome browsers.
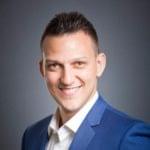
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.