Creating an Account
To get started, all you need to do is sign up for an account. This takes seconds to do, and will give access to to the app console.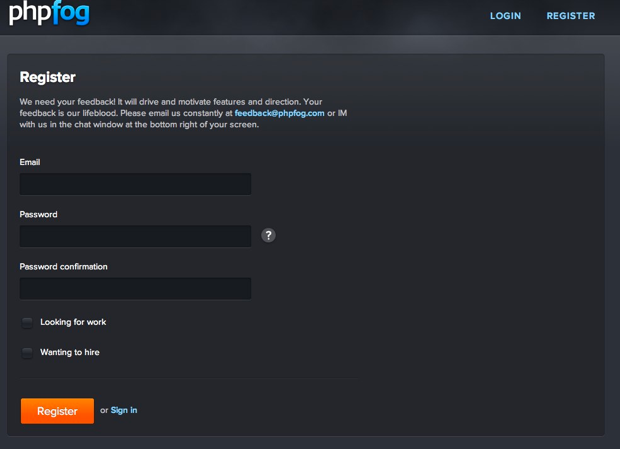
Create an Application
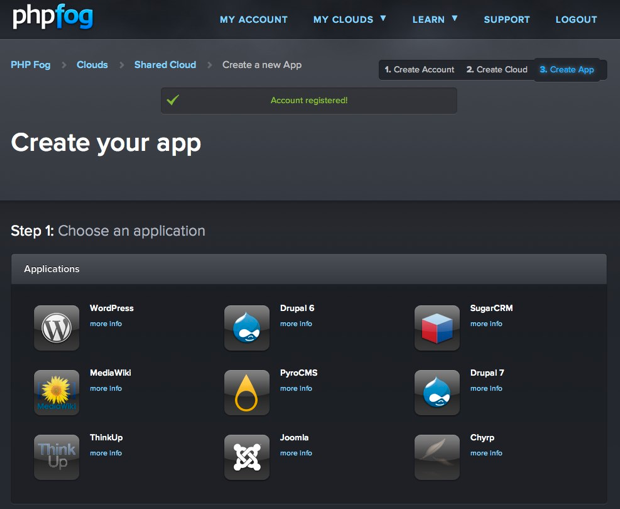
Create an App with a Framework Installation
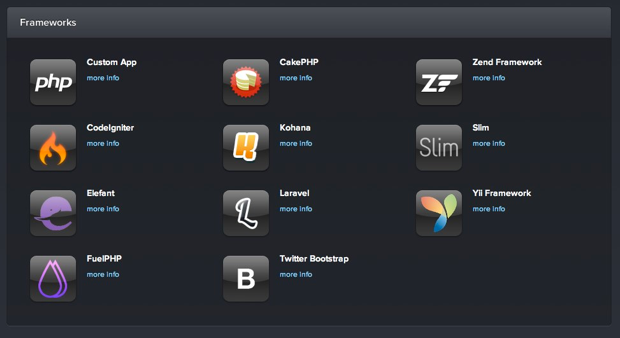
Create an App
For this article, I am going to guide you though how to create a skeleton app using the CodeIgniter framework. So in the lower half of the window, click the CodeIgniter icon: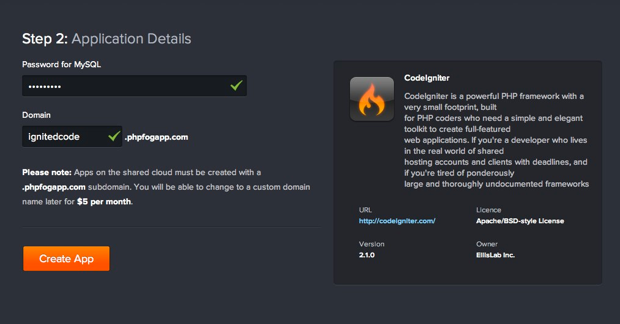
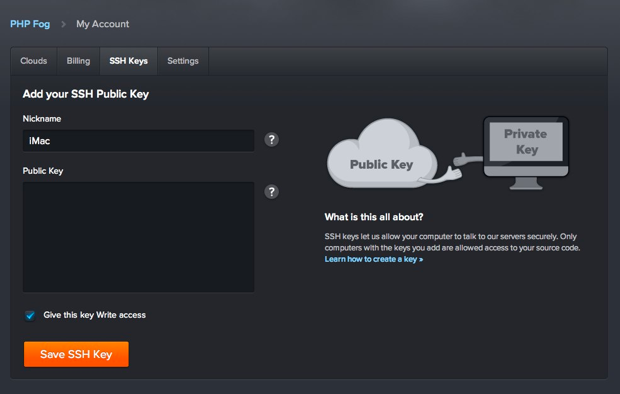
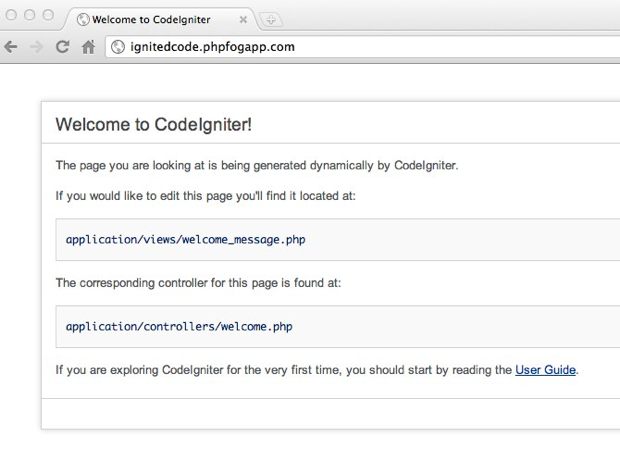
Create an SSH Key
It might be that you have created a public/private key pair on your computer already. But if you haven’t you will need to create one. PHPFog have a very useful guide that explains how to do that. It covers Mac and Windows; the procedure for Linux is much the same as for a Mac. You need the ssh keys, so that the PHPFog server can