In any development process, errors are something that you cannot avoid, no matter how competent and thorough you are when writing your code.
As a WordPress developer, it is your job to make sure any of your code errors are being handled correctly without affecting the end user. WordPress is shipped with a basic error handling class, namely WP_Error
that can be used and integrated into your code for basic error handling.
In this tutorial, we will take a look at the basic anatomy of the WP_Error
class, how it works and most importantly, we will cover how to integrate the WP_Error
class within our application.
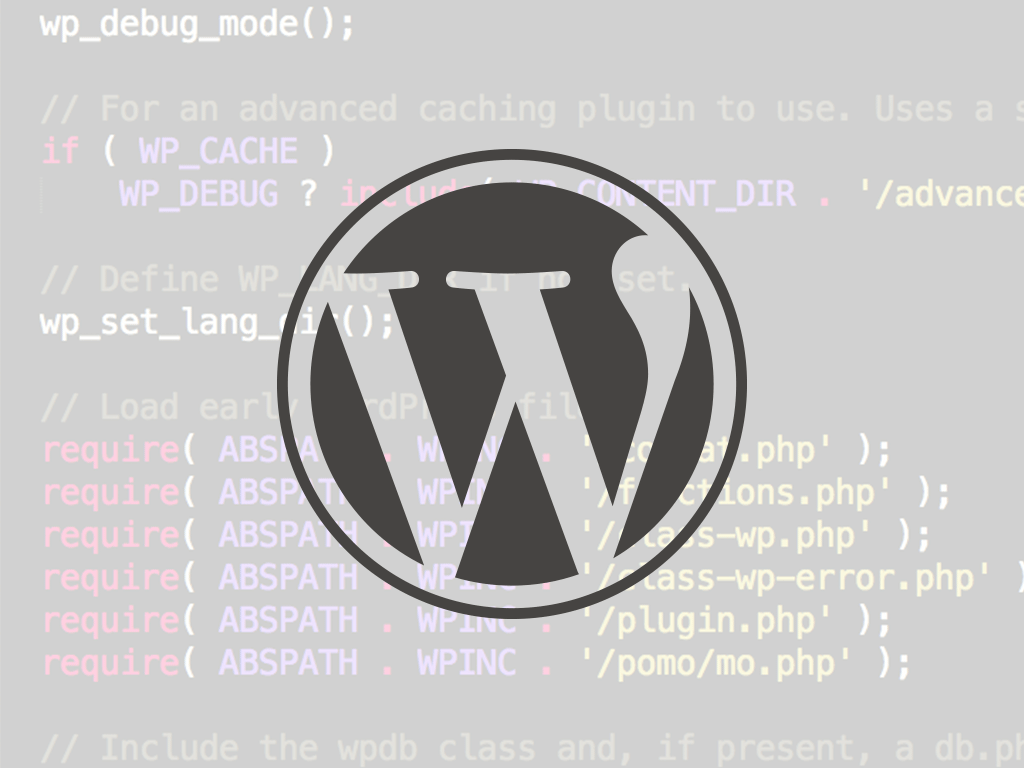
What’s Inside
The structure of the WP_Error
class is actually really simple and yet powerful enough to be used as an error handling mechanism for your plugin.
The whole source code for the WP_Error
class can be found in the wp-includes/class-wp-error.php
file. Let’s take a look at its properties and the methods available.
Properties
WP_Error
only has two private properties namely $errors
and $error_data
. $errors
is used to store related error messages while $error_data
can be used optionally to store related data that you want to access later on. WP_Error
uses a simple key-value pair to store the related errors and data into the object, so the key defined in WP_Error
must be unique to avoid it overwriting the previously defined key.
Methods
WP_Error
comes with various methods that are ultimately modifying the two properties it contains. Let’s take a look at a few of them.
get_error_codes
– Returns all the available error codes for that specificWP_Error
instance. If you only need the first error code, there is another separate function calledget_error_code
.get_error_messages( $code )
– If$code
is not supplied, the function will simply return all the stored messages in that specificWP_Error
instance. Again, if you only need to return a message for a specific error code, simply useget_error_message( $code )
.add( $code, $message, $data )
– This function is particularly useful when you want to modify the errors stored in the instantiated.WP_Error
object. Note that even$message
and$data
are not compulsory, the$errors
variable will still be populated.add_data( $data, $code)
– If you intend to only modify the$error_data
property, this function can be used. Note that the$code
argument comes second, opposite of what we have with theadd
method. If no$code
is supplied, the error data will be added to the first error code instead.remove( $code )
– This is a rather new method added recently in WordPress 4.1 that removes all the associated error messages and data from the specific key.
Function
How can you know if a specific variable or data returned from a function is an instance of the WP_Error
? Well, there is a utility function that you can simply check that, called is_wp_error
which return true or false depending on the variable given.
is_wp_error( $thing )
– Return true if$thing
is aWP_Error
instance, false otherwhise.
Implement Your Own
It is not enough to just to know how the WP_Error
internally works, you also need to learn how to implement it well within your own application. Let’s walk through a few examples to get a better idea on how it works.
Handling Errors Returned by WordPress Core Function
WordPress offers a lot of utility functions that can be used to speed up our development process. Most of the functions are also well equipped with basic error handling capabilities that we can use.
As an example, wp_remote_post
is a very helpful function that we can use to make a remote POST request to a specific URL. However, we cannot expect that the remote URL is always accessible or our request is always successful. What we do know from the codex page is that this function will return the WP_Error
on failure. This knowledge will help us along the way to implement error handling correctly in our application.
