jQuery code snippet to make capitals of the first letter of every word in the string. This could be used to prevent users from entering all caps for titles or text when they input data into forms.
//usage
$("input").keyup(function() {
toUpper(this);
});
//function
function toUpper(obj) {
var mystring = obj.value;
var sp = mystring.split(' ');
var wl=0;
var f ,r;
var word = new Array();
for (i = 0 ; i < sp.length ; i ++ ) {
f = sp[i].substring(0,1).toUpperCase();
r = sp[i].substring(1).toLowerCase();
word[i] = f+r;
}
newstring = word.join(' ');
obj.value = newstring;
return true;
}
Frequently Asked Questions (FAQs) about Capitalizing the First Letter of a Word in JavaScript
How can I capitalize the first letter of a string in JavaScript using the slice method?
The slice method in JavaScript can be used to capitalize the first letter of a string. This method extracts parts of a string and returns the extracted parts in a new string. Here’s how you can use it:function capitalizeFirstLetter(string) {
return string.charAt(0).toUpperCase() + string.slice(1);
}
In this function, charAt(0)
selects the first character of the string, toUpperCase()
converts this character to uppercase, and slice(1)
selects the rest of the string. The function then returns the concatenation of the uppercase first letter and the rest of the string.
Can I use the toUpperCase method to capitalize the first letter of every word in a string?
Yes, you can use the toUpperCase
method in combination with the split
and map
methods to capitalize the first letter of every word in a string. Here’s how:function capitalizeWords(string) {
return string.split(' ').map(function(word) {
return word.charAt(0).toUpperCase() + word.slice(1);
}).join(' ');
}
In this function, split(' ')
breaks the string into an array of words, map
applies a function to each word, and join(' ')
combines the words back into a string.
What is the difference between the toUpperCase and toLocaleUpperCase methods in JavaScript?
The toUpperCase
method converts all the lowercase characters in a string to uppercase. The toLocaleUpperCase
method does the same, but it takes into account the host environment’s current locale. For most languages, toUpperCase
and toLocaleUpperCase
will yield the same result. However, for languages that have language-specific case mappings, like Turkish, the results may differ.
How can I capitalize the first letter of a string without using the toUpperCase method?
You can capitalize the first letter of a string without using the toUpperCase
method by using ASCII values. Each character in JavaScript has a corresponding ASCII value. The ASCII values for lowercase letters are 97-122 and for uppercase letters are 65-90. Here’s how you can do it:function capitalizeFirstLetter(string) {
let firstLetter = string.charCodeAt(0);
if (firstLetter >= 97 && firstLetter <= 122) {
firstLetter = firstLetter - 32;
}
return String.fromCharCode(firstLetter) + string.slice(1);
}
In this function, charCodeAt(0)
gets the ASCII value of the first character of the string. If this value is between 97 and 122 (the ASCII values for lowercase letters), the function subtracts 32 to get the ASCII value for the uppercase version of the letter. The function then returns the concatenation of the uppercase first letter and the rest of the string.
How can I capitalize the first letter of a string in JavaScript using regular expressions?
Regular expressions (regex) provide a powerful way to manipulate strings in JavaScript. You can use a regex to capitalize the first letter of a string as follows:function capitalizeFirstLetter(string) {
return string.replace(/^(.)/, function(match) {
return match.toUpperCase();
});
}
In this function, the regex ^(.)
matches the first character of the string. The replace
method then replaces this character with its uppercase version.
How can I capitalize the first letter of a string in JavaScript using the substring method?
The substring
method in JavaScript extracts characters from a string between two specified indices. You can use this method to capitalize the first letter of a string as follows:function capitalizeFirstLetter(string) {
return string.substring(0, 1).toUpperCase() + string.substring(1);
}
In this function, substring(0, 1)
selects the first character of the string, toUpperCase()
converts this character to uppercase, and substring(1)
selects the rest of the string. The function then returns the concatenation of the uppercase first letter and the rest of the string.
How can I capitalize the first letter of a string in JavaScript using the charAt method?
The charAt
method in JavaScript returns the character at a specified index in a string. You can use this method to capitalize the first letter of a string as follows:function capitalizeFirstLetter(string) {
return string.charAt(0).toUpperCase() + string.slice(1);
}
In this function, charAt(0)
selects the first character of the string, toUpperCase()
converts this character to uppercase, and slice(1)
selects the rest of the string. The function then returns the concatenation of the uppercase first letter and the rest of the string.
How can I capitalize the first letter of a string in JavaScript using the split method?
The split
method in JavaScript splits a string into an array of substrings. You can use this method to capitalize the first letter of a string as follows:function capitalizeFirstLetter(string) {
let stringArray = string.split('');
stringArray[0] = stringArray[0].toUpperCase();
return stringArray.join('');
}
In this function, split('')
splits the string into an array of characters. The function then converts the first character to uppercase and joins the characters back into a string.
How can I capitalize the first letter of a string in JavaScript using the replace method?
The replace
method in JavaScript replaces some or all matches of a pattern in a string. You can use this method to capitalize the first letter of a string as follows:function capitalizeFirstLetter(string) {
return string.replace(/^./, string[0].toUpperCase());
}
In this function, the regex ^.
matches the first character of the string. The replace
method then replaces this character with its uppercase version.
How can I capitalize the first letter of a string in JavaScript using the concat method?
The concat
method in JavaScript concatenates two or more strings. You can use this method to capitalize the first letter of a string as follows:function capitalizeFirstLetter(string) {
return string[0].toUpperCase().concat(string.slice(1));
}
In this function, toUpperCase()
converts the first character of the string to uppercase, and slice(1)
selects the rest of the string. The function then concatenates the uppercase first letter and the rest of the string.
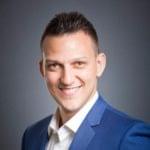
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.