Many years ago, when first learning to program, I was fascinated by John Conway’s life-form simulation “Game of Life”. Over the years there have been many variants, written in different languages, on a multitude of platforms. Whatever the language chosen, coding ones own version of Game of Life was a rite of passage for any budding programmer. That’s what I propose to demonstrate in this article. However, with today’s ubiquitous presence of the browser and associated programming languages, we can dispense with the traditional coding environments and languages. Instead we can learn some logic coding and array handling by writing a version of Game of Life to run in a browser.
The Game Board
The game board consists of a grid of cells. Each cell can be logically on or off, meaning a life-form is present in that cell or the cell is empty. The grid can be any size we choose, but typically a 10 x 10 grid is the starting point for beginners. Also, we will start with a pre-determined grid, or seed generation, rather than enter one using mouse or keyboard input. The following grid shows the seed generation we will use, which will result in an oscillating population in just eleven generations.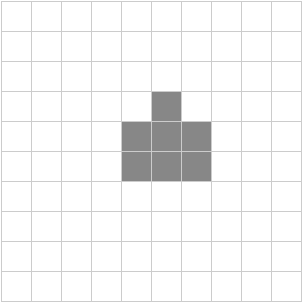
Rules of Propagation
The rules of propagation can be made as complex as you like, but in this minimalist implementation of the game we’ll be using the simplest of rules:- If a cell is empty and there are exactly three neighbors, populate the cell.
- If a cell is populated and there are fewer than two or greater than three neighbors, empty the cell.
adjacent = countAdjacent(i, j);
switch (generationThis[i][j]) {
case 0:
if ( (adjacent == 3) ) {
generationNext[i][j] = 1;
}
break;
case 1:
if ( (adjacent == 2) || (adjacent == 3) ) {
generationNext[i][j] = 1;
}
}
An additional aspect of simplicity for this demo is to have just one life-form. More than one life-form, each with its own color, would make for a very interesting simulation, but would make the increase in complexity of the coding unsuitable for this demo.
Generations
There is a range of possible outcomes from this life-form simulation:- Extinction.
- Steady state population.
- Oscillating population.
- Open-ended population change.
- If the new generation is completely empty, the life-form is extinct.
- If adjacent generations are identical, the population is steady.
- If the next and previous generations are identical, the population is oscillating.
User Interface
HTML, CSS and JavaScript are all that’s required for the Game of Life. This example uses theCANVAS
element to display the grid. A grid of DIV
elements could equally be used, as could a TABLE
, but let’s bring Game of Life up to date by the use of HTML5 and CSS3.
All that’s needed for the game board is a canvas element and a button to cause the next generation to be calculated.
<form>
<p>Generation: <span id="generation"></span> <span id="status"></span></p>
<canvas id="gameboard"></canvas>
<input type="button" value="generate next" id="btnNext">
</form>
The seed generation can be calculated once the page has loaded, after which each button press will progress the game to the next generation. As an alternative to using a button, the calculation of each successive generation could be automated by the use of JavaScript’s setTimeout()
function. This, however, is better left until we’re sure the code is functioning correctly and we know we can trap the three generation states listed above.
<script src="gameoflife.js"></script>
<script>
$(document).ready(function() {
seedGeneration();
$("#btnNext").click(function(){
nextGeneration();
checkStatus();
copyGrids();
drawGeneration();
});
});
</script>
And that is all that’s needed for a minimalist implementation of Game of Life in a browser. The full code for this article, which includes the gameoflife.js
file, is available for download.
An improvement to the interface would be to provide interactive user input to set the cells for the seed generation, but that’s beyond the scope of this demo.
Further Reading
dmoz open directory project’s Game of Life Game of Life newsSummary
In this article we have covered the essential elements of writing a browser-based version of John Conway’s “Game of Life”. Using nothing more than HTML, CSS and JavaScript, we have seen how to create a simple simulation that runs natively in a browser, a simulation that traditionally was written in languages such as BASIC and Pascal.Frequently Asked Questions (FAQs) about Conway’s Game of Life
How does Conway’s Game of Life work?
