Progressive Enhancement Techniques 2: the CSS
Key Takeaways
- Progressive Enhancement in CSS starts with a simple, functional website and adds more complex functionalities for advanced browsers or devices, making sure the content is accessible to all users, including those with disabilities and search engine bots.
- CSS should be kept simple and functional without JavaScript, and any JavaScript-dependent styling changes should be implemented by adding a class to appropriate DOM nodes after the page has loaded. This ensures that the CSS is only activated if the script runs successfully.
- When styling a tabbed box using CSS, the tabs can be floated to the left with a 10px margin, and the tab colors and borders specified. The active tab can be highlighted using JavaScript-enabled CSS, and the content area can be styled to ensure it remains functional without JavaScript.
In my previous article we built a tab control using Plain Old Semantic HTML (POSH). It worked in all browsers, but wouldn’t win any design awards. This is the second of a three-part series that illustrates how to build a simple tabbed box using progressive enhancement techniques.
The Luscious Layer
CSS is the web page layer that adds the layout, styling and background graphics to your page. Although CSS properties are well-documented and (mostly) logical, devices will have their own ideas about rendering. The browsers that will cause the most grief are IE6 and, to a lesser extent, IE7 — both are old compared to the competition but a large proportion of people continue to use them. It is possible to overcome most issues, but please remember:
Pixel-perfection is a myth!
If you need an accurately-designed layout you should forget the web — distribute your masterpiece on paper or as a PDF instead! IT systems vary immensely: people use different operating systems, screen sizes, DPI settings, font typefaces, color depths and browsers. CSS can only suggest a layout — it is up to the browser to interpret your code and it may not always do what you expect. Users will rarely notice a widget being a few pixels out of position, but they will complain if the functionality is broken.
Unobtrusive CSS
Our tab control HTML has been coded to work in any browser. We will use CSS to style and improve the layout so it looks like a tabbed box, but we must be careful not to damage our accessibility efforts.
Always be wary about implementing any CSS that depends on scripting. For example, inactive content in our tab box could be hidden using the CSS display: none;
property. However, that content would never appear if JavaScript were disabled. In general:
- Keep your CSS simple.
- Ensure your CSS works without JavaScript.
- If your JavaScript implements styling changes, use that script to add a class to appropriate DOM nodes after the page has loaded. Therefore, JavaScript-dependent CSS is only activated if the script runs successfully.
For example, your script could add a class of “jsenabled” to the
body
tag which triggers CSS styling changes:p { ... styles applied always ... } body.jsenabled p { ... styles applied when JS executes ... }
Note that it is possible to disable CSS and enable JavaScript. It’s less likely, but we must not make assumptions about our users!
Styling the Tabbed Box
The tabs can be styled using the following CSS:
/* tab control CSS: the tabs */
.tabs
{
position: relative;
bottom: -1px;
height: 20px;
list-style-type: none;
padding: 0;
margin: 0;
}
.tabs li
{
display: inline;
float: left;
padding: 0;
margin: 0 0 0 10px;
}
.tabs a
{
display: block;
width: 5em;
height: 18px;
line-height: 18px;
text-align: center;
text-decoration: none;
color: #555;
background-color: #ccc;
border: 1px solid #999;
outline: 0 none;
}
.tabs a.active, .tabs a:hover, .tabs a:focus, .tabs a:active
{
color: #222;
background-color: #fff;
border-bottom-color: #fff;
}
The code floats the list elements to the left with a 10px margin. The a
link selectors specify the tab colors and borders. The ul
tag is positioned 1px down so the tabs are rendered on top of the border that is applied around the content.
The .tabs a.active
selector is an example of JavaScript-enabled CSS. In this case, the styles will be applied when JavaScript assigns a class of “active” to a tab.
The tab content area is styled using the following CSS:
.tabcontent
{
width: 26em;
height: 10em;
margin: 0 0 2em 0;
border: 1px solid #999;
overflow: auto;
}
.tabcontent div
{
height: 10em;
margin: 0 10px;
}
.tabcontent div h2
{
padding-top: 10px;
margin-top: 0;
}
The outer div
(class of “tabcontent”) is set to 10em in height and has overflow
set to auto so scrollbars appear when required. Our content blocks (the inner div
elements) are also set to a height of 10em.
The result is a tabbed box with a scrolling inner area. The user can access content using the scrollbars or by clicking the tabs to jump to the correct place.
The CSS has made the content easier to view and it remains functional without JavaScript. However, it’s not perfect: the active tab is not highlighted and scrollbars are a little clunky for a slick web application.
In the next post, we create a reusable JavaScript module that adds the final enhancements.
Resource links:
Other parts in this series:
- Progressive Enhancement Techniques 1: the HTML
- Progressive Enhancement Techniques 2: the CSS
- Progressive Enhancement Techniques 3: the JavaScript
Related reading:
- Progressive Enhancement and Graceful Degradation: an Overview
- Progressive Enhancement and Graceful Degradation: Making a Choice
- 5 Reasons Why You Should Not Publish Supported Browser Lists
- Microsoft Office Online: a Case Against Supported Browser Lists
- Who’s Using ARIA?