communicate securely with your computer. From the account window, click on SSH Keys. Here, you can add the public part of the key you just created: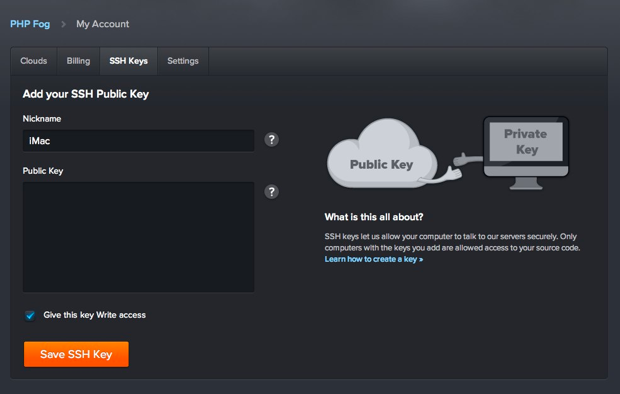
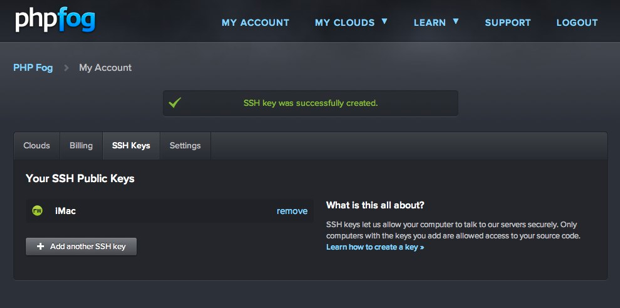
Cloning the App to your Computer
Now the fun really starts. I’m going to assume that you already have Git installed on your computer. If you don’t have it you can find it here. You can use Git to clone the repository PHPFog created for you. If you look at the top right of the app console window, you will see the Git clone url that you need to use: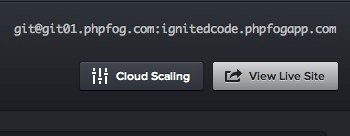
git clone git@git01.phpfog.com:your-domain.phpfogapp.com your-project-name
You will see Git download the code from your PHPFog app into the directory you named at the end of the clone command. Now you can open the code as a project in your favourite IDE/text editor. Next we’ll make some adjustments to the configuration of the framework, and push the changes back to the PHPFog repository.
