- 1. Using jQuery for AJAX in ASP.NET
- 2. Using jQuery Grid with ASP.NET MVC
- 3. Using jQuery to directly call ASP.NET AJAX page methods
- 4. Use jQuery and ASP.NET to Build a News Ticker
- 5. jQuery & asp.net: How to implement paging,sorting and filtering on a client side grid using asp.net,jTemplates and JSON
- 6. jQuery MicroAjax for ASP.NET
- 7. ASP.Net default button fix
- 8. Ajax Dot Net
- 9. Using jQuery for ASP.NET Textbox Autocomplete
- 10. Check username availability with JQuery and ASP.NET
- Frequently Asked Questions (FAQs) about jQuery in ASP.NET
10 awesome ASP.NET jQuery plugins which can help do things like AJAX requests, grids, json manage, panels and more. Learn how to integrate ASP.NET using jQuery and Ajax. Check out our featured tutorials below.
1. Using jQuery for AJAX in ASP.NET
This article will demonstrate how to use a popular JavaScript library (jQuery) to add AJAX capabilities to your application with a minimal amount of code.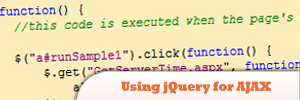
2. Using jQuery Grid with ASP.NET MVC
A common scenario when building web user interfaces is providing a pageable and sortable grid of data. Even better if it uses AJAX to make it more responsive and snazzy. Since ASP.NET MVC includes jQuery, I figured it’d be fun to use a jQuery plugin for this demo, so I chose jQuery Grid.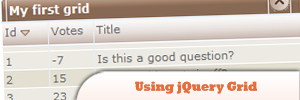
3. Using jQuery to directly call ASP.NET AJAX page methods
In this post, we will tell exactly what is and isn’t necessary in order to use page methods. Then, we’ll show you how to use jQuery to call a page method without using the ScriptManager.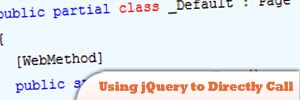
4. Use jQuery and ASP.NET to Build a News Ticker
This article is the first in a series that explores how to create your own news ticker widget using jQuery and ASP.NET. jQuery is a free, popular, open-source JavaScript library that simplifies many common client-side tasks, like event handling, DOM manipulation, and Ajax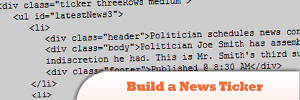
5. jQuery & asp.net: How to implement paging,sorting and filtering on a client side grid using asp.net,jTemplates and JSON
This article will concentrate only on high performance grid implementation using jQuery,JSON, .net,Linq.Most of us in our career face a situation when we want performance intensive i.e solutions where high performance is the topmost priority.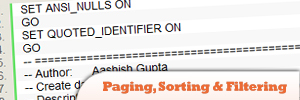
6. jQuery MicroAjax for ASP.NET
MicroAjax is a set of jQuery plugins and .NET components designed to provide simple, powerful and efficient Ajax centric web application design patterns.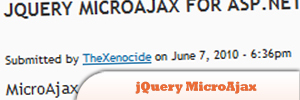
7. ASP.Net default button fix
This small plugin fixes a known issue with the ‘DefaultButton’ property of an ASP.Net Panel when used with a LinkButton. The DefaultButton property doesn’t work correctly in FireFox due to the fact that an ‘A’ doesn’t have an onClick property by default in FF.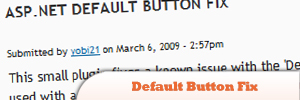
8. Ajax Dot Net
Provides a function which allows GET and POST requests to ASP.NET Ajax enabled PageMethods and Web Services. The function only supports JSON requests and requires JSON2.js from json.org.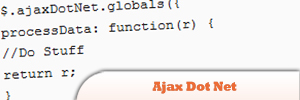
9. Using jQuery for ASP.NET Textbox Autocomplete
Autocomplete an input field to enable users quickly finding and selecting some value, leveraging searching and filtering.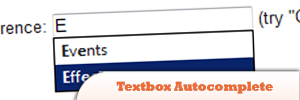
10. Check username availability with JQuery and ASP.NET
A cool use for AJAX is to let users know if a particular username is available or not while they are filling out the registration form.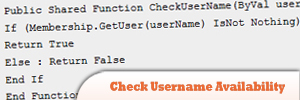
Frequently Asked Questions (FAQs) about jQuery in ASP.NET
How do I include jQuery in my ASP.NET project?
To include jQuery in your ASP.NET project, you need to download the jQuery library from the official jQuery website. Once downloaded, you can include it in your project by adding a script tag in your HTML file. The script tag should point to the location of the jQuery file in your project. For example, if you have placed the jQuery file in a folder named ‘scripts’, the script tag would look like this: <script src="scripts/jquery-3.5.1.min.js"></script>
. This line of code should be placed in the head section of your HTML file.
Can I use jQuery with ASP.NET WebForms?
Yes, you can use jQuery with ASP.NET WebForms. jQuery is a JavaScript library that simplifies HTML document traversing, event handling, and animation. It works perfectly with ASP.NET WebForms, enhancing the client-side functionality of your web application. To use jQuery with ASP.NET WebForms, you need to include the jQuery library in your project and then use jQuery syntax in your script tags.
How can I add jQuery programmatically to a Web Form?
To add jQuery programmatically to a Web Form, you can use the ClientScriptManager
class in ASP.NET. This class provides methods to manage JavaScript and CSS at runtime. Here is an example of how you can use it to add jQuery to your Web Form:if (!Page.ClientScript.IsClientScriptIncludeRegistered("jQuery"))
{
Page.ClientScript.RegisterClientScriptInclude("jQuery", "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js");
}
In this code, the IsClientScriptIncludeRegistered
method checks if jQuery is already registered. If not, the RegisterClientScriptInclude
method is used to register jQuery.
What are some common uses of jQuery in ASP.NET?
jQuery is commonly used in ASP.NET to enhance the user interface and improve the user experience. Some common uses include form validation, AJAX calls, DOM manipulation, and animation effects. jQuery can also be used to handle user events such as clicks, mouse movements, and keyboard input.
How can I use jQuery for AJAX calls in ASP.NET?
jQuery provides several methods for AJAX functionality. The $.ajax()
method is a powerful and flexible method that allows you to make AJAX requests. Here is an example of how you can use it in ASP.NET:$.ajax({
url: 'YourUrl',
type: 'POST',
data: { 'id': id },
success: function (data) {
// Handle the response here
},
error: function (xhr, status, error) {
// Handle the error here
}
});
In this code, the url
parameter is the URL you are making the AJAX request to. The type
parameter is the HTTP method to use for the request, and the data
parameter is the data to send to the server. The success
and error
parameters are callback functions that are executed when the request succeeds or fails, respectively.
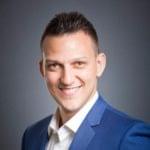
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.