Today, I wanted to use the Public Twitter Search API and grab the latest 5 tweets tagged “jquery4u”. Here is how you can do it yourself. Update 09/10/13: Twitter search API is deprecated.
The JavaScript/jQuery
Here we can access the public feed from the Twitter search API and specify a JSON callback for the data. Parameters: q = the search string (make sure it’s uri encoded) rpp = the number of tweets we want to get//get the JSON data from the Twitter search API
$.getJSON("http://search.twitter.com/search.json?q=jquery4u&rpp=5&callback=?", function(data)
{
//loop the tweets
$(data.results).each(function(i,v)
{
//...see full code below
}
}
The HTML
<div id="twitter-widget"></div>
The CSS
.twitStream{
font-family: verdana;
font-size: 11px;
}
.twitStream a{
font-family: verdana;
font-size: 11px;
}
.tweet{
display: block;
padding: .4em;
margin: .4em 0;
}
.tweet-left{
float: left;
margin-right: 1em;
}
.tweet-left img{
border: 2px solid #000000;
}
.tweet p.text{
margin: 0;
padding: 0;
}
Full Code Listing
Here is the full JavaScript Object for the Twitter Widget./**
* JQUERY4U
*
* Displays the latest tweets.
*
* @author Sam Deering
* @copyright Copyright (c) 2012 JQUERY4U Pty Ltd
* @license http://jquery4u.com/license/
* @since Version 1.0
* @filesource js/jquery4u.twitter.js
*
*/
(function($,W,D)
{
W.JQUERY4U.TWITTER = {
name: "JQUERY4U TWITTER",
init: function(wid)
{
//helper functions
String.prototype.linkify=function(){
return this.replace(/[A-Za-z]+://[A-Za-z0-9-_]+.[A-Za-z0-9-_:%&;?/.=]+/g,function(m){
return m.link(m);
});
};
String.prototype.linkuser=function(){
return this.replace(/[@]+[A-Za-z0-9-_]+/g,function(u){
return u.link("http://twitter.com/"+u.replace("@",""));
});
};
String.prototype.linktag=function(){
return this.replace(/[]+[A-Za-z0-9-_]+/,function(t){
return t;
});
};
//load twitter stylesheet
$("head").append('');
//get the tweets from Twitter API
$.getJSON("http://search.twitter.com/search.json?q=jquery4u&rpp=5&callback=?", function(data)
{
// console.log(data.items.length);
$(data.results).each(function(i,v)
{
var tTime=new Date(Date.parse(this.created_at));
var cTime=new Date();
var sinceMin=Math.round((cTime-tTime)/60000);
if(sinceMin==0){
var sinceSec=Math.round((cTime-tTime)/1000);
if(sinceSec<10)
var since='less than 10 seconds ago';
else if(sinceSec<20)
var since='less than 20 seconds ago';
else
var since='half a minute ago';
}
else if(sinceMin==1){
var sinceSec=Math.round((cTime-tTime)/1000);
if(sinceSec==30)
var since='half a minute ago';
else if(sinceSec<60)
var since='less than a minute ago';
else
var since='1 minute ago';
}
else if(sinceMin<45)
var since=sinceMin+' minutes ago';
else if(sinceMin>44&&sinceMin<60)
var since='about 1 hour ago';
else if(sinceMin<1440){
var sinceHr=Math.round(sinceMin/60);
if(sinceHr==1)
var since='about 1 hour ago';
else
var since='about '+sinceHr+' hours ago';
}
else if(sinceMin>1439&&sinceMin<2880)
var since='1 day ago';
else{
var sinceDay=Math.round(sinceMin/1440);
var since=sinceDay+' days ago';
}
var tweetBy='<a class="tweet-user" href="http://twitter.com/'+this.from_user+'" target="_blank" rel="noopener">@'+this.from_user+'</a> <span class="tweet-time">'+since+'</span>';
tweetBy=tweetBy+' · <a class="tweet-reply" href="http://twitter.com/?status=@'+this.from_user+' &in_reply_to_status_id='+this.id+'&in_reply_to='+this.from_user+'" target="_blank" rel="noopener">Reply</a>';
tweetBy=tweetBy+' · <a class="tweet-view" href="http://twitter.com/'+this.from_user+'/statuses/'+this.id+'" target="_blank" rel="noopener">View Tweet</a>';
tweetBy=tweetBy+' · <a class="tweet-rt" href="http://twitter.com/?status=RT @'+this.from_user+' '+escape(this.text.replace(/"/g,'" target="_blank" rel="noopener">RT</a>';
var tweet='
<div class="tweet">
<div class="tweet-left"><a href="http://twitter.com/'+this.from_user+'" target="_blank" rel="noopener"><img src="'+this.profile_image_url+'" alt="'+this.from_user+' on Twitter" width="48" height="48" /></a></div>
<div class="tweet-right">
<p class="text">'+this.text.linkify().linkuser().linktag().replace(/'+tweetBy+'</p>
</div>
</div>
';
$("#twitter").append(tweet); //add the tweet...
