jQuery code snippet to get the dynamic variables stored in the url as parameters and store them as JavaScript variables ready for use with your scripts. Used differently to Hash Url’s as the world turns to dynamic web apps. Thus things like Decoding URL Strings will be ever popular for years to come.
$.urlParam = function(name){
var results = new RegExp('[\?&]' + name + '=([^&#]*)').exec(window.location.href);
return results[1] || 0;
}
// example.com?param1=name¶m2=&id=6
$.urlParam('param1'); // name
$.urlParam('id'); // 6
$.urlParam('param2'); // null
//example params with spaces
http://www.jquery4u.com?city=Gold Coast
console.log($.urlParam('city'));
//output: Gold%20Coast
console.log(decodeURIComponent($.urlParam('city')));
//output: Gold Coast
This could be used for example to set the default value of a text input field:
$('#city').val(decodeURIComponent($.urlParam('city')));
Thanks to bjverde for improvements to this function:
$.urlParam = function(name){
var results = new RegExp('[\?&]' + name + '=([^&#]*)').exec(window.location.href);
if (results==null){
return null;
}
else{
return results[1] || 0;
}
}
Frequently Asked Questions (FAQs) about URL Parameters in jQuery
How can I get URL parameters using jQuery?
To get URL parameters using jQuery, you can use the window.location.search
property. This property returns the query string parameters of a URL. You can then use the substring()
method to remove the question mark at the start of the query string. After that, you can split the parameters by the ampersand character. Here’s a simple example:var params = window.location.search.substring(1).split('&');
Each element in the params
array now represents a URL parameter. You can further split each parameter by the equals sign to separate the parameter name and value.
How can I use the jQuery.param() method?
The jQuery.param()
method is a utility function that creates a serialized representation of an array or an object. This can be useful when you want to create a query string from an array or object. Here’s an example:var params = { name: 'John', age: 30 };
var queryString = $.param(params);
In this example, queryString
will be the string ‘name=John&age=30’.
Can I get URL parameters without using jQuery?
Yes, you can get URL parameters without using jQuery by using plain JavaScript. The window.location
object in JavaScript provides properties and methods you can use to work with the current URL. For example, you can use the window.location.search
property to get the query string parameters.
How can I set URL parameters using jQuery?
jQuery does not provide a built-in method to set URL parameters. However, you can use the window.location
object in JavaScript to change the URL. If you want to change the URL without reloading the page, you can use the history.pushState()
method.
How can I remove a specific URL parameter using jQuery?
To remove a specific URL parameter, you can first get all the parameters using the window.location.search
property. Then, you can create a new query string without the parameter you want to remove. After that, you can set the new query string using the history.pushState()
method.
How can I check if a URL parameter exists using jQuery?
You can check if a URL parameter exists by getting all the parameters using the window.location.search
property. Then, you can split the parameters and check if the parameter you are looking for is in the array.
Can I use jQuery to work with hash parameters in the URL?
Yes, you can use the window.location.hash
property to work with hash parameters in the URL. This property returns the part of the URL that follows the hash sign.
How can I get multiple URL parameters using jQuery?
To get multiple URL parameters, you can use the window.location.search
property and split the parameters by the ampersand character. Each element in the resulting array represents a URL parameter.
Can I use jQuery to change the URL without reloading the page?
Yes, you can use the history.pushState()
method in JavaScript to change the URL without reloading the page. This method adds a new entry to the browser’s history.
How can I handle URL parameters in AJAX requests using jQuery?
When making AJAX requests using jQuery, you can include URL parameters in the URL string. Alternatively, you can use the data
option in the $.ajax()
method to include parameters. This option automatically converts an object or array to a query string.
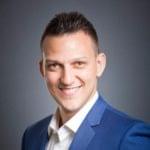
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.