This is very useful for loading mutiple scripts with a callback function containing code that you want to run only when all of the scripts have been loaded. To load mutiple scripts you need to enhance the AJAX $.getScript() function to handle mutiple scripts then simply add them to an array for the first parameter and the callback function as the second parameter.
Single jQuery Get Script
$.getScript('script1.js', function(data, textStatus) {
//do something after script has loaded
});
Multiple jQuery Get Scripts
/* enhance $.getSctipt to handle mutiple scripts */
var getScript = jQuery.getScript;
jQuery.getScript = function( resources, callback ) {
var // reference declaration & localization
length = resources.length,
handler = function() { counter++; },
deferreds = [],
counter = 0,
idx = 0;
for ( ; idx < length; idx++ ) {
deferreds.push(
getScript( resources[ idx ], handler )
);
}
jQuery.when.apply( null, deferreds ).then(function() {
callback && callback();
});
};
$.getScript(['script1.js','script2.js','script3.js'], function() {
//do something after all scripts have loaded
});
//or seperate into an array to include
var scripts = ['script1.js','script2.js','script3.js'];
$.getScript(scripts, function(data, textStatus) {
//do something after all scripts have loaded
});
Any way I discovered:
You could just do it this way, say you had to load 3 scripts and the third has a callback.
$.get("js/ext/flowplayer-3.2.8.min.js")
.pipe($.get("js/eviflowplayer.js"))
.pipe($.get("js/evi.flowplayer.js", {}, function()
{
W.EVI.FLOWPLAYER.init(elem.attr('id'));
});
$.get("js/ext/flowplayer-3.2.8.min.js")
.pipe($.get("js/eviflowplayer.js"))
.pipe($.get("js/evi.flowplayer.js", {}, function()
{
W.EVI.FLOWPLAYER.init(elem.attr('id'));
});
Frequently Asked Questions (FAQs) about getScript and Multiple Scripts
What is getScript and how does it work?
getScript is a method in jQuery that loads and executes a JavaScript file using an HTTP GET request. It’s a shorthand Ajax function, which is equivalent to the $.ajax() method. The getScript method is particularly useful when you want to load and execute external JavaScript files on demand. It works by sending a GET HTTP request to the server to fetch the script, then it executes the script.
How can I use getScript to load multiple scripts?
To load multiple scripts using getScript, you can chain the getScript calls. This means calling getScript within the callback function of another getScript. This ensures that the scripts are loaded and executed in the correct order. However, this can lead to callback hell if you have many scripts to load. To avoid this, you can use promises or async/await to handle the asynchronous nature of getScript.
What are the benefits of using getScript?
Using getScript has several benefits. First, it allows you to load and execute JavaScript files on demand, which can improve the performance of your web page by reducing the initial load time. Second, it allows you to organize your code better by separating it into different files. Third, it allows you to reuse your code across different web pages by loading the same script file.
Can I use getScript with other JavaScript libraries or frameworks?
Yes, you can use getScript with other JavaScript libraries or frameworks as long as they support the $ object and the getScript method. However, you should be aware that the behavior of getScript might be different in different libraries or frameworks.
How can I handle errors when using getScript?
You can handle errors when using getScript by using the .fail() method. This method is called when the request fails. You can use it to display an error message or to perform some other action when the script fails to load.
Can I use getScript to load scripts from different domains?
Yes, you can use getScript to load scripts from different domains. This is known as Cross-Origin Resource Sharing (CORS). However, you should be aware that not all servers allow CORS, and trying to load a script from a server that doesn’t allow CORS will result in an error.
Can I use getScript to load scripts synchronously?
By default, getScript loads scripts asynchronously. This means that it doesn’t block the execution of the rest of your code while it’s loading the script. However, you can change this behavior by using the $.ajax() method with the async option set to false.
Can I use getScript to load non-JavaScript files?
No, getScript is designed to load and execute JavaScript files. Trying to load a non-JavaScript file with getScript will result in an error.
Can I use getScript to load scripts that depend on each other?
Yes, you can use getScript to load scripts that depend on each other. However, you should be aware that getScript loads scripts asynchronously by default, which means that it doesn’t guarantee the order of execution. To ensure that the scripts are executed in the correct order, you can chain the getScript calls or use promises or async/await.
Can I use getScript in a loop to load multiple scripts?
Yes, you can use getScript in a loop to load multiple scripts. However, you should be aware that getScript loads scripts asynchronously by default, which means that it doesn’t guarantee the order of execution. If the order of execution is important, you should chain the getScript calls or use promises or async/await.
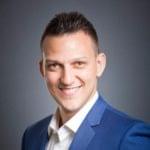
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.