I’ve had quite a lot of requests for how to upload images using Ajax and show a thumbnail so I decided to do a quick demo to show you how it can be done. The Script facilitates the process of uploading an image via Ajax and using PHP to create a thumbnail, return the image source and display to the user as a thumbnail of the image uploaded. All without the page reloading. To help you understand how it works I’ve commented on a few of the key parts below.
View Project on Git
The Upload Image Function
This function sends the image to the backend and receives back data about the uploaded image and it’s created thumbnail. It uses the ajaxFileUpload function.uploadImage: function()
{
var _this = this,
$imgInput = $('#image-upload');
this.cache.$imgPreview.hide();
this.cache.$imgOriginal.hide();
$('.img-data').remove(); //remove any previous image data
$.ajaxFileUpload(
{
url: _this.settings.uploadImageUrl,
secureuri: false,
fileElementId: 'image-upload',
dataType: "json",
success: function(data)
{
console.log(data);
_this.cache.$imgPreview.attr('src',data.thumb.img_src);
_this.cache.$imgOriginal.attr('src',data.master.img_src);
//show img data
_this.cache.$imgPreview.after('<div class="img-data">'+$.objToString(data.thumb)+'</div>');
_this.cache.$imgOriginal.after('<div class="img-data">'+$.objToString(data.master)+'</div>');
$('#remove-image-upload').show();
},
error: function(xhr, textStatus, errorThrown)
{
console.log(xhr, textStatus, errorThrown + 'error');
return false;
},
complete: function()
{
//hide loading image
_this.cache.$form.find('.loading').hide();
_this.cache.$imgPreview.show();
_this.cache.$imgOriginal.show();
}
});
}
The Image Thumbnail
The image thumbnail src gets updated with the new thumbnail image src once the image has been uploaded.<!-- Generated Image Thumbnail -->
<img alt="image preview " src="img/350x150.jpg" id="image-thumb"/>
Example Form Submit
The form data can be grabbed as normal and the thumbnail src is added to the data which is sent as part of the form submit.submitForm: function()
{
/* example of submitting the form */
var $theForm = $('#submit-plugin-form'),
formData = $theForm.serialize(); //get form data
//include video thumb src
formData += '&image-thumb=' + $('#image-thumb').attr('src');
$theForm.find(':input').attr('disabled', 'disabled'); //lock form
$.ajax(
{
type: "POST",
url: 'php/submitForm.php',
dataType: "json",
data: formData,
success: function(ret)
{
//...
},
error: function(xhr, textStatus, errorThrown)
{
console.log(xhr, textStatus, errorThrown + 'error');
return false;
}
});
}
The backend PHP Script
I have written a backend script in PHP which receives an image from front end and uploads it, creates a thumbnail and returns both the master and thumb image info as JSON. View PHP Script.Folder Structure
The uploaded image and thumb are stored in a temp folder then when the form is submitted the images are moved and renamed into the main images folder.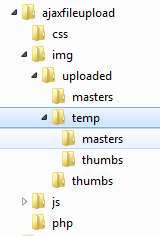
Naming of Files
The temp files are named using a timestamp and thumbs with their dimension.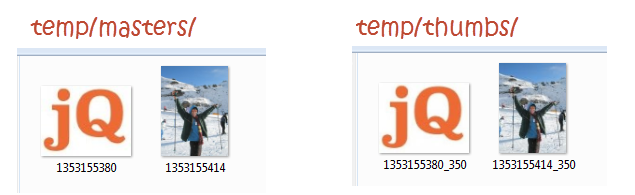
Security
You’ll need to make sure the images directory has writable permissions 774 should be high enough.Frequently Asked Questions (FAQs) about Image Upload
How can I upload multiple images at once using PHP and AJAX?
Uploading multiple images at once can be achieved by modifying the input field in your HTML form to accept multiple files. You can do this by adding the ‘multiple’ attribute to your input tag. In your PHP script, you will need to loop through the $_FILES superglobal array to handle each uploaded file individually. AJAX can be used to submit the form without refreshing the page, enhancing user experience.
How can I display a progress bar during image upload?
A progress bar can be implemented using AJAX’s ‘progress’ event. This event is triggered periodically during the file upload process, allowing you to calculate and display the percentage of the upload that has been completed. You can then use this percentage to update a progress bar element in your HTML.
How can I validate the file type and size before uploading?
File type and size validation can be performed in both client-side JavaScript and server-side PHP. In JavaScript, you can access the file’s type and size through the File API. In PHP, these properties can be accessed through the $_FILES superglobal array. You can then compare these values to your allowed file types and maximum file size to determine whether the file should be uploaded.
How can I handle errors during the image upload process?
Error handling can be implemented in your PHP script by checking the ‘error’ property of each file in the $_FILES superglobal array. This property will be 0 if the file was uploaded successfully, and a different value if an error occurred. You can then use a switch statement to handle each possible error code and provide a helpful error message to the user.
How can I resize images before uploading them?
Resizing images before upload can help to reduce the load on your server and speed up the upload process. This can be achieved using the HTML5 Canvas API in combination with JavaScript’s File API. You can create a new canvas element, draw the image onto it at the desired size, and then convert the canvas back to a Blob object for upload.
How can I upload images without using a form?
Images can be uploaded without a form using the Drag and Drop API in combination with AJAX. You can create a drop zone in your HTML where users can drag and drop their files, and then use JavaScript to handle the ‘drop’ event, read the dropped files, and send them to the server via AJAX.
How can I secure my image upload script?
Securing your image upload script is crucial to prevent malicious files from being uploaded to your server. This can be achieved by validating the file type, checking the file’s MIME type, limiting the file size, and renaming the file before saving it to your server.
How can I save uploaded images to a database?
Saving uploaded images to a database can be achieved by first moving the uploaded file to a temporary directory on your server using the move_uploaded_file() function in PHP. You can then insert a new record into your database that includes the file’s name and the path to the file on your server.
How can I display uploaded images on my website?
Displaying uploaded images can be achieved by querying your database for the file paths of the uploaded images, and then using these paths to create img elements in your HTML.
How can I delete uploaded images from my server?
Deleting uploaded images can be achieved by using the unlink() function in PHP. This function takes a file path as its argument and deletes the file at that path. You should also remove the corresponding record from your database to keep it in sync with your file system.
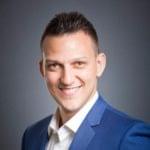
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.