Ok so for some reason you want to use JavaScript to redirect a web page. You can use either jQuery or plain JavaScript to do the job. Probably the latter if it’s an instant redirect required.
Redirect Using plain JavaScript
jQuery is not necessary, and window.location.replace(…) will best simulate an HTTP redirect.// simulates similar behavior as an HTTP redirect
window.location.replace("http://jquery4u.com");
// simulates similar behavior as clicking on a link
window.location.href = "http://jquery4u.com";
Redirect Using jQuery
var url = "http://jquery4u.com";
$(location).attr('href',url);
It is better than using window.location.href =, because replace() does not put the originating page in the session history, meaning the user won’t get stuck in a never-ending back-button fiasco. If you want to simulate someone clicking on a link, use location.href (works for every browser). If you want to simulate an HTTP redirect, use location.replace.
Frequently Asked Questions (FAQs) about jQuery Redirect
What is jQuery redirect and why is it used?
jQuery redirect is a method used in web development to navigate from one webpage to another. It is a part of jQuery, a fast, small, and feature-rich JavaScript library. The redirect function is used when you want to redirect the user to a different page or website URL. This can be useful in a variety of scenarios, such as after a user has submitted a form and you want to redirect them to a thank you page, or if a page has moved and you want to send users to the new location.
How can I implement a jQuery redirect?
Implementing a jQuery redirect is quite straightforward. You need to use the window.location object in JavaScript. Here’s a simple example:$(document).ready(function(){
window.location.href = "https://www.newurl.com";
});
In this example, when the document is ready, the browser will redirect to the new URL.
Can I redirect to a new window using jQuery?
Yes, you can redirect to a new window using jQuery. Instead of using window.location.href, you would use window.open(). Here’s an example:$(document).ready(function(){
window.open("https://www.newurl.com", "_blank");
});
In this example, the “_blank” parameter causes the new URL to open in a new window or tab.
Can I delay a jQuery redirect?
Yes, you can delay a jQuery redirect using the setTimeout() function in JavaScript. Here’s an example:$(document).ready(function(){
setTimeout(function(){
window.location.href = "https://www.newurl.com";
}, 5000);
});
In this example, the redirect will occur after a delay of 5000 milliseconds, or 5 seconds.
Can I use jQuery to redirect based on certain conditions?
Yes, you can use jQuery to redirect based on certain conditions. For example, you might want to redirect users based on the form input. Here’s an example:$(document).ready(function(){
if($("#inputField").val() == "specificValue"){
window.location.href = "https://www.newurl.com";
}
});
In this example, if the value of the input field with the id “inputField” is “specificValue”, the user will be redirected to the new URL.
Can I use jQuery to redirect to a relative URL?
Yes, you can use jQuery to redirect to a relative URL. Instead of providing the full URL, you would provide the path relative to the current page. Here’s an example:$(document).ready(function(){
window.location.href = "/newpage.html";
});
In this example, the user will be redirected to “newpage.html” in the same domain as the current page.
Can I use jQuery to redirect to an external URL?
Yes, you can use jQuery to redirect to an external URL. You would provide the full URL as the parameter to window.location.href. Here’s an example:$(document).ready(function(){
window.location.href = "https://www.externalurl.com";
});
In this example, the user will be redirected to the external URL.
Can I use jQuery to redirect after a button click?
Yes, you can use jQuery to redirect after a button click. You would use the click event handler in jQuery. Here’s an example:$(document).ready(function(){
$("#myButton").click(function(){
window.location.href = "https://www.newurl.com";
});
});
In this example, when the button with the id “myButton” is clicked, the user will be redirected to the new URL.
Can I use jQuery to redirect after a form submission?
Yes, you can use jQuery to redirect after a form submission. You would use the submit event handler in jQuery. Here’s an example:$(document).ready(function(){
$("#myForm").submit(function(){
window.location.href = "https://www.newurl.com";
});
});
In this example, when the form with the id “myForm” is submitted, the user will be redirected to the new URL.
Can I use jQuery to redirect with a fade out effect?
Yes, you can use jQuery to redirect with a fade out effect. You would use the fadeOut() function in jQuery. Here’s an example:$(document).ready(function(){
$("body").fadeOut(2000, function(){
window.location.href = "https://www.newurl.com";
});
});
In this example, the body of the current page will fade out over 2 seconds, after which the user will be redirected to the new URL.
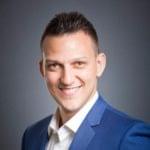
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.