Frequently Asked Questions (FAQs) on Progressive Enhancement in CSS
What is the main difference between Progressive Enhancement and Graceful Degradation?
Progressive Enhancement and Graceful Degradation are two methodologies used in web design. The main difference between them lies in their approach. Progressive Enhancement starts with a basic, functional website and then adds more complex functionalities or enhancements for more advanced browsers or devices. On the other hand, Graceful Degradation starts with a complex site with all the features and then scales back for older browsers or devices. The goal of both is to provide a good user experience across all devices and browsers, but their approach is different.
How does Progressive Enhancement benefit SEO?
Progressive Enhancement is beneficial for SEO because it ensures that all content is accessible to all users, including search engine bots. Since the basic content is available to all, it can be easily crawled and indexed by search engines, improving the site’s visibility and ranking. Moreover, it also improves the site’s usability and user experience, which are important ranking factors for search engines.
Can Progressive Enhancement be used with CSS Grid?
Yes, Progressive Enhancement can be used with CSS Grid. CSS Grid is a powerful layout system that allows for complex responsive layouts. However, not all browsers support CSS Grid. By using Progressive Enhancement, you can provide a basic layout for browsers that do not support CSS Grid and then enhance it for those that do. This ensures that your site is functional and usable on all browsers.
What are some techniques for implementing Progressive Enhancement in CSS?
There are several techniques for implementing Progressive Enhancement in CSS. One common technique is to use feature detection to check if a browser supports a certain feature before applying it. Another technique is to use fallbacks for older browsers. For example, you can use CSS variables with a fallback for browsers that do not support them. You can also use @supports rule to apply styles only if a certain feature is supported.
How does Progressive Enhancement improve accessibility?
Progressive Enhancement improves accessibility by ensuring that the basic content and functionality of a website are accessible to all users, regardless of their device or browser. This includes users with disabilities who may be using assistive technologies. By starting with a basic, functional website and then adding enhancements for more advanced browsers or devices, Progressive Enhancement ensures that everyone can access and use the site.
Is Progressive Enhancement still relevant today?
Yes, Progressive Enhancement is still relevant today. With the wide variety of devices and browsers in use today, it’s more important than ever to ensure that your website is accessible and functional for all users. Progressive Enhancement allows you to do this by starting with a basic, functional website and then adding enhancements for more advanced browsers or devices.
How does Progressive Enhancement relate to responsive web design?
Progressive Enhancement and responsive web design are both methodologies that aim to make websites accessible and usable on all devices and browsers. While responsive web design focuses on adjusting the layout and design of a website based on the device’s screen size, Progressive Enhancement focuses on starting with a basic, functional website and then adding enhancements for more advanced browsers or devices. Both methodologies complement each other and can be used together to create a website that is both responsive and progressively enhanced.
Can Progressive Enhancement be used with JavaScript?
Yes, Progressive Enhancement can be used with JavaScript. In fact, it’s a common practice to use Progressive Enhancement with JavaScript to ensure that the basic functionality of a website is accessible even if JavaScript is disabled or not supported by the browser. This is done by providing a basic functionality that works without JavaScript and then enhancing it with JavaScript for browsers that support it.
What are some challenges in implementing Progressive Enhancement?
Some challenges in implementing Progressive Enhancement include ensuring compatibility with a wide range of browsers and devices, testing the website on different browsers and devices, and maintaining the balance between providing basic functionality and enhancing it for more advanced browsers or devices. It can also be challenging to implement Progressive Enhancement with certain features or technologies that are not widely supported.
How does Progressive Enhancement affect performance?
Progressive Enhancement can improve performance by ensuring that only the necessary features and enhancements are loaded for each browser or device. This can reduce the amount of data that needs to be downloaded and processed, resulting in faster load times. Moreover, by starting with a basic, functional website, Progressive Enhancement ensures that the site is usable even if some features take longer to load.
Craig is a freelance UK web consultant who built his first page for IE2.0 in 1995. Since that time he's been advocating standards, accessibility, and best-practice HTML5 techniques. He's created enterprise specifications, websites and online applications for companies and organisations including the UK Parliament, the European Parliament, the Department of Energy & Climate Change, Microsoft, and more. He's written more than 1,000 articles for SitePoint and you can find him @craigbuckler.
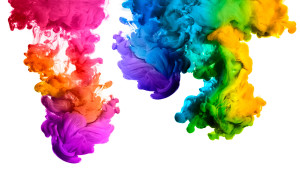
Published in
·automation·Development Environment·E-Commerce·Libraries·Miscellaneous·Patterns & Practices·PHP·February 13, 2017
Published in
·Debugging·Development Environment·Meta·Patterns & Practices·PHP·Programming·July 1, 2014