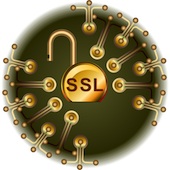
Typical Browser-Website SSL Flow.
Certificate Authority: Verisign, Godaddy, DigiCert, etc Client: Browser Service: Website- The Certificate Authority (CA) creates a certificate. This is THEIR certificate that is used to prove the identity of the CA.
- The Service creates a CSR (Certificate Signing Request). The CSR will have details about the identity of the website and company.
- The Service will then submit this CSR to the CA to get validated.
- The CA will validate the details in the CSR.
- The CA uses their certificate to digitally sign the CSR (Certificate Signing Request). This creates an SSL Certificate which is then issued by the service.
Setup SSL Authentication
First thing we need to do is create a Certificate Authority (We are our own Certificate Authority now). This will be used to sign the Client CSR. Since we are reversing everything, the client issues the CSR instead of the Service. Here’s how to generate a private key and a certificate request, followed by self-signing the certificate.openssl genrsa -out ca.key 1024
openssl req -new -key ca.key -out ca.csr
openssl x509 -req -days 365 -in ca.csr -signkey ca.key -out ca.crt
You will then need to copy the ca.crt file to your Service. This is our list of trusted CAs, consisting of just us.
If using apache you will need to set options in httpd.conf file:
...
SSLCACertificateFile /path/to/cacert/ca.crt
SSLVerifyClient require
...
Check out apache’s site for more information on other options.
For NGINX, add the following to the nginx.conf file:
http {
server {
……...
ssl_client_certificate /path/to/cacert/ca.crt;
ssl_verify_client on;
……..
}
}
Check out nginx’s site for more options.
Each Client will need its own SSL Certificate. The Client will create a CSR and send a request to the Certificate Authority (which is us).
Generate the private key (in this case client.key) and a certificate request for the Client
openssl genrsa -out client.key 1024
openssl req -new -key client.key -out client.csr
Then sign the request using the Certificate Authority certificate and key we created (ca.crt, ca.key) and return the signed certificate.
openssl x509 -req -days 365 -CA ca.crt -CAkey ca.key -CAcreateserial -in client.csr -out client.crt
Now when the client makes a request to the service it should pass the client.crt
Going back to our first example. I’m going to make a request to add an event to the schedule from the mail app. I am going to use Faraday because it is awesome.
request = Faraday::Connection.new https://schedule.com, :ssl => {
:verify => false,
:client_cert => OpenSSL::X509::Certificate.new(File.read(/path/to/mail_client.crt)),
:client_key => OpenSSL::PKey::RSA.new(File.read(/path/to/mail_client.key)),
}
request.headers['Accept'] = 'application/json'
request.headers['Content-Type'] = 'application/json'
response = request.post "/events", {event: {start_time:"2013-08-01T16:20:00+00:00", end_time:"2013-08-01T18:20:00+00:00", name:"Meeting"}}.to_json
:verify
in this case means that the Client is verifying that the Service is who they say they are (Typical Browser-Website Flow). If set to true
you need to add the :ca_file => 'path/to/cafile'
option as well. The ca_file
in this case is the CA file used to create your domain’s (meaning, the Service) SSL certificate. If you bought your SSL Certificate from Godaddy you would need to download the CA Certificate they used to create your SSL Certificate. (They have a list here ).
Why this approach then instead of basic auth or oauth? The biggest thing is that the service doesn’t have to do anything when adding more clients. A new client would simply create a CSR and request an SSL Certificate from the same Certificate Authority.
Originally got the idea from this
Collective Idea article.
We are pretty early in our approach, but so far, it looks very promising.
Frequently Asked Questions (FAQs) on Inter-Service Communication Using Client Certificate Authentication
What is the role of client certificate authentication in inter-service communication?
Client certificate authentication plays a crucial role in inter-service communication, especially in a microservices architecture. It provides a secure method of verifying the identity of clients. In this process, a client presents a digital certificate, which the server verifies before granting access. This method is highly secure as it uses cryptographic techniques, making it difficult for unauthorized entities to gain access. It’s particularly useful in microservices where multiple services need to communicate securely.
How does client certificate authentication enhance the security of microservices?
Client certificate authentication enhances the security of microservices by ensuring that only authorized services can communicate with each other. It uses digital certificates, which are hard to forge, thus reducing the risk of unauthorized access. This is particularly important in a microservices architecture where services often need to communicate over unsecured networks. By verifying the identity of the communicating services, client certificate authentication helps prevent data breaches and other security incidents.
What are the steps involved in setting up client certificate authentication?
Setting up client certificate authentication involves several steps. First, you need to generate a Certificate Authority (CA) and use it to issue certificates for your services. Next, you configure your services to present these certificates when making requests. Finally, you configure your services to verify the certificates presented by other services. This involves setting up a trust store that contains the CA certificate and configuring your services to reject any certificate not issued by the CA.
How does inter-service communication work in a microservices architecture?
In a microservices architecture, services communicate with each other to perform complex tasks. This communication can take various forms, including HTTP/REST, gRPC, or messaging queues. Regardless of the method used, it’s crucial to secure this communication to prevent unauthorized access. One way to do this is through client certificate authentication, which verifies the identity of the communicating services.
What are the challenges of inter-service communication in microservices?
Inter-service communication in microservices presents several challenges. One of the main challenges is ensuring secure communication. With multiple services communicating over potentially unsecured networks, there’s a risk of data breaches. Another challenge is managing the complexity of communication. As the number of services increases, so does the complexity of their interactions. This can make it difficult to understand and manage the system as a whole.
How can I troubleshoot issues with client certificate authentication?
Troubleshooting issues with client certificate authentication can involve several steps. First, check that your certificates are correctly configured and not expired. Next, ensure that your services are correctly presenting their certificates when making requests. Finally, check that your services are correctly verifying the certificates presented by other services. If you’re still experiencing issues, it may be helpful to use a tool like Wireshark to inspect the network traffic and identify any anomalies.
Can client certificate authentication be used with other security measures?
Yes, client certificate authentication can be used in conjunction with other security measures to provide layered security. For example, you could use it alongside transport layer security (TLS) to encrypt the communication between services. You could also use it with an API gateway to provide additional access control.
What are the alternatives to client certificate authentication for securing inter-service communication?
There are several alternatives to client certificate authentication for securing inter-service communication. These include using an API gateway to control access, using OAuth or JWT for authentication, or using a service mesh like Istio to manage and secure communication. The best choice depends on your specific needs and the nature of your microservices architecture.
How does client certificate authentication compare to other authentication methods?
Client certificate authentication offers several advantages over other authentication methods. It’s highly secure, as it uses cryptographic techniques that are hard to forge. It’s also scalable, as you can easily issue new certificates as you add more services. However, it can be more complex to set up and manage than other methods, and it requires careful management of certificates to avoid security issues.
What are the best practices for managing certificates in a microservices architecture?
Managing certificates in a microservices architecture involves several best practices. First, ensure that your certificates are securely stored and only accessible to authorized services. Second, regularly rotate your certificates to reduce the risk of them being compromised. Finally, monitor your certificates for any signs of unusual activity, and have a plan in place for responding to potential security incidents.