Conway’s Game of Life is a cellular automaton invented by British mathematician John Horton Conway. It’s a zero-player game, meaning its evolution is determined by its initial state and doesn’t require any further input. The game takes place on an infinite two-dimensional grid of square cells, each of which is in one of two possible states, live or dead. Every cell interacts with its eight neighbors, which are the cells horizontally, vertically, or diagonally adjacent. At each step in time, the following transitions occur: Any live cell with fewer than two live neighbors dies, any live cell with two or three live neighbors lives on to the next generation, any live cell with more than three live neighbors dies, and any dead cell with exactly three live neighbors becomes a live cell.
What are the rules of Conway’s Game of Life?
The rules of Conway’s Game of Life are simple yet create complex patterns. They are based on the concept of birth, death, and survival, represented by the cells in the game. The rules are as follows: 1) Any live cell with fewer than two live neighbors dies, as if by underpopulation. 2) Any live cell with two or three live neighbors lives on to the next generation. 3) Any live cell with more than three live neighbors dies, as if by overpopulation. 4) Any dead cell with exactly three live neighbors becomes a live cell, as if by reproduction.
How can I start playing Conway’s Game of Life?
To start playing Conway’s Game of Life, you need to set up an initial configuration of live cells on the grid. This configuration evolves according to the rules of the game, without any further input from you. You can create your initial configuration by clicking on the cells in the grid to toggle them between live and dead states. Once you’re satisfied with your setup, click the ‘Start’ button to begin the game. You can pause the game at any time to examine the current state of the grid.
What are some interesting patterns in Conway’s Game of Life?
Conway’s Game of Life is known for its ability to generate a wide variety of complex patterns. Some of these include ‘Still Lifes’, which do not change from one generation to the next, ‘Oscillators’, which cycle through a set of states, and ‘Spaceships’, which move across the grid. The most famous pattern is probably the ‘Glider’, a small spaceship that moves diagonally across the grid.
Can Conway’s Game of Life simulate a Turing machine?
Yes, Conway’s Game of Life is Turing complete, meaning it can simulate a Turing machine. This was proven by constructing a pattern known as a ‘universal constructor’, which can create any pattern given a suitable initial state. This means that, in theory, the Game of Life could perform any computation that a computer can, given enough time and space.
Is there an end to Conway’s Game of Life?
There is no definitive end to Conway’s Game of Life. The game continues indefinitely, with each generation evolving from the previous one according to the rules of the game. However, many patterns eventually reach a stable state where they either become static or start repeating the same sequence of states over and over. In such cases, the game can be considered to have reached an end state.
Can I create my own patterns in Conway’s Game of Life?
Absolutely! Part of the fun of Conway’s Game of Life is experimenting with different initial configurations and seeing what patterns they evolve into. You can create your own patterns by setting up the initial state of the cells on the grid. Try creating a few live cells in a row, or a small cluster of live cells, and see what happens!
What is the significance of Conway’s Game of Life in computer science?
Conway’s Game of Life has had a significant impact on computer science and mathematics. It’s a simple model that can generate complex behaviors, making it a useful tool for studying emergent phenomena. It’s also a classic example of a cellular automaton, a concept that has applications in many areas of computer science, including cryptography, image processing, and the simulation of physical systems.
Can Conway’s Game of Life be played on a finite grid?
While Conway’s Game of Life is traditionally played on an infinite grid, it can also be played on a finite grid. However, the behavior of the game can be quite different in this case. For example, patterns that move across the grid, like spaceships, can’t exist on a finite grid. Also, the edges of the grid can have a significant impact on the evolution of the game.
Are there variations of Conway’s Game of Life?
Yes, there are many variations of Conway’s Game of Life. These include games with different rules, games played on different types of grids (like hexagonal or three-dimensional grids), and games with more than two states per cell. These variations can produce a wide range of interesting and complex behaviors, further expanding the richness of the Game of Life universe.
David is a web developer based in England. He is an experienced programmer having developed software for various platforms including 8-bit CPUs, corporate mainframes, and most recently the Web. His preference is for simplicity and efficiency, avoiding where possible software that's complex, bloated, or closed.