obj = $('p'); // Get all paragraphs into a jQuery object
for (i = 0; i < obj.length; i++) {
// Do something with obj[i]
}
As you can see, jQuery objects have .length and []. But, .pop() and .reverse() are missing. And you may want to pass the array to a function that expects a native array. So do this:
obj = $('p'); // Get all paragraphs
a = $.makeArray(obj); // One way to do it
a = obj.toArray(); // The other way
also try:
$.each(piv, function(name, value)
{
console.log(name, value);
});
or this
for (var key in piv) {
// var obj = piv[key];
console.log(key, piv[key]);
}
Also see: http://api.jquery.com/jQuery.makeArray/
Frequently Asked Questions (FAQs) about Converting jQuery Objects to Arrays
What is the difference between jQuery.makeArray() and toArray() in jQuery?
Both jQuery.makeArray() and toArray() are used to convert an object into an array in jQuery. However, there is a subtle difference between the two. jQuery.makeArray() is a jQuery utility function that takes an array-like object and transforms it into a true JavaScript array. On the other hand, toArray() is a method that belongs to the jQuery object prototype, and it returns a JavaScript array of the selected DOM elements.
How can I convert a JavaScript object to an array using jQuery?
You can convert a JavaScript object to an array using the jQuery.map() function. This function translates all items in an array or object to new array elements. Here is a simple example:var obj = { "One": 1, "Two": 2, "Three": 3, "Four": 4 };
var arr = $.map(obj, function(value, index) {
return [value];
});
console.log(arr); // Output: [1, 2, 3, 4]
Can I convert a jQuery object to an array without using jQuery?
Yes, you can convert a jQuery object to an array without using jQuery. JavaScript provides several methods to do this, such as Array.from() and the spread operator (…). Here’s an example using Array.from():var $elements = $('.myClass'); // jQuery object
var elements = Array.from($elements); // JavaScript array
What is the use of jQuery.makeArray()?
The jQuery.makeArray() function is used to convert an array-like object into a true JavaScript array. This can be useful when you want to use methods that exist only for arrays, such as pop() or push(), on an array-like object.
How can I convert a multidimensional object to an array in jQuery?
Converting a multidimensional object to an array in jQuery can be achieved using the $.map() function. However, because the object is multidimensional, you would need to use a nested $.map() function to access the inner objects.
Can I convert a jQuery object to an array in JavaScript?
Yes, you can convert a jQuery object to an array in JavaScript. You can use the Array.from() method or the spread operator (…) to achieve this.
What is the difference between a jQuery object and a JavaScript array?
A jQuery object is an array-like object that contains DOM elements selected by the jQuery selector function $(). A JavaScript array, on the other hand, is a high-level, list-like object that is a collection of data.
How can I convert an array to a jQuery object?
You can convert an array to a jQuery object by passing the array to the jQuery function $(). Here’s an example:var arr = [document.getElementById('id1'), document.getElementById('id2')];
var $elements = $(arr);
Can I use jQuery.map() to convert a JavaScript object to an array?
Yes, you can use jQuery.map() to convert a JavaScript object to an array. The jQuery.map() function translates all items in an array or object to new array elements.
How can I convert a jQuery object to an array in jQuery?
You can convert a jQuery object to an array in jQuery using the .toArray() method. This method returns a JavaScript array of the selected DOM elements.
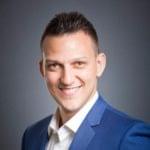
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.