Sometimes you just need a simple way of reading XML into your JavaScript code and creating variables from the XML items. Fortunately, jQuery can read xml easy! :) There are many plugins and code snippets available and here are the pick of the bunch! In this post we cover following to guide you through your XML reading options:
- JavaScript XML Parsing
- jQuery XML Parsing
- Using jQuery.get() to get data from xml
- jQuery XML Plugin
- Tips on Parsing XML
- What JS functions generally don’t work well
Regular JavaScript XML Parsing
var xmlDoc = request.responseXML;
try // Build Markers, if available
{
var markers = xmlDoc.getElementsByTagName("marker") ;
for ( var i = 0; i < markers.length ; i++ ) {
var point = {
markers[i].getAttribute("lat")),
markers[i].getAttribute("lng")
};
}
} catch(e) {}
jQuery XML Parsing
$(request.responseXML).find("marker").each(function() {
var marker = $(this);
var point = {
marker.attr("lat"),
marker.attr("lng")
};
});
$(request.responseXML).find("marker").each(function() {
var marker = $(this);
var point = {
marker.attr("lat"),
marker.attr("lng")
};
});
Using jQuery.get() to get data from xml
$('Contact',xml).each(function() {
srno = parseInt($(this).find("srno").text());
empId = $(this).find("empid").text();
name = $(this).find("name").text();
contact = $(this).find("contact-data").text();
type = $(this).find("type").text();
}
jParse jQuery XML Plugin
“jParse is a jQuery plugin that allows you to parse XML that was fetched with the jQuery .ajax method (making it fully customizable). It’s compatible with jQuery 1.4+, easy to use and ultra lightweight at only 4KB! Best of all, it’s compatible with all major browsers:” Demo Download SourceTips on Parsing XML
- Try to limit your xml file size to less than 5mb to avoid the system slow up (if your file is too big you could try taking the xml data and turning it into a JSON object. There are lots of ways to do this, including a jQuery plugin)
- If you are unfamiliar with XML synatax, check out the W3School’s rules on XML syntax.
- Put your code inside the $(document).ready(function(){ //here }
- You may find that some code works fine in FF and but does not work in IE (it seems IE has issues with the way jQuery handles XML on the local filesystem. If you upload the same code on a server it works without a problem)
- If you are pulling data for dynamic pages then try to use relative path over absolute path
What jQuery XML commands commonly don’t work
var response = xmlHttp.responseText;
var sms = $(response).find('node').text();
var sms = $.parseXML(response).find('node').text();
Further readings: http://think2loud.com/reading-xml-with-jquery/
Frequently Asked Questions about jQuery XML Plugin
How can I use jQuery to parse XML data?
Parsing XML data using jQuery is a straightforward process. First, you need to use the $.ajax() method to load the XML file. Inside the success callback function, you can use the find() method to find specific XML elements. You can then extract the data from these elements using the text() method. Remember to include error handling in your code to manage any issues that might arise while loading or parsing the XML file.
What is the purpose of the jQuery.parseXML() method?
The jQuery.parseXML() method is used to parse a string into an XML document, which can then be manipulated using jQuery. This method is particularly useful when you need to retrieve XML data from a server and then work with it in your JavaScript code. It creates a native XMLDocument object that can be traversed and manipulated using jQuery methods.
How can I handle errors when parsing XML with jQuery?
Error handling is an important aspect of parsing XML with jQuery. You can handle errors by including an error callback function in your $.ajax() method. This function will be called if there’s an error while loading the XML file. The function receives three parameters: jqXHR (a jQuery XMLHttpRequest object), textStatus (a string describing the type of error), and errorThrown (an optional exception object).
Can I use jQuery to parse XML from a remote server?
Yes, you can use jQuery to parse XML data from a remote server. However, due to the same-origin policy, your web page can only load XML data from the same domain that it was served from. If you need to load XML data from a different domain, you can use a technique called JSONP or a server-side proxy.
How can I extract attribute values from XML elements using jQuery?
You can extract attribute values from XML elements using the attr() method in jQuery. After parsing the XML document, you can use the find() method to locate the specific element, and then use the attr() method to retrieve the value of the attribute.
Can I use jQuery to modify XML documents?
While jQuery is primarily used to parse and traverse XML documents, it can also be used to modify them. However, these modifications will only affect the XML document in memory and will not be saved back to the original XML file.
How can I convert an XML document to a string using jQuery?
jQuery does not provide a built-in method to convert an XML document to a string. However, you can use the XMLSerializer object in JavaScript to achieve this. The XMLSerializer.serializeToString() method can be used to convert an XML document into a string.
Can I use jQuery to parse XML namespaces?
jQuery’s built-in XML parsing methods do not support XML namespaces. However, you can use the “namespace-aware” methods provided by the native XML DOM to work with XML namespaces.
How can I use jQuery to parse CDATA sections in XML?
CDATA sections in XML are used to include text that should not be parsed by the XML parser. jQuery treats CDATA sections as text nodes, and you can extract the text inside a CDATA section using the text() method.
Can I use jQuery to parse XML in Internet Explorer?
Yes, you can use jQuery to parse XML in Internet Explorer. However, older versions of Internet Explorer use a different method to parse XML than other browsers. You may need to include additional code to ensure that your XML parsing works correctly in all browsers.
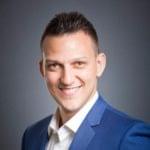
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.