//monitor the closing of a popup window
var win = window.open("http://dev.com/index.php?m=social&a=testLinkedIn",'','height=500,width=500');
var winTimer = window.setInterval(function()
{
if (win.closed !== false)
{
// !== is required for compatibility with Opera
window.clearInterval(winTimer);
log('win closed...');
}
}, 200);
Frequently Asked Questions about jQuery Popups
How can I create a simple popup using jQuery?
Creating a simple popup using jQuery involves a few steps. First, you need to create a div in your HTML that will act as the popup. This div should be hidden by default. Next, you need to write a jQuery function that will show this div when a certain event occurs, such as clicking a button. You can use the .show() method to make the div visible. Finally, you can use the .hide() method to hide the popup when the user clicks outside of it or on a close button.
Why is my jQuery popup not closing when I click outside of it?
If your jQuery popup is not closing when you click outside of it, it’s likely because you haven’t written the necessary code to detect this event. You can use the .hide() method in combination with the .on() method to hide the popup when a click event occurs outside of the popup div.
How can I generate a popup that closes automatically after a few seconds?
To create a popup that closes automatically after a few seconds, you can use the setTimeout function in JavaScript. This function allows you to execute a piece of code after a specified amount of time. You can use it to call the .hide() method on your popup div after a few seconds.
How can I add a fade effect to my jQuery popup?
jQuery provides several methods to add effects to your elements, including .fadeIn() and .fadeOut(). These methods gradually change the opacity of the element, creating a fade effect. You can use these methods instead of .show() and .hide() to add a fade effect to your popup.
How can I center my jQuery popup on the screen?
Centering a popup on the screen involves setting the top and left CSS properties to 50%, and then subtracting half the width and height of the popup. You can do this using the .css() method in jQuery.
How can I prevent the user from scrolling the page when the popup is open?
You can prevent scrolling by adding overflow: hidden to the body element when the popup is open. You can do this using the .css() method in jQuery.
How can I add a close button to my jQuery popup?
To add a close button to your popup, you can create a button element in your HTML and position it within your popup div. Then, you can write a jQuery function that hides the popup when this button is clicked.
How can I make my jQuery popup responsive?
Making your popup responsive involves setting its width and height to a percentage of the screen size instead of a fixed number of pixels. You can do this using the .css() method in jQuery.
How can I add a background overlay when the popup is open?
To add a background overlay, you can create a div that covers the entire screen and is positioned behind your popup. You can show this div when the popup is open and hide it when the popup is closed.
How can I animate the opening and closing of my jQuery popup?
jQuery provides several methods to animate elements, including .slideDown(), .slideUp(), and .slideToggle(). You can use these methods instead of .show() and .hide() to animate the opening and closing of your popup.
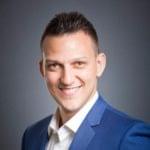
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.