What is .alert()
Sometimes you need to go back to basics and use the .alert() function in jQuery to see what a variable is or check if your JavaScript is actually working at all. Probably one of the most underated functions, but certainly the most used, is jquery.Alert(). Also known as a jQuery command box or system popup window, jQuery alerts contain text information displayed to the user.
How to use .alert()
You can use the following code:
//when the webpage is loaded
jQuery(document).ready(function($) {
alert("js is working");
});
Other variations:
alert($('#test'));
// or
alert($('#test').get(0));
// also try
alert(document.getElementById('test'));
If the last one doesn’t work (it should alert ‘HTMLElement’, depending on the browser) then jQuery is not working, you might need to check your imports are correct or check for errors in your JavaScript files.
Frequently Asked Questions (FAQs) about jQuery alert()
What is alert() in jQuery?
alert() is not specific to jQuery; it’s a JavaScript function that displays a popup dialog box with a message and an OK button. It’s commonly used to show notifications or messages to users in a web application.
How do I use jQuery alerts?
Using alert() is simple. Just call the function and pass a message as an argument. For example:alert("Hello, World!");
This will display an alert box with the message “Hello, World!” and an OK button.
Is alert() a jQuery-specific function?
No, alert() is a native JavaScript function that’s available in all web browsers. It’s not specific to jQuery.
Do I need to include any external libraries to use alert()?
No, you don’t need any external libraries like jQuery to use alert(). It’s a core part of JavaScript and is supported by browsers natively.
Can I customize the appearance of the alert() dialog?
No, the appearance and behavior of the alert() dialog cannot be customized. It’s a system-generated default dialog with a fixed style, font size and behavior.
Are there any alternatives to alert() for displaying messages?
Yes, there are alternatives like confirm() and prompt(). confirm() displays a confirm box with OK and Cancel buttons, while prompt() has an input field lets the user enter text. Additionally, many developers use HTML and CSS to create custom modal dialogs for more control over appearance and behavior.
Can I use alert() for debugging purposes?
Yes, alert() is often used for simple debugging by displaying popup boxes with variable values or checking code flow. However, using popup boxes extensively for debugging can be cumbersome and less effective compared to dedicated browser developer tools.
Are there any drawbacks to using alert()?
While alert() is easy to use, it has limitations. It can disrupt user experience, especially if used excessively. It’s a blocking function, meaning it halts script execution until the user dismisses the dialog. For more user-friendly alternatives, consider using modal dialogs or notifications.
How can I include line breaks in the alert() message?
You can use the escape sequence \n to add line breaks in the alert() message. For example:alert("Line 1\nLine 2");
Can I style the text or buttons inside an alert() dialog?
No, you cannot directly style the text or buttons inside an alert() dialog. If you need customization, you’ll need to explore alternative methods like creating custom modal dialogs using HTML, CSS, and JavaScript.
Can I use jQuery to create custom dialogs with more control?
Yes, jQuery UI provides a dialog widget that allows you to create custom dialogs with various options for styling, buttons, and behavior. This gives you more control over the appearance and interactions compared to the native alert() function.
Is it possible to capture the user’s response to an alert()?
No, alert() doesn’t provide a way to capture user input or responses. It’s a simple notification dialog without any interaction beyond clicking the OK button.
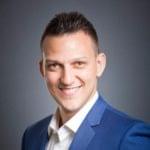
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.