When using opacity to fade in or fade out over time using jQuery.animate, you’ll run into font rendering issues with all versions of Internet Explorer. This leaves you with 2 options: Use jQuery’s opacity(‘show’) or opacity(‘hide’) shortcut properties which handle IE’s shortcomings, or Set the filter css property to ‘none’ once you’ve completed your animation Obviously, if you’re fading something in from being completely hidden, option 1 makes sense, otherwise you’ve got no choice but to use option 2. I came across this as I had font rendering issues for Cruiseabout in tabs content that was being caused by my use of opacity in FCL.TABS. As a result, I’ve patched FCL.TABS to use opacity’s “show” and “hide” properties instead of “0? and “1? and the problem has been fixed.
Broken code
$tabContent.css('opacity', 0);
$tabContent.animate(
{
opacity: 1
}, 350);
Fixed code
$tabContent.css('opacity', 'hide');
$tabContent.animate(
{
opacity: 'show'
}, 350);
Frequently Asked Questions about Animating Opacity with jQuery Animate
How Can I Use jQuery Animate to Fade an Element to a Specific Opacity?
To fade an element to a specific opacity using jQuery animate, you need to select the element and use the .animate() method. The .animate() method allows you to create custom animations. You can specify the CSS property you want to animate and the duration of the animation. For example, to fade an element to 50% opacity over 2 seconds, you would use the following code:$("#element").animate({
opacity: 0.5
}, 2000);
In this code, “#element” is the ID of the element you want to animate, “opacity: 0.5” sets the final opacity to 50%, and “2000” sets the duration of the animation to 2 seconds.
What is the Difference Between jQuery fadeTo() and animate()?
Both fadeTo() and animate() methods in jQuery can be used to change the opacity of an element. The main difference between the two is that fadeTo() is specifically designed to change the opacity, while animate() is a more general function that can animate any CSS property.
The fadeTo() method takes two arguments: the duration of the fade and the final opacity. For example, to fade an element to 50% opacity over 2 seconds, you would use the following code:$("#element").fadeTo(2000, 0.5);
On the other hand, the animate() method can be used to animate multiple CSS properties at once. It takes an object as an argument, where each property-value pair represents a CSS property and its final value. For example, to animate both the opacity and the width of an element, you would use the following code:$("#element").animate({
opacity: 0.5,
width: "50%"
}, 2000);
In this code, “opacity: 0.5” sets the final opacity to 50%, “width: “50%”” sets the final width to 50% of its original size, and “2000” sets the duration of the animation to 2 seconds.
How Can I Animate the Opacity of an Element on Mouse Hover Using jQuery?
To animate the opacity of an element on mouse hover using jQuery, you can use the hover() method in combination with the animate() method. The hover() method takes two functions as arguments: one to execute when the mouse enters the element, and one to execute when the mouse leaves the element.
For example, to fade an element to 50% opacity when the mouse hovers over it, and then fade it back to 100% opacity when the mouse leaves, you would use the following code:$("#element").hover(
function() {
$(this).animate({opacity: 0.5}, 2000);
},
function() {
$(this).animate({opacity: 1}, 2000);
}
);
In this code, “#element” is the ID of the element you want to animate, “opacity: 0.5” sets the final opacity to 50% when the mouse enters the element, “opacity: 1” sets the final opacity to 100% when the mouse leaves the element, and “2000” sets the duration of each animation to 2 seconds.
Can I Use jQuery Animate to Fade an Element In and Out Continuously?
Yes, you can use jQuery animate to continuously fade an element in and out. To do this, you can create a custom function that fades the element out and then calls itself when the animation is complete to fade the element back in. This creates a loop that continues until the page is refreshed or the function is stopped.
Here’s an example of how you can do this:function fadeInOut() {
$("#element").animate({opacity: 0}, 2000, function() {
$(this).animate({opacity: 1}, 2000, fadeInOut);
});
}
fadeInOut();
In this code, “#element” is the ID of the element you want to animate, “opacity: 0” fades the element out, “opacity: 1” fades the element back in, “2000” sets the duration of each animation to 2 seconds, and “fadeInOut” is the name of the function that is called when each animation is complete.
