Unfortunately jQuery can’t be used to change the title of a web page. However the same effect can be achieved using plain JavaScript. JavaScript code snippet to change the current web page full title (this is the title you see on your browser header). Simple.
document.title = 'new title';
Frequently Asked Questions (FAQs) about Changing Page Title with jQuery
How can I change the title of a specific page using jQuery?
To change the title of a specific page using jQuery, you need to use the ‘document.title’ property. This property allows you to get or set the text in the title bar. Here’s a simple example of how you can use it:$(document).ready(function(){
document.title = "New Page Title";
});
In this code, ‘New Page Title’ is the new title you want to set for your page. This code should be placed within the script tags in your HTML file.
Can I change the title of a page dynamically with jQuery?
Yes, you can change the title of a page dynamically with jQuery. This can be useful in situations where you want to update the title based on user interaction or other events. Here’s an example:$(document).ready(function(){
$('#button').click(function(){
document.title = "New Page Title";
});
});
In this code, the page title changes to ‘New Page Title’ when the user clicks on the element with the id ‘button’.
Is it possible to change the title of an external page with jQuery?
No, it’s not possible to change the title of an external page with jQuery due to the same-origin policy. This policy restricts how a document or script loaded from one origin can interact with a resource from another origin. It’s a critical security mechanism for isolating potentially malicious documents.
Can I use jQuery to change the title of a page in a specific browser tab?
Yes, you can use jQuery to change the title of a specific browser tab. The ‘document.title’ property changes the title of the current document, which is displayed in the browser tab. Here’s an example:$(document).ready(function(){
document.title = "New Tab Title";
});
In this code, ‘New Tab Title’ is the new title you want to set for your browser tab.
How can I revert back to the original title after changing it with jQuery?
To revert back to the original title after changing it with jQuery, you need to store the original title in a variable before changing it. Here’s an example:$(document).ready(function(){
var originalTitle = document.title;
document.title = "New Page Title";
// To revert back to the original title
document.title = originalTitle;
});
In this code, the original title is stored in the ‘originalTitle’ variable. You can then use this variable to revert back to the original title.
Can I change the title of a page based on a specific condition with jQuery?
Yes, you can change the title of a page based on a specific condition with jQuery. This can be useful in situations where you want to update the title based on certain conditions. Here’s an example:$(document).ready(function(){
if(condition){
document.title = "New Page Title";
}
});
In this code, the page title changes to ‘New Page Title’ if the condition is true.
Can I use jQuery to change the title of a page in all browser tabs?
No, you can’t use jQuery to change the title of a page in all browser tabs. The ‘document.title’ property only changes the title of the current document, which is displayed in the current browser tab.
Can I change the title of a page without using jQuery?
Yes, you can change the title of a page without using jQuery. You can use pure JavaScript to achieve this. Here’s an example:document.title = "New Page Title";
In this code, ‘New Page Title’ is the new title you want to set for your page.
Can I use jQuery to change the title of a page on page load?
Yes, you can use jQuery to change the title of a page on page load. You can use the ‘ready’ method to achieve this. Here’s an example:$(document).ready(function(){
document.title = "New Page Title";
});
In this code, the page title changes to ‘New Page Title’ when the page loads.
Can I use jQuery to change the title of a page on a button click?
Yes, you can use jQuery to change the title of a page on a button click. You can use the ‘click’ method to achieve this. Here’s an example:$('#button').click(function(){
document.title = "New Page Title";
});
In this code, the page title changes to ‘New Page Title’ when the user clicks on the element with the id ‘button’.
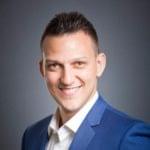
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.