Once again, I’ve gathered some of the useful jQuery tips and tricks for you to see how great jQuery can be.
Here they are:
1. Preloading Images
With this trick you could preload images in JavaScript before using them.
jQuery.preloadImages = function()
{
for(var i = 0; i ").attr("src", arguments[i]);
}
};
// Usage
$.preloadImages("image1.gif", "/path/to/image2.png", "some/image3.jpg");
2. Target Blank Links
Avoid XHTML 1.0 to restrict your target=blank code. Use this jQuery trick to do such thing.
$('a[@rel$='external']').click(function(){
this.target = "_blank";
});
/*
Usage:
lepinskidesign.com.br
*/
3. Get the total number of matched elements
$('element').size();
4. Detect Browser
CSS’ conditional comment is pretty useful to detect browser and apply some css style.
//A. Target Safari
if( $.browser.safari ) $("#menu li a").css("padding", "1em 1.2em" );
//B. Target anything above IE6
if ($.browser.msie && $.browser.version > 6 ) $("#menu li a").css("padding", "1em 1.8em" );
//C. Target IE6 and below
if ($.browser.msie && $.browser.version = "1.8" ) $("#menu li a").css("padding", "1em 1.8em" );
5. Columns of equal Height
Yes this is possible with the following jQuery trick.
function equalHeight(group) {
tallest = 0;
group.each(function() {
thisHeight = $(this).height();
if(thisHeight > tallest) {
tallest = thisHeight;
}
});
group.height(tallest);
}
/*
Usage:
$(document).ready(function() {
equalHeight($(".recent-article"));
equalHeight($(".footer-col"));
});
*/
6. Font Resize
Resize fonts using jQuery.
$(document).ready(function(){
// Reset Font Size
var originalFontSize = $('html').css('font-size');
$(".resetFont").click(function(){
$('html').css('font-size', originalFontSize);
});
// Increase Font Size
$(".increaseFont").click(function(){
var currentFontSize = $('html').css('font-size');
var currentFontSizeNum = parseFloat(currentFontSize, 10);
var newFontSize = currentFontSizeNum*1.2;
$('html').css('font-size', newFontSize);
return false;
});
// Decrease Font Size
$(".decreaseFont").click(function(){
var currentFontSize = $('html').css('font-size');
var currentFontSizeNum = parseFloat(currentFontSize, 10);
var newFontSize = currentFontSizeNum*0.8;
$('html').css('font-size', newFontSize);
return false;
});
});
7. Remove a word in a text
Remove any word in a text using this jQuery trick.
var el = $('#id');
el.html(el.html().replace(/word/ig, ""));
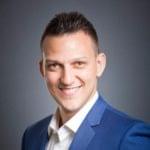
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.