How Can I Stop a jQuery Animate Opacity Animation?
To stop a jQuery animate opacity animation, you can use the stop() method. The stop() method stops the currently running animation on the selected element.
For example, to stop the animation on an element with the ID “element”, you would use the following code:$("#element").stop();
This code will immediately stop the current animation on the element. If you want to stop all animations on the element, you can pass true as the first argument to the stop() method:$("#element").stop(true);
This code will stop all animations on the element, not just the current one.
Can I Use jQuery Animate to Change the Opacity of a Background Image?
Unfortunately, you cannot directly change the opacity of a background image using jQuery animate. The opacity property in CSS applies to the entire element, not just the background image. This means that if you animate the opacity of an element, all of its content (including text and child elements) will also become transparent.
However, there is a workaround. You can create a separate div for the background image and animate the opacity of that div. This way, the opacity of the background image can be changed without affecting the opacity of the other content.
Here’s an example of how you can do this:<div id="background"></div>
<div id="content">Your content here</div>
#background {
position: absolute;
width: 100%;
height: 100%;
background-image: url('your-image.jpg');
}
#content {
position: relative;
}
$("#background").animate({opacity: 0.5}, 2000);
In this code, “#background” is the ID of the div with the background image, “#content” is the ID of the div with the content, “opacity: 0.5” sets the final opacity to 50%, and “2000” sets the duration of the animation to 2 seconds.
How Can I Use jQuery Animate to Fade an Element to a Specific Opacity Over a Specific Duration?
To fade an element to a specific opacity over a specific duration using jQuery animate, you need to select the element and use the .animate() method. The .animate() method allows you to create custom animations. You can specify the CSS property you want to animate, the final value of that property, and the duration of the animation.
For example, to fade an element to 50% opacity over 5 seconds, you would use the following code:$("#element").animate({
opacity: 0.5
}, 5000);
In this code, “#element” is the ID of the element you want to animate, “opacity: 0.5” sets the final opacity to 50%, and “5000” sets the duration of the animation to 5 seconds.
Can I Use jQuery Animate to Fade Multiple Elements at Once?
Yes, you can use jQuery animate to fade multiple elements at once. To do this, you need to select all the elements you want to animate and use the .animate() method. The .animate() method will apply the animation to each selected element.
For example, to fade all elements with the class “fade” to 50% opacity over 2 seconds, you would use the following code:$(".fade").animate({
opacity: 0.5
}, 2000);
In this code, “.fade” is the class of the elements you want to animate, “opacity: 0.5” sets the final opacity to 50%, and “2000” sets the duration of the animation to 2 seconds.
How Can I Use jQuery Animate to Fade an Element to a Specific Opacity and Then Back to Its Original Opacity?
To fade an element to a specific opacity and then back to its original opacity using jQuery animate, you can use the .animate() method twice in a row. The first .animate() method will fade the element to the specific opacity, and the second .animate() method will fade it back to its original opacity.
For example, to fade an element to 50% opacity and then back to 100% opacity over 2 seconds each, you would use the following code:$("#element").animate({
opacity: 0.5
}, 2000).animate({
opacity: 1
}, 2000);
In this code, “#element” is the ID of the element you want to animate, “opacity: 0.5” sets the final opacity to 50% for the first animation, “opacity: 1” sets the final opacity to 100% for the second animation, and “2000” sets the duration of each animation to 2 seconds.
Can I Use jQuery Animate to Fade an Element to a Specific Opacity When a Button is Clicked?
Yes, you can use jQuery animate to fade an element to a specific opacity when a button is clicked. To do this, you need to select the button and use the .click() method. The .click() method takes a function as an argument, which is executed when the button is clicked.
For example, to fade an element to 50% opacity when a button with the ID “button” is clicked, you would use the following code:$("#button").click(function() {
$("#element").animate({
opacity: 0.5
}, 2000);
});
In this code, “#button” is the ID of the button, “#element” is the ID of the element you want to animate, “opacity: 0.5” sets the final opacity to 50%, and “2000” sets the duration of the animation to 2 seconds.
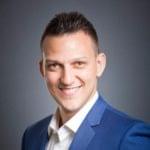
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.