Working with the Source Code
The first thing we will do, so that we can use pretty url’s in our CodeIgniter web app, is add a .htaccess file. That will serve as a good quick test of pushing code back to the PHPFog repository too. Here is a .htaccess file you can use with CodeIgniter:<IfModule mod_rewrite.c>
RewriteEngine On
RewriteBase /
RewriteCond %{REQUEST_URI} ^system.*
RewriteRule ^(.*)$ /index.php?/$1 [L]
#Checks to see if the user is attempting to access a valid file,
#such as an image or css document, if this isn't true it sends the
#request to index.php
RewriteCond %{REQUEST_FILENAME} !-f
RewriteCond %{REQUEST_FILENAME} !-d
RewriteRule ^(.*)$ /index.php?/$1 [L]
</IfModule>
<IfModule !mod_rewrite.c>
# If we don't have mod_rewrite installed, all 404's
# can be sent to index.php, and everything works as normal.
# Submitted by: ElliotHaughin
ErrorDocument 404 /index.php
</IfModule>
Add this to a .htaccess file in the root directory of the project. Now, back in your terminal window, you can run:
git status
And the reply will show you the newly added file. Do a:
git add .
to stage the file, and then commit the changes:
git commit -am 'add a .htaccess file for pretty url's'
Finally, to send this file to the server, you can push:
git push origin master
Updating the Framework Config
You have just updated the source code on the PHPFog server in the last step. You can’t really see this change yet though. Also, although you have the database configuration information, you still need to add it to the framework so it knows how to talk the the MySQL database.Update the Database Config
Open the file application/config/database.php and add the database configuration options PHPFog created for you:$db['default']['hostname'] = 'mysql-shared-02.phpfog.com';
$db['default']['username'] = 'your username';
$db['default']['password'] = 'your password';
$db['default']['database'] = 'your database name';
$db['default']['dbdriver'] = 'mysql';
Commit your changes, and push up to the PHPFog server again. Refresh the site in your browser, and the CodeIgniter default page should appear. You might think nothing has happened, but if the refresh worked without error, in means CodeIgniter is now connecting to your database.
Update the Site Config
Next, we will add the correct site url and remove the index.php file from our url’s since we are using a .htaccess file. Open application/config/config.php:$config['base_url'] = 'http://your-name-here.phpfogapp.com/';
$config['index_page'] = '';
While we are at it, we will autoload a couple of resources that will be useful. Open application/config/autoload.php, and update the libraries and helpers sections:
$autoload['libraries'] = array('database');
$autoload['helper'] = array('url');
Commit and push your changes.
Proving Things Work
We are now ready to do some coding that will actually affect the web site in a way that we can see. Open application/views/welcome_message.php and copy the contents into a new file called page2.php in the views folder. Then open application/controllers/welcome.php, and create a new method:public function page2()
{
$this->load->view('page2');
}
Then, you can make a minor change to application/views/welcome_message.php:
<div id="body">
<p><?=anchor(base_url() . 'welcome/page2', 'Go to page 2')?></p>
We are using CodeIgniters’ url helper here. You can do something similar in application/views/page2.php:
<div id="body">
<p><?=anchor(base_url() . 'welcome', 'Go to the home page')?></p>
Commit and push your changes, then try it out in your browser:
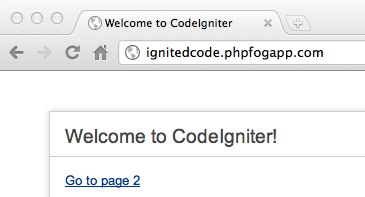
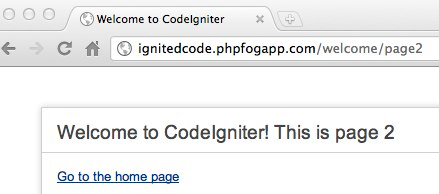
Testing the database
I created a simple table called ‘notes’ via the phpMyAdmin installation provided by PHPFog. I added the following fields: id, title, note. Then I created a couple of test notes. Back in your editor, create a new file in application/models called ‘notes_model.php’. Then add a method for retrieving all the notes:<?php
class Notes_model extends CI_Model
{
public function __construct()
{
parent:: __construct();
}
public function get_notes()
{
$data = array();
$sql = "SELECT * FROM notes";
$q = $this->db->query($sql);
if($q->num_rows() > 0)
{
foreach($q->result() as $row)
{
$data[] = $row;
}
return $data;
}
else
{
return 0;
}
}
}
Now we need to hook up the model to a method in the controller (application/controllers/welcome.php):
public function page2()
{
$this->load->model('notes_model');
$data['notes'] = $this->notes_model->get_notes();
$this->load->view('page2',$data);
}
Here, you can see that we are loading our notes model, calling the ‘get_notes()’ method, and assigning the results to an array ($data) that can be passed to the view.
