If you have more than 1 field which you want to validate as a group and not display 3 separate validation messages (such as date of birth, address etc…) this is how you can do it! Group your validation rules into 1 Validation Message, great to know!
$("form").validate({
rules: {
DayOfBirth: { required: true },
MonthOfBirth: { required: true },
YearOfBirth: { required: true }
},
groups: {
DateofBirth: "DayOfBirth MonthOfBirth YearOfBirth"
},
errorPlacement: function(error, element) {
if (element.attr("name") == "DayOfBirth" || element.attr("name") == "MonthOfBirth" || element.attr("name") == "YearOfBirth")
error.insertAfter("#YearOfBirth");
else
error.insertAfter(element);
}});
Frequently Asked Questions about jQuery Group Rules for Form Validation
How can I use jQuery to validate multiple fields in a form?
jQuery provides a powerful validation plugin that you can use to validate multiple fields in a form. To do this, you need to group the fields together using the ‘groups’ option in the validate method. This option takes an object where the key is the name of the group and the value is a string of space-separated field names. Once grouped, you can apply validation rules to the group as a whole, ensuring that all fields in the group meet the specified criteria.
What is the purpose of the ‘require_from_group’ method in jQuery validation?
The ‘require_from_group’ method is a special validation rule in jQuery that requires a certain number of fields in a group to be filled out by the user. This is useful in situations where you want to ensure that at least one field in a group of related fields is filled out. For example, you might have a form where the user can enter either a phone number or an email address, but at least one of these fields must be filled out.
How can I customize the error messages in jQuery validation?
jQuery validation plugin allows you to customize the error messages that are displayed when a validation rule is not met. You can do this by using the ‘messages’ option in the validate method. This option takes an object where the key is the field name and the value is another object containing the validation rules and their corresponding custom error messages.
Can I use jQuery validation plugin to validate dynamic form fields?
Yes, you can use jQuery validation plugin to validate dynamic form fields. The plugin provides a ‘rules’ method that you can use to add or remove validation rules for a specific field at runtime. This is particularly useful when you have a form that changes based on user input, and you need to adjust the validation rules accordingly.
How can I trigger validation manually using jQuery validation plugin?
jQuery validation plugin provides a ‘valid’ method that you can use to manually trigger validation for a form or a specific field. When called, this method checks whether the form or field is valid according to the specified validation rules and returns a boolean value. You can use this method in combination with event handlers to trigger validation at specific points in your code.
What is the difference between ‘validate’ and ‘valid’ methods in jQuery validation plugin?
The ‘validate’ method in jQuery validation plugin is used to initialize the validation functionality for a form, while the ‘valid’ method is used to check whether a form or a specific field is valid according to the specified validation rules. The ‘validate’ method should be called once when the form is loaded, while the ‘valid’ method can be called multiple times whenever you need to check the validity of the form or field.
Can I use jQuery validation plugin with AJAX forms?
Yes, you can use jQuery validation plugin with AJAX forms. The plugin provides a ‘submitHandler’ option that you can use to specify a function to be executed when the form is valid and ready to be submitted. This function can contain your AJAX code to submit the form data to the server without refreshing the page.
How can I use jQuery validation plugin to validate a checkbox group?
To validate a checkbox group using jQuery validation plugin, you need to group the checkboxes together using the ‘groups’ option in the validate method, and then apply the ‘required’ rule to the group. This will ensure that at least one checkbox in the group is checked by the user.
Can I use jQuery validation plugin to validate file inputs?
Yes, you can use jQuery validation plugin to validate file inputs. The plugin provides several validation rules for file inputs, such as ‘accept’ to specify the allowed file types, ‘maxfilesize’ to specify the maximum file size, and ‘minfilesize’ to specify the minimum file size.
How can I disable validation for a specific field using jQuery validation plugin?
To disable validation for a specific field using jQuery validation plugin, you can use the ‘ignore’ option in the validate method. This option takes a string or array of field names to be ignored by the validation. Once ignored, the field will not be validated and no error messages will be displayed for it.
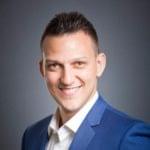
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.