- 1. Shortcut for “Ready” event
- 2. Element Checker
- 3. Properly rename your jQuery object when using another framework
- 4. Compress your jQuery scripts
- 5. Minimize DOM Manipulation
- 6. Give Context for your Selectors
- 7. Proper usage of animation
- 8. Do your own Selectors
- 9. Speed up content load for SEO benefits
- 10. Use CHEAT SHEET
- Frequently Asked Questions (FAQs) on Improving jQuery Performance
There’s jQuery almost everywhere, you can find it on famous websites available today including social networking websites, micro blogs, etc. jQuery is indeed cool and very useful, but sometimes it’s best to see a different flavor or another side of it. Or perhaps make improvements for it. Improving jQuery would make your website load faster and do similar things that will help your website stand out. This could include a jQuery script or code via Google codes and stuffs like that. Like I said, it’s best to improve or edit a jQuery plugin or syntax that will be used by your website to make it more prominent. What I have here is a list of ten tips to improve your jQuery.
1. Shortcut for “Ready” event
Are you tired of typing this?$(document).ready(function (){
// your code
});
Well you can make a shortcut for it, try this:
$(function (){
// your code
});
2. Element Checker
Be sure to check if the element really exists on the page before you manipulate it. Use this simple but not obvious code:if ($('#myDiv).length) {
// your code
}
3. Properly rename your jQuery object when using another framework
Use the noconflict() jQuery function to take control of $ back and allow yourself to set your own variable name.var $j = jQuery.noConflict();
$j('#myDiv').hide();
4. Compress your jQuery scripts
A big project probably means a lot of jQuery plugin usage too. But be aware that this might slow down your page, so you should compress all of your jQuery by using Packer by Dean Edwards. It’s a web based javascript compressor.5. Minimize DOM Manipulation
Insertion operations of DOM such as .prepend() .append() .wrap() and .after() would really slow things down. You could make codes faster; all you need to do is build the list by using concatenation then after that, a single use of function to add them to your list that’s unordered like .html() is much quicker. Example:var myList = $('#myList');
for (i=0; i<1000; i++){
myList.append('This is list item ' + i);
}
That example is relatively slow, but if you build the list item as a string and use the html method to do the insertion. You might want to try the following instead:
Example:
var myList = $('.myList');
var myListItems = '';
for (i = 0; i < 1000; i++) {
myListItems += 'This is list item ' + i + '';
}
myList.html(myListItems);
That would make the page load faster!
6. Give Context for your Selectors
Normally if you use a selector like $(‘.myDiv’) DOM would definitely traversed, which depends on the page could be expensive. Performing selections would make the jQuery take a second parameter. jQuery( expression, context ) By putting context into a single selector, you would give that selector an element to start with so it doesn’t have to traverse the whole of the DOM. Before:var selectedItem = $('#listItem' + i);
After:
var selectedItem = $('#listItem' + i, $('.myList'));
That should speed things up!
7. Proper usage of animation
Animation is the core power of jQuery. It’s really cool and provides very eye catchy effects. jQuery’s .animate() method is very easy to use and powerful. If you look at the codes internally those methods are just shortened and uses the .animate() function. Example:slideDown: function(speed,callback){
return this.animate({height: "show"}, speed, callback);
},
fadeIn: function(speed, callback){
return this.animate({opacity: "show"}, speed, callback);
}
The animate() function simply changes the element’s CSS properties such as height, width, color, opacity, background-color, etc.
Example:
$('#myList li').mouseover(function() {
$(this).animate({"height": 100}, "slow");
});
Unlike jQuery other functions, animations are automatically queued. If you want to execute another animation when the first animation is finished then just call the animate method twice.
Example:
$('#myBox').mouseover(function() {
$(this).animate({ "width": 200 }, "slow");
$(this).animate({"height": 200}, "slow");
});
If you want multiple animations, do it this way:
$('#myBox').mouseover(function() {
$(this).animate({ "width": 200, "height": 200 }, "slow");
});
8. Do your own Selectors
jQuery has many built-in selectors for selection of elements by ID, tag, attribute and many more. But what would you do if you need to select elements based on something else wherein jQuery doesn’t have a selector. Probably, you would add classes to the lements from the beginning and use those to select them. But it beats the purpose of jQuery’s ease of extension to add new selectors. Example:$.extend($.expr[':'], {
over100pixels: function(a) {
return $(a).height() > 100;
}
});
$('.box:over100pixels').click(function() {
alert('The element you clicked is over 100 pixels high');
});
Creation of custom selector is in the first block of code which finds any elements that’s more than 100 pixels tall.