In application/views/page2.php add the following code:
<div id="body">
<p><?=anchor(base_url() . 'welcome', 'Go to the home page')?></p>
<?php if($notes > 0):?>
<?php foreach($notes as $n):?>
<p>
<?=$n->title?><br />
<?=$n->note?>
</p>
<?php endforeach;?>
<?php else:?>
No notes found.
<?php endif?>
Add, commit, and push your changes, then check the results in your browser:
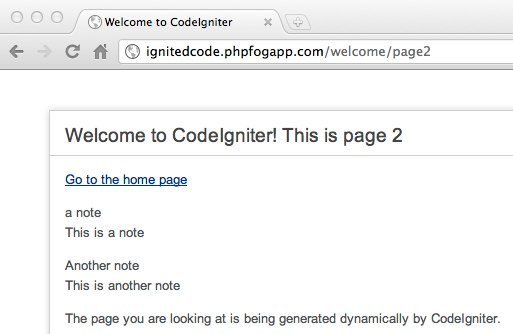
Conclusion
PHPFog makes it really easy to set up and deploy PHP web apps to the cloud. You don’t have to worry about the server set up, or databases, or any updating. It is all done for you. Coding on the cloud also makes it possible to streamline your own development tool chain too, since all you really need, is a text editor and Git. No more FTP pain! Ignition of match with smoke via ShutterstockFrequently Asked Questions about Building a CodeIgniter Web App on PHPFog
What is PHPFog and how does it relate to CodeIgniter?
PHPFog is a cloud-based hosting platform specifically designed for PHP applications. It provides a robust and scalable environment for running PHP applications, including those built with CodeIgniter, a powerful PHP framework. PHPFog simplifies the deployment and management of PHP applications, allowing developers to focus on coding rather than server management.
How do I start building a CodeIgniter web app on PHPFog?
To start building a CodeIgniter web app on PHPFog, you first need to create an account on PHPFog. Once your account is set up, you can create a new app and choose CodeIgniter as your framework. From there, you can start building your app using CodeIgniter’s MVC architecture and PHPFog’s cloud-based hosting environment.
What are the benefits of using PHPFog for hosting my CodeIgniter web app?
PHPFog offers several benefits for hosting CodeIgniter web apps. It provides a scalable and robust hosting environment, automatic backups, and easy deployment of your app. It also offers a dedicated MySQL database for each app, and the ability to easily scale your app to handle increased traffic.
Can I migrate my existing CodeIgniter web app to PHPFog?
Yes, you can migrate your existing CodeIgniter web app to PHPFog. PHPFog provides detailed documentation on how to migrate your app, including how to transfer your database and files.
What happened to PHPFog? Is it still available?
PHPFog was a popular cloud-based hosting platform for PHP applications. However, it has since been discontinued and is no longer available. If you’re looking for a similar service, there are several other cloud-based hosting platforms available that support PHP applications, including Heroku, AWS, and Google Cloud.
What are some alternatives to PHPFog for hosting my CodeIgniter web app?
There are several alternatives to PHPFog for hosting your CodeIgniter web app. These include Heroku, AWS, Google Cloud, and DigitalOcean. Each of these platforms offers robust and scalable hosting environments for PHP applications.
How do I deploy my CodeIgniter web app on PHPFog?
To deploy your CodeIgniter web app on PHPFog, you first need to push your code to a Git repository. Once your code is in the repository, you can use PHPFog’s deployment tools to deploy your app to the cloud.
Can I use PHPFog to host other types of PHP applications?
While PHPFog was specifically designed for PHP applications, it was not limited to any particular framework. This means you could use it to host any type of PHP application, not just those built with CodeIgniter.
How does PHPFog handle database management for my CodeIgniter web app?
PHPFog provided a dedicated MySQL database for each app. This allowed for easy database management and scaling as your app grows.
What are the system requirements for running a CodeIgniter web app on PHPFog?
To run a CodeIgniter web app on PHPFog, you needed a PHPFog account, a Git client for deploying your code, and a CodeIgniter web app. PHPFog supported PHP 5.3 and above, and MySQL 5.1 and above.
Andy Hawthorne is from Coventry in the UK. He is a senior PHP developer by day, and a freelance writer by night, although lately that is sometimes the other way around.