});
});
}
}
})(jQuery,window,document);
Usage
jQuery(document).ready(function($) {
JQUERY4U.TWITTER.init();
});
Some of the code used above is courtesy of TwitStream. Thanks guys.
Frequently Asked Questions about Twitter Search API
What is Twitter Search API and how does it work?
Twitter Search API is a function provided by Twitter that allows developers to interact with Twitter data. It works by sending HTTP requests to Twitter’s servers, which then return data in a structured format. This data can be tweets, user profiles, or other types of information available on Twitter. Developers can use this data to build applications, analyze trends, or for other purposes.
How can I use Twitter Search API for marketing purposes?
Twitter Search API can be a powerful tool for marketing. By analyzing tweets, you can understand what people are saying about your brand, identify trends, and even find potential customers. For example, you can search for tweets containing certain keywords related to your product, and then engage with those users directly.
What are the limitations of Twitter Search API?
While Twitter Search API is a powerful tool, it does have some limitations. For example, it only returns tweets from the past 7 days, and there are rate limits on how many requests you can make in a certain period of time. Additionally, not all tweets are available through the API, especially older ones.
How can I get started with Twitter Search API?
To get started with Twitter Search API, you first need to create a Twitter Developer account and apply for API access. Once you have access, you can start making requests to the API using HTTP requests. There are also many libraries available in various programming languages that can simplify this process.
Can I use Twitter Search API to track hashtags?
Yes, you can use Twitter Search API to track hashtags. By sending a request to the API with a specific hashtag as the query, you can get a list of tweets containing that hashtag. This can be useful for tracking the popularity of a hashtag, or for analyzing the conversation around a specific topic.
Is there a cost associated with using Twitter Search API?
Twitter Search API is free to use, but there are rate limits on how many requests you can make. If you need to make more requests, you can upgrade to a premium or enterprise plan, which have higher rate limits but come with a cost.
How can I analyze the data returned by Twitter Search API?
The data returned by Twitter Search API is in a structured format, which makes it easy to analyze. You can use programming languages like Python or R to analyze the data, or you can use data visualization tools to create charts and graphs. You can analyze trends, sentiment, and more.
Can I use Twitter Search API to stream tweets in real-time?
Yes, Twitter provides a Streaming API that allows you to stream tweets in real-time. This can be useful for tracking live events, monitoring brand mentions, or for other real-time applications.
What kind of data can I get from Twitter Search API?
Twitter Search API can return a wide variety of data, including tweets, user profiles, and more. Each tweet contains a lot of information, including the text of the tweet, the user who posted it, the time it was posted, and more. You can also get information about the user, such as their profile description, location, and follower count.
How can I handle errors when using Twitter Search API?
When using Twitter Search API, you may encounter errors, such as rate limit errors or errors due to invalid requests. These errors are returned in the HTTP response from the API. You should handle these errors in your code, for example by waiting and retrying when you hit a rate limit, or by fixing the request if it’s invalid.
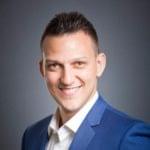
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.