Take a look at this snippet.
// Make a responseo to remote url $url
$response = wp_remote_post( $url, array(
'timeout' => 30,
'body' => array( 'foo' => 'bar' )
)
);
if ( is_wp_error( $response ) ) {
echo 'ERROR: ' . $response->get_error_message();
} else {
// do something
}
As you can see, we are executing a remote POST request to a $url
. However, we do not simply take the $response
data as it is, but instead, we do a little checking with our handy is_wp_error
function that we covered earlier. If everything is fine, then only we can proceed with what we want to do with our $response
.
Returning a Custom Error in Your Own Application
Let’s say you have a custom function that handles a contact form submission called handle_form_submission
. Assuming, we have a custom form setup somewhere, let’s see how we can make the function better by implementing our own error handling functionality.
function handle_form_submission() {
// do your validation, nonce and stuffs here
// Instantiate the WP_Error object
$error = new WP_Error();
// Make sure user supplies the first name
if ( empty( $_POST['first_name'] ) ) {
$error->add( 'empty', 'First name is required' );
}
// And last name too
if ( empty( $_POST['last_name'] ) ) {
$error->add( 'empty', 'Last name is required' );
}
// Check for email address
if ( empty( $_POST['email'] ) ) {
$error->add( 'empty', 'Last name is required' );
} elseif ( ! is_email( $_POST['email'] ) ) {
$error->add( 'invalid', 'Email address must be valid' );
}
// Lastly, check for the message
if ( empty( $_POST['message'] ) ) {
$error->add( 'empty', 'Your message is required' );
}
// Send the result
if ( empty( $error->get_error_codes() ) ) {
return $error;
}
// Everything is fine
return true;
}
Of course, you will need to implement your own sanitization and validation in that functions as well, but that is out of scope for this tutorial. Now, knowing that we are correctly returning the WP_Error
instance on error, we can use it to supply a more meaningful error message to the end user.
Assuming you have a specific part in your application to display the form submission error, you can do this:
$result = handle_form_submission();
if ( is_wp_error( $result ) ) {
echo '<p>Ops, something went wrong. Please rectify this errors</p>';
echo '<ul>';
echo '<li>' . implode( '</li><li>', $result->get_error_messages() ) . '</li>';
echo '</ul>';
} else {
// Everything went well
}
External References
- Class Referece/WP_Error on WordPress Codex
- Introduction to the WP_Error Class by Pippin Williamson
- Why WP_Error Sucks by Ryan McCue
Conclusion
Perfecting your craft in software development also means knowing what to do when your code fails to do what it is supposed to do, and making sure that your application can handle it gracefully.
As far as WordPress goes, using the WP_Error
class that is shipped with it provides a rather simple yet powerful implementation of error handling that you can integrate it within your application.
What are your thoughts? How are you using the WP_Error
in your daily development process? Please share it with us below.
Frequently Asked Questions (FAQs) about the WP_Error Class
What is the WP_Error class in WordPress and why is it important?
The WP_Error class is a core part of WordPress. It’s a way for WordPress to handle errors within the system. This class is used to contain and manage error messages from different parts of the WordPress system. It’s important because it provides a consistent way to handle errors and can help developers debug issues more effectively.
How do I use the WP_Error class in my code?
To use the WP_Error class, you first need to instantiate it. You can do this by creating a new instance of the class and passing in an error code and message. For example: $error = new WP_Error( 'broke', 'I've fallen and can't get up' );
. You can then check for errors using the is_wp_error()
function.
How can I add additional error messages to an existing WP_Error instance?
You can add additional error messages to an existing WP_Error instance using the add()
method. This method takes two parameters: an error code and an error message. For example: $error->add( 'broke', 'I've fallen and can't get up' );
.
How can I retrieve error messages from a WP_Error instance?
You can retrieve error messages from a WP_Error instance using the get_error_message()
method. This method takes one parameter: an error code. It returns the corresponding error message. For example: echo $error->get_error_message( 'broke' );
.
Can I retrieve all error codes from a WP_Error instance?
Yes, you can retrieve all error codes from a WP_Error instance using the get_error_codes()
method. This method returns an array of all error codes in the instance. For example: print_r( $error->get_error_codes() );
.
Can I retrieve all error messages from a WP_Error instance?
Yes, you can retrieve all error messages from a WP_Error instance using the get_error_messages()
method. This method returns an array of all error messages in the instance. For example: print_r( $error->get_error_messages() );
.
How can I remove error messages from a WP_Error instance?
You can remove error messages from a WP_Error instance using the remove()
method. This method takes one parameter: an error code. It removes all error messages associated with that code. For example: $error->remove( 'broke' );
.
Can I check if a specific error code exists in a WP_Error instance?
Yes, you can check if a specific error code exists in a WP_Error instance using the has_errors()
method. This method takes one parameter: an error code. It returns true if the code exists, and false otherwise. For example: if ( $error->has_errors( 'broke' ) ) { /* do something */ }
.
Can I add data to an error in a WP_Error instance?
Yes, you can add data to an error in a WP_Error instance using the add_data()
method. This method takes two parameters: the data to add, and the error code to add it to. For example: $error->add_data( 'some data', 'broke' );
.
How can I retrieve data from an error in a WP_Error instance?
You can retrieve data from an error in a WP_Error instance using the get_error_data()
method. This method takes one parameter: an error code. It returns the data associated with that code. For example: echo $error->get_error_data( 'broke' );
.

Firdaus Zahari is a web developer who comes all the way from Malaysia. His passion revolves around (but is not limited to) WordPress and front-end development.