A jQuery code snippet to check if field is read only.
//if field is not read only
if($(this).attr('disabled'))
{
...
}
Frequently Asked Questions (FAQs) about jQuery Check Field Read
How can I check if a field is read-only using jQuery?
To check if a field is read-only in jQuery, you can use the .attr() method. This method returns undefined for attributes that have not been set. To check if an input field is read-only, you can use the following code:if ($('#myInput').attr('readonly') !== undefined) {
// The input field is read-only
} else {
// The input field is not read-only
}
In this code, ‘#myInput’ is the ID of the input field you want to check. If the field is read-only, the .attr() method will return ‘readonly’, otherwise it will return undefined.
What is the difference between .prop() and .attr() in jQuery?
The .prop() method gets the property value for only the first element in the matched set. It returns undefined for values of undefined properties. The .attr() method gets the attribute value for only the first element in the matched set. It returns undefined for attributes that have not been set.
In the context of checking if a field is read-only, both .prop() and .attr() can be used. However, .prop() is recommended as it correctly retrieves the property values for boolean attributes such as readonly.
How can I make a field read-only using jQuery?
To make a field read-only in jQuery, you can use the .attr() method and set the ‘readonly’ attribute to ‘readonly’. Here is an example:$('#myInput').attr('readonly', 'readonly');
In this code, ‘#myInput’ is the ID of the input field you want to make read-only.
Can I toggle the read-only state of a field using jQuery?
Yes, you can toggle the read-only state of a field using jQuery. You can use the .prop() method to get the current state, and then set the opposite state. Here is an example:$('#myInput').prop('readonly', !$('#myInput').prop('readonly'));
In this code, ‘#myInput’ is the ID of the input field you want to toggle.
How can I check if a field is read-only without using jQuery?
If you want to check if a field is read-only without using jQuery, you can use plain JavaScript. Here is an example:if (document.getElementById('myInput').readOnly) {
// The input field is read-only
} else {
// The input field is not read-only
}
In this code, ‘myInput’ is the ID of the input field you want to check.
How can I remove the read-only attribute from a field using jQuery?
To remove the read-only attribute from a field using jQuery, you can use the .removeAttr() method. Here is an example:$('#myInput').removeAttr('readonly');
In this code, ‘#myInput’ is the ID of the input field you want to remove the read-only attribute from.
Can I use jQuery to check if a field is read-only on page load?
Yes, you can use jQuery to check if a field is read-only on page load. You can use the .ready() method to run code as soon as the document is ready (when the page has finished loading). Here is an example:$(document).ready(function() {
if ($('#myInput').prop('readonly')) {
// The input field is read-only
} else {
// The input field is not read-only
}
});
In this code, ‘#myInput’ is the ID of the input field you want to check.
How can I check if a field is read-only using jQuery on form submission?
You can use jQuery to check if a field is read-only on form submission. You can use the .submit() method to attach a function to the submit event of the form. Here is an example:$('#myForm').submit(function() {
if ($('#myInput').prop('readonly')) {
// The input field is read-only
} else {
// The input field is not read-only
}
});
In this code, ‘#myForm’ is the ID of the form and ‘#myInput’ is the ID of the input field you want to check.
Can I use jQuery to check if a field is read-only when the field value changes?
Yes, you can use jQuery to check if a field is read-only when the field value changes. You can use the .change() method to attach a function to the change event of the field. Here is an example:$('#myInput').change(function() {
if ($(this).prop('readonly')) {
// The input field is read-only
} else {
// The input field is not read-only
}
});
In this code, ‘#myInput’ is the ID of the input field you want to check.
Can I use jQuery to check if a field is read-only when the field is clicked?
Yes, you can use jQuery to check if a field is read-only when the field is clicked. You can use the .click() method to attach a function to the click event of the field. Here is an example:$('#myInput').click(function() {
if ($(this).prop('readonly')) {
// The input field is read-only
} else {
// The input field is not read-only
}
});
In this code, ‘#myInput’ is the ID of the input field you want to check.
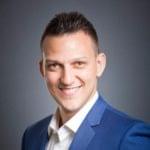
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.