9. Speed up content load for SEO benefits
If you think that if you neaten up your HTML code it would make your page load faster. You are right, search spiders is too lazy to load whole code using an AJAX request after the rest of the page has loaded. User can start browsing right away and spiders only see the content you wish them to index. Example:$('#forms').load('content/headerForms.html', function() {
// Code here runs once the content has loaded
// Put all your event handlers etc. here.
});
10. Use CHEAT SHEET
Getting tired of jQuery tips? Here are some great cheat sheets available for most languages! It’s a printable jQuery functions on an A4 sheet for your own reference. http://www.gscottolson.com/weblog/2008/01/11/jquery-cheat-sheet/ http://colorcharge.com/jquery/Frequently Asked Questions (FAQs) on Improving jQuery Performance
What are some common mistakes that can slow down jQuery performance?
There are several common mistakes that can slow down jQuery performance. One of the most common is using inefficient selectors. For example, using class selectors can be slower than using ID selectors. Another common mistake is not caching jQuery objects. Every time you use a jQuery selector, it has to search the entire DOM. By caching jQuery objects, you can avoid this unnecessary search. Lastly, using too many animations or effects can also slow down performance. It’s important to use these sparingly and only when necessary.
How can I optimize jQuery selectors for better performance?
Optimizing jQuery selectors can significantly improve performance. Here are a few tips:
- 1. Use ID selectors whenever possible as they are the fastest.
- 1. Avoid universal selectors as they are the slowest.
- 1. Be specific with your selectors. The more specific you are, the less the browser has to search.
- 1. Cache your selectors. This means storing them in a variable so you can reuse them without having to search the DOM again.
How can I use chaining to improve jQuery performance?
Chaining is a powerful feature in jQuery that allows you to run multiple jQuery methods (or functions) on the same element without selecting the element again. This can significantly improve performance as it reduces the number of times the DOM has to be traversed. To use chaining, simply append your methods one after the other. For example, instead of writing $('#myDiv').hide(); $('#myDiv').css('color', 'red');
, you can chain these methods together like this: $('#myDiv').hide().css('color', 'red');
.
How can event delegation improve jQuery performance?
Event delegation is a technique in jQuery where you bind an event handler to a parent element instead of binding it to the specific elements that trigger the event. This can improve performance, especially when dealing with dynamic elements (elements that are added or removed after the page has loaded). With event delegation, you only need to bind the event handler once, instead of every time a new element is added.
How can I use the .data() method to improve jQuery performance?
The .data() method in jQuery allows you to attach data of any type to DOM elements. This can improve performance as it reduces the need to make unnecessary DOM searches. For example, instead of repeatedly searching the DOM for a particular value, you can store that value using the .data() method and retrieve it whenever you need it.
How can I use the .end() method to improve jQuery performance?
The .end() method in jQuery allows you to end the most recent filtering operation in the current chain and return the set of matched elements to its previous state. This can improve performance as it reduces the need to make unnecessary DOM searches. For example, instead of repeatedly searching the DOM for a particular element, you can use the .end() method to return to the original set of matched elements.
How can I use the .filter() method to improve jQuery performance?
The .filter() method in jQuery allows you to narrow down the search for elements in a jQuery object. This can improve performance as it reduces the number of elements that need to be searched. For example, instead of searching all the elements in a jQuery object, you can use the .filter() method to only search the elements that meet certain criteria.
How can I use the .find() method to improve jQuery performance?
The .find() method in jQuery allows you to search for elements within a jQuery object. This can improve performance as it reduces the need to make unnecessary DOM searches. For example, instead of searching the entire DOM for a particular element, you can use the .find() method to only search within a specific jQuery object.
How can I use the .each() method to improve jQuery performance?
The .each() method in jQuery allows you to loop through elements in a jQuery object. This can improve performance as it reduces the need to make unnecessary DOM searches. For example, instead of searching the DOM for each individual element, you can use the .each() method to loop through all the elements in a jQuery object at once.
How can I use the .ready() method to improve jQuery performance?
The .ready() method in jQuery allows you to make sure that the DOM is fully loaded before running any jQuery code. This can improve performance as it ensures that your jQuery code doesn’t run until all the elements are available. For example, instead of running your jQuery code immediately and potentially causing errors or slow performance, you can use the .ready() method to wait until the DOM is fully loaded.
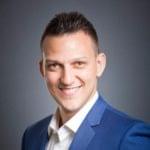
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.