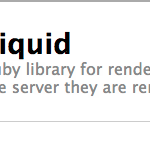
But, what’s wrong with ERB?
ERB is great for certain types of apps—but it does have some shortcomings, both aesthetic and practical.Full Ruby
What’s the saying? ‘With great power comes terrible separation of concerns.’ ERB pretty much gives you access to the full the complete and terrifying power of Ruby. The temptation will always be close to flirt with models and libraries which have no business being called in view code. Sure, just once isn’t a crime but the temptation is there and so is the slippery slope. Also, being able to execute arbitrary code in your views means your view layer is stuck in—house. You won’t be able to let outside folk create themes for your app (if that’s a thing you’d like to them to do) because they’re only a back tick and badly set up server away from causing some real damage, should you have a falling out with them.Things could be nicer
If there’s one thing that’s true about the Ruby community—perhaps to a fault sometimes—is that there’s a general desire for code to be beautiful. ERB isn’t beautiful, but some efforts like Moustache and HAML allow much of the power of ERB while improving on the aesthetics.Using Liquid
My preferred templating language is Liquid. Liquid was extracted from Shopify and powers their shop building efforts. My appreciation for liquid is two fold: First, it allows nice safe templates I can allow (power) users to edit in much the same way as Shopify does. Second, it forces a cleaner distinction between the data layer of your application and the presentation layer.Getting started
As with most things Rails, installation is a breeze (head over to the Liquid homepage for up to date instructons). Generally speaking, liquid templates are parsed and and then rendered with a context. The context, in most simple cases, need only be a Hash. When writing the template, each key in the hash becomes the name for variable with it’s value fully available to you. Substitutions for these keys can be made by using double curlys: {{x}} [gist id=’3202509′]Filters
One of the features that I love in liquid is filters. Filters behave in much the same way, and should be used for much the same purpose, as helpers in Rails. They’re short methods which aid in the presenting of information. Let’s take a look at how we can use filters. [gist id=’3202511′] As you might guess, this will output the string HELLOWORLD. There are a few things to remember here. Each pipe signifies that the output of the previous section will be passed to the next, so ‘world’ is passed to the prepend method with the additional argument hello which is in the passed to the method upcase—so we can get shouty.Tags & blocks
Filters are great, but you’ll eventually want to perform some logic, like an if statement or a for loop. For that, we’ve got tags and blocks. Tags, much like ERB, use an alternative syntax for logic sections: {%%}. This is best shown by example. [gist id=’3202513′]Extending liquid
So, that’s pretty much all you need to know to start using liquid for your templating needs. One of the awesome things about liquid, over the other similar templating engines, is how extendable it is.Filters
Filters are pretty much just helpers. They take one or more arguments, the first of which is the value to the left of the pipe (|), additional arguments are passed one by one after this. Let’s take a look at the implementation of the truncate filter from the standard set. [gist id=’3202515′] The value of ‘sometext’ will be passed through as input while length and truncatestring are passed through in order.Tags & blocks
Tags and blocks, as we’ve seen, provide extendibility beyond that of filters. To illustrate how useful blocks can be, we’ll write a block which allows our designer to process a specific twitter timeline and output it in any way he or she see’s fit. Usage will look something like: [gist id=’3118892′] The first step in creating a new block is to inherit fromLiquid::Block
and define a initializer. You’ll want to call super in your initializer on the first line, it’ll do a bunch of setup for you. The initializer takes three arguments.
- tag_name
- markup — the arguments passed to be block. In the usage example above, this would be the string
source: https://www.sitepoint.com/feed/
- tokens — a fully passed list of tokens. Unless your doing a fairly advanced tag that gets into the guts of liquid, you can ignore this.
Liquid::TagAttributes
, which will help us process the above string and come out with a hash in the way you’d expect.
The actual rendering in a liquid block is done in the render
method. The render
method always takes one argument: context. The context is the current state of the rendering stack. All of your inputs and data live in this data structure.
We’re creating a block which is going to create temporary variables that’ll be used only in the context of the block. This behaves just like a block in ruby (if you’re calling each on an array, for example.) We can temporarily push to the stack by calling #stack
on the context object and providing a block.
If you plan to have any output for your block-and in this case we definitely do—then the #render_all
method is the key. It takes two arguments: a context and a node list. If you’ve called super
in your initialiser then the nodelist will be provided as @nodelist
.
