The calculateSince function()
/**
* Calculates the Twitter time since the tweet was created
* @param datetime returned by Twitter API in created_at
* @return time since in html
*/
function calculateSince(datetime)
{
var tTime=new Date(datetime);
var cTime=new Date();
var sinceMin=Math.round((cTime-tTime)/60000);
if(sinceMin==0)
{
var sinceSec=Math.round((cTime-tTime)/1000);
if(sinceSec<10)
var since='less than 10 seconds ago';
else if(sinceSec<20)
var since='less than 20 seconds ago';
else
var since='half a minute ago';
}
else if(sinceMin==1)
{
var sinceSec=Math.round((cTime-tTime)/1000);
if(sinceSec==30)
var since='half a minute ago';
else if(sinceSec<60)
var since='less than a minute ago';
else
var since='1 minute ago';
}
else if(sinceMin<45)
var since=sinceMin+' minutes ago';
else if(sinceMin>44&&sinceMin<60)
var since='about 1 hour ago';
else if(sinceMin<1440){
var sinceHr=Math.round(sinceMin/60);
if(sinceHr==1)
var since='about 1 hour ago';
else
var since='about '+sinceHr+' hours ago';
}
else if(sinceMin>1439&&sinceMin<2880)
var since='1 day ago';
else
{
var sinceDay=Math.round(sinceMin/1440);
var since=sinceDay+' days ago';
}
return since;
};
Refresh the Time Since Tweeted
This is how you could use a setInterval to refresh the time since tweeted using the calculateSince function above.//auto refresh interval to load more tweets
setInterval(function()
{
console.log('updating time since...');
var tweets = $('#tweets .tweet');
$.each(tweets, function(i,v)
{
//update the time since for the tweet
$(v).find('.tweet-time').html(calculateSince($(v).find('.tweet-user').attr('created_at'))).fadeIn();
});
}, 30000);
Frequently Asked Questions about Calculating Twitter Time to Tweet with JavaScript
How can I authenticate my Twitter API request using JavaScript?
To authenticate your Twitter API request using JavaScript, you need to use OAuth 2.0. OAuth 2.0 is a protocol that allows a user to grant limited access to their resources on one site, to another site, without having to expose their credentials. You can use libraries like jsOAuth which is a JavaScript implementation of the OAuth protocol. You will need to create an application on the Twitter Developer portal to get the API keys required for authentication.
Can I schedule tweets using JavaScript?
No, Twitter API does not support scheduling tweets directly. However, you can build your own scheduling feature using JavaScript. You can create a function that delays the execution of the tweet post request. However, remember that the user’s browser must be open for the JavaScript to run.
How can I handle errors when posting tweets using JavaScript?
When posting tweets using JavaScript, you can handle errors by using the catch block in your promise chain. The catch block will be executed when the promise is rejected, which usually happens when there is an error. In the catch block, you can log the error message to understand what went wrong.
How can I include images in my tweets using JavaScript?
To include images in your tweets using JavaScript, you need to use the media/upload endpoint to upload the image to Twitter first. After uploading, you will receive a media_id which you can use in the statuses/update endpoint to attach the image to your tweet.
How can I retweet a tweet using JavaScript?
To retweet a tweet using JavaScript, you need to use the statuses/retweet/:id endpoint. Replace :id with the id of the tweet you want to retweet. This will create a new tweet with the original tweet embedded.
How can I delete a tweet using JavaScript?
To delete a tweet using JavaScript, you need to use the statuses/destroy/:id endpoint. Replace :id with the id of the tweet you want to delete. This will delete the tweet from Twitter.
How can I get the number of retweets of a tweet using JavaScript?
To get the number of retweets of a tweet using JavaScript, you need to use the statuses/show/:id endpoint. Replace :id with the id of the tweet you want to get information about. The response will include a retweet_count field which contains the number of retweets.
How can I get the number of likes of a tweet using JavaScript?
To get the number of likes of a tweet using JavaScript, you need to use the statuses/show/:id endpoint. Replace :id with the id of the tweet you want to get information about. The response will include a favorite_count field which contains the number of likes.
How can I reply to a tweet using JavaScript?
To reply to a tweet using JavaScript, you need to use the statuses/update endpoint. In the status parameter, include the @username of the user you want to reply to. In the in_reply_to_status_id parameter, include the id of the tweet you want to reply to.
How can I get the user who posted a tweet using JavaScript?
To get the user who posted a tweet using JavaScript, you need to use the statuses/show/:id endpoint. Replace :id with the id of the tweet you want to get information about. The response will include a user field which contains information about the user who posted the tweet.
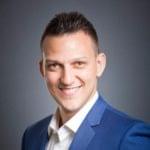
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.