This jQuery utility function checks if a paramter is present in the current page URL and if it doesn’t exist it adds it and returns the url in full. Could be useful if you needed to do an ajax request to update a database with new form data and simply want to redirect to the same url but with an updated flag to show an updated box.
(function($,W,D)
{
var JQUERY4U = {};
JQUERY4U.UTIL =
{
/**
* Add a parameter to url if doesn't already exist
* @param param - the parameter to add
* @param value - the value of the parameter
* @return url - the url with the appended parameter
*/
addParamToUrl: function(param, value)
{
//check if param exists
var result = new RegExp(param + "=([^&]*)", "i").exec(W.location.search);
result = result && result[1] || "";
//added seperately to append ? before params
var loc = W.location;
var url = loc.protocol + '//' + loc.host + loc.pathname + loc.search;
//param doesn't exist in url, add it
if (result == '')
{
//doesn't have any params
if (loc.search == '')
{
url += "?" + param + '=' + value;
}
else
{
url += "&" + param + '=' + value;
}
}
//return the finished url
return url;
}
}
//example usage
var updatedUrl = JQUERY4U.UTIL.addParamToUrl('updated', 'true');
console.log(updatedUrl);
//input: http://jquery4u.com/index.php
//output: http://jquery4u.com/index.php?updated=true
})(jQuery, window, document);
Frequently Asked Questions (FAQs) about jQuery Add Param URL Function
How can I add multiple parameters to a URL using jQuery?
Adding multiple parameters to a URL using jQuery is quite straightforward. You can use the $.param()
method, which creates a serialized representation of an array or an object. Here’s an example:var data = { param1: "value1", param2: "value2" };
var url = "http://example.com/page?" + $.param(data);
In this example, $.param(data)
will generate a string “param1=value1¶m2=value2”, which is then appended to the URL.
How can I remove a parameter from a URL using jQuery?
jQuery does not provide a built-in method to remove parameters from a URL. However, you can achieve this by using JavaScript’s URL
and URLSearchParams
objects. Here’s an example:var url = new URL("http://example.com/page?param1=value1¶m2=value2");
url.searchParams.delete('param1');
In this example, the delete
method of URLSearchParams
object is used to remove ‘param1’ from the URL.
How can I check if a URL contains a specific parameter using jQuery?
You can use the URLSearchParams
object of JavaScript to check if a URL contains a specific parameter. Here’s an example:var url = new URL("http://example.com/page?param1=value1¶m2=value2");
var hasParam1 = url.searchParams.has('param1');
In this example, the has
method of URLSearchParams
object is used to check if ‘param1’ exists in the URL.
How can I change the value of a specific parameter in a URL using jQuery?
You can use the URL
and URLSearchParams
objects of JavaScript to change the value of a specific parameter in a URL. Here’s an example:var url = new URL("http://example.com/page?param1=value1¶m2=value2");
url.searchParams.set('param1', 'newValue1');
In this example, the set
method of URLSearchParams
object is used to change the value of ‘param1’ in the URL.
How can I add a parameter to a URL without reloading the page using jQuery?
You can use the history.pushState
method of the window
object to add a parameter to a URL without reloading the page. Here’s an example:var url = new URL(window.location.href);
url.searchParams.append('param1', 'value1');
window.history.pushState({}, '', url);
In this example, the append
method of URLSearchParams
object is used to add ‘param1’ to the URL, and then history.pushState
is used to update the URL in the browser without reloading the page.
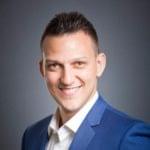
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.