So, put all these pieces together and you should come up with something like this:
[gist id=’3118852′]
You’ll almost certainly want to add some caching and error handling here—I’ve left it out for readability.
It’s worth remebering that the the arguments to this block will always come through as strings and as such you won’t be able to use variables to populate the source out of the box. But, no matter—the context (which you’ll remember is passed to the render method) provides all the variables the designer has been using. Somewhere in the render block you’ll be able to pull out the variable value like this:
[gist id=’3220855′]
Notice how we first check if the context has the appropriate key. We have to do this because we have no way of knowing if the token refers to a variable or is just a string. Alternatively, for this particular tag you could check if the source looks like a URL before inspecting the context.
Wrap up
And so, I hope you’ll agree Liquid is pretty neat. It’s a powerful and expressive templating langauge that does a great job at providing you (‘the programmer’) the tools to allow your designers to build excellent sites without the worry that they’llrm -rf
the whole thing. With luck, I’ve been clear—but if not I’ll be lurking around the comment section if you have any questions.
Frequently Asked Questions (FAQs) about Using Liquid in Rails
What are the benefits of using Liquid over ERB in Rails?
Liquid is a powerful and safe templating engine used by many platforms, including Shopify. It offers several advantages over ERB. Firstly, it provides a secure environment where users can edit the application’s templates without affecting the server-side code. This is particularly useful in multi-tenant applications where each user needs to customize their interface. Secondly, Liquid has a simple and intuitive syntax that is easy to learn and use. It also supports advanced features like filters and tags, which can simplify complex operations.
How do I install and set up Liquid in a Rails application?
Installing Liquid in a Rails application is straightforward. You need to add the ‘liquid’ gem to your Gemfile and run the ‘bundle install’ command. After that, you can use Liquid templates in your views by changing the file extension from ‘.html.erb’ to ‘.liquid’. You can also use the ‘render’ method in your controllers to render Liquid templates.
Can I use Liquid filters in Rails, and how do they work?
Yes, you can use Liquid filters in Rails. Filters are simple methods that modify the output of numbers, strings, variables, and objects. They are placed within an output tag and are denoted by a pipe character ‘|’. For example, the ‘upcase’ filter can be used to convert a string to uppercase.
How can I create custom Liquid tags in Rails?
Creating custom Liquid tags in Rails involves defining a new class that inherits from ‘Liquid::Tag’ and implementing the ‘render’ method. This method takes a context object and returns a string that will replace the tag in the template. Once the tag class is defined, you can register it with Liquid using the ‘Liquid::Template.register_tag’ method.
How do I handle errors and exceptions in Liquid?
Liquid has a robust error handling mechanism. It catches and suppresses all exceptions by default, and replaces them with a blank string in the output. However, you can customize this behavior by setting the ‘error_mode’ option on the Liquid::Template object. You can also use the ‘errors’ method to retrieve a list of errors that occurred during rendering.
Can I use Liquid in combination with other templating engines like Haml or Slim?
Yes, you can use Liquid in combination with other templating engines like Haml or Slim. However, you need to be aware of the differences in syntax and behavior between these engines. For example, Haml uses indentation to denote nesting, while Liquid uses tags.
How do I test Liquid templates in Rails?
Testing Liquid templates in Rails can be done using the standard Rails testing tools. You can create a test that renders the template with a set of inputs and asserts that the output matches the expected result. You can also use libraries like Capybara to perform integration tests that involve Liquid templates.
How do I debug Liquid templates in Rails?
Debugging Liquid templates in Rails can be challenging due to the lack of traditional debugging tools like ‘binding.pry’. However, you can use the ‘inspect’ filter to output the value of a variable in the template. You can also use the ‘errors’ method to retrieve a list of errors that occurred during rendering.
Can I use Liquid to generate dynamic content in Rails?
Yes, you can use Liquid to generate dynamic content in Rails. You can pass variables from your controllers to your Liquid templates, and use these variables in combination with Liquid tags and filters to generate dynamic content.
How do I secure Liquid templates in Rails?
Securing Liquid templates in Rails involves restricting the types of operations that can be performed in the template. By default, Liquid disallows operations that could affect the server-side code or the underlying system. However, you can further restrict the available tags and filters by using a custom ‘Liquid::Context’ object.
Daniel Cooper is a web developer from Brighton, UK