Because the action is not followed we may need to know when the user prompted the action and can use event.isDefaultPrevented()
to determine if this method has been called by an event handler that was triggered by this event. This can be used to trigger a function call. Could also be useful for dynamic URL’s with XHtmlRequest in jQuery to fetch a page with a module for example href=”index.php?page=contact instead of contact.php. You may also want to look at MOD Rewrite options which can also provide this functionality and SEO goodness. jQuery Stop Event Functions. Watch out if you’re using $(document).bind(“keydown keypress”, function(event) then e.preventDefault() won’t work try.. event.preventDefault(). Sometimes you have a hyperlink that needs to be a hyperlink but you don’t want it to process and open the link but only call a javascript function say. Fortunately there is a function in jQuery to stop the hyperlink action.
jQuery Preventdefault Function Demo
Example 1 – Prevent and catch a hyperlink click
< !DOCTYPE html>
default click action is prevented
$("a").click(function(event) {
event.preventDefault();
$('<div>')
.append('default ' + event.type + ' prevented')
.appendTo('#log');
});
Example 2 – Prevent form submit button
$('#myform').submit(function(event) {
event.preventDefault();
var self = this;
window.setTimeout(function() {
self.submit();
}, 2000);
});
Example 3 – Delay hyperlink target until after animation effect
$("#ELEMENT_WHICH_AFFECT_THE_SLIDEUP")
.click(function(event){
event.preventDefault(); // prevents link to be redirected
var time = 1000; // time for slideup effect
var url = $(this).attr("href"); // pick url for redirecting via javascript
$("#ELEMENT_TO_SLIDE_UP").slideUp(time); // effect
window.setTimeout(function(){document.location.href=url;}, time); // timeout and waiting until effect is complete
return -1;
});
Example 4 – Disable keydown scroll button
$(document).keydown(function(event){
// Move Down
if(event.keyCode == '40'){
event.preventDefault();
var posY = $('#'+selectedTxtID).css('top');
posY = parseFloat(posY);
var newPosY = posY + 1;
$('#'+selectedTxtID).css('top', newPosY+'px');
}
})
This function can also be used not only on hyperlinks but anything that has a default action you wish to prevent.
Frequently Asked Questions (FAQs) about jQuery’s preventDefault() Method
What is the main function of the preventDefault() method in jQuery?
The preventDefault() method in jQuery is primarily used to stop the default action of an event from occurring. For instance, if you have a link on your webpage and you don’t want it to redirect to another page when clicked, you can use the preventDefault() method to stop this default action. This method is particularly useful when you want to control event actions in your code, providing a higher level of interactivity in your web applications.
How does the preventDefault() method differ from the stopPropagation() method?
While both methods are used to control event actions, they serve different purposes. The preventDefault() method stops the default action of an event from happening, while the stopPropagation() method prevents the event from bubbling up the DOM tree, preventing any parent handlers from being notified of the event. In other words, preventDefault() deals with the default behavior of an element, while stopPropagation() deals with the event flow in the DOM hierarchy.
Can I use the preventDefault() method with all events?
No, the preventDefault() method cannot be used with all events. It only works with events that have a default action to be prevented. For example, it can be used with the ‘click’ event of an anchor tag to prevent the default action of navigating to a new URL. However, it cannot be used with custom events that do not have a default action.
How can I check if the preventDefault() method has been called for an event?
You can use the isDefaultPrevented() method to check if preventDefault() was called on an event. This method returns a Boolean value – true if preventDefault() was called, and false otherwise.
Can I use the preventDefault() method in Internet Explorer?
Yes, you can use the preventDefault() method in Internet Explorer. However, older versions of Internet Explorer (IE8 and below) do not support this method. For these versions, you can use the returnValue property of the event object to achieve the same effect.
What happens if I call preventDefault() on a non-cancelable event?
If you call preventDefault() on a non-cancelable event, the method will have no effect. Non-cancelable events are those events whose default action cannot be prevented. You can check if an event is cancelable or not by using the cancelable property of the event object.
Can I call preventDefault() multiple times for the same event?
Yes, you can call preventDefault() multiple times for the same event, but it will not have any additional effect. Once the default action of an event is prevented, calling preventDefault() again will not change anything.
Can I use preventDefault() with keyboard events?
Yes, you can use preventDefault() with keyboard events. For instance, you can prevent the default action of the ‘keydown’ event to stop a character from being entered into a text field.
How can I use preventDefault() in a conditional statement?
You can use preventDefault() in a conditional statement to control when the default action of an event should be prevented. For example, you can prevent a form from being submitted if certain validation checks fail.
Can I use preventDefault() with touch events?
Yes, you can use preventDefault() with touch events. For instance, you can prevent the default action of the ‘touchstart’ event to stop a touch gesture from being recognized.
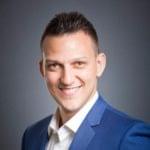
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.