JQuery code snippet to read a text file via the built in AJAX jQuery.get() call and then process the txt file line by line. The example adds the lines to a html element for display on page. Important: The jQuery code will only replace the first occurrence of the word (jQuery4u) and not every occurrence like PHP’s str_replace() would. To replace every occurrence of a string in JavaScript, you must provide the replace() method a regular expression with a global modifier as the first parameter as such:
.replace(/jQuery4u/g,'jQuery4u FTW!');
jQuery Code to read text file line by line
jQuery.get('file.txt', function(data) {
alert(data);
//process text file line by line
$('#div').html(data.replace('n',''));
});
Note: Browsers restrict access to local drives (and to server drives) for security reasons. However, you can use the standard jQuery ajax call $.ajax().
Frequently Asked Questions (FAQs) about Reading Text Files with jQuery
How can I read a local text file using jQuery?
To read a local text file using jQuery, you can use the jQuery.get() method. This method loads data from the server using an HTTP GET request. Here’s a simple example:$.get("file.txt", function(data) {
alert("Data Loaded: " + data);
});
In this example, “file.txt” is the local text file you want to read. The function(data) is a callback function that is executed if the request succeeds. The data parameter contains the data returned from the server – in this case, the contents of the text file.
Can I read a text file from a different domain using jQuery?
Due to security restrictions known as the same-origin policy, you cannot read a text file from a different domain using jQuery’s AJAX methods. The same-origin policy restricts how a document or script loaded from one origin can interact with a resource from another origin. However, there are ways to circumvent this, such as using a server-side proxy or JSONP, but these methods have their own limitations and security concerns.
How can I handle errors when reading a text file with jQuery?
You can handle errors when reading a text file with jQuery by using the .fail() method. This method is called when the request fails. Here’s an example:$.get("file.txt")
.done(function(data) {
alert("Data Loaded: " + data);
})
.fail(function() {
alert("Error occurred");
});
In this example, if the request to load “file.txt” fails, the .fail() method is called, and an error message is displayed.
Can I read a text file line by line using jQuery?
jQuery does not provide a built-in method to read a text file line by line. However, you can achieve this by splitting the file content by newline characters. Here’s an example:$.get("file.txt", function(data) {
var lines = data.split("\n");
$.each(lines, function(n, elem) {
console.log("Line " + (n+1) + ": " + elem);
});
});
In this example, the file content is split by newline characters (“\n”) into an array of lines. Then, each line is logged to the console.
How can I read a CSV file using jQuery?
You can read a CSV file using jQuery in a similar way to reading a text file. However, you need to parse the file content into a usable format. Here’s an example:$.get("file.csv", function(data) {
var lines = data.split("\n");
$.each(lines, function(n, elem) {
var fields = elem.split(",");
console.log("Fields: " + fields);
});
});
In this example, each line of the file is split by comma characters (“,”) into an array of fields. Then, each array of fields is logged to the console.
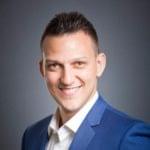
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.