Quick jQuery code snippet to extract numbers from string using a replace regex method.
function getId(str)
{
return str.replace(/[^d.,]+/,'');
}
Frequently Asked Questions (FAQs) about Extracting Numbers from a String using jQuery
How can I extract multiple numbers from a string using jQuery?
To extract multiple numbers from a string using jQuery, you can use the match() method with a global regular expression. The match() method searches a string for a match against a regular expression, and returns the matches, as an array. The global regular expression (g) is used to find all matches rather than stopping after the first match. Here is an example:var str = "The price is 25 dollars and 50 cents";
var numbers = str.match(/\d+/g);
console.log(numbers); // Output: ["25", "50"]
Can I extract both integers and floating-point numbers from a string?
Yes, you can extract both integers and floating-point numbers from a string using jQuery. You can use the match() method with a regular expression that matches both integers and floating-point numbers. Here is an example:var str = "The price is 25.50 dollars";
var numbers = str.match(/(\d+\.\d+|\d+)/g);
console.log(numbers); // Output: ["25.50"]
How can I remove numbers from a string using jQuery?
To remove numbers from a string using jQuery, you can use the replace() method with a regular expression that matches numbers. The replace() method searches a string for a specified value, or a regular expression, and returns a new string where the specified values are replaced. Here is an example:var str = "The price is 25 dollars";
var newStr = str.replace(/\d+/g, "");
console.log(newStr); // Output: "The price is dollars"
Can I extract numbers from a string and convert them to integers or floating-point numbers?
Yes, you can extract numbers from a string and convert them to integers or floating-point numbers using jQuery. After extracting the numbers as strings using the match() method, you can convert them to integers using the parseInt() function or to floating-point numbers using the parseFloat() function. Here is an example:var str = "The price is 25.50 dollars";
var numbers = str.match(/(\d+\.\d+|\d+)/g);
var floatNumber = parseFloat(numbers[0]);
console.log(floatNumber); // Output: 25.5
How can I extract numbers from a string and add them together?
To extract numbers from a string and add them together using jQuery, you can use the match() method to extract the numbers, and then use the reduce() method to add them together. The reduce() method reduces an array to a single value by executing a provided function for each value of the array. Here is an example:var str = "The price is 25 dollars and 50 cents";
var numbers = str.match(/\d+/g);
var sum = numbers.reduce(function(a, b) {
return parseInt(a) + parseInt(b);
}, 0);
console.log(sum); // Output: 75
Can I extract numbers from a string and sort them?
Yes, you can extract numbers from a string and sort them using jQuery. After extracting the numbers as strings using the match() method, you can sort them using the sort() method. The sort() method sorts the elements of an array in place and returns the array. Here is an example:var str = "The numbers are 25, 50, and 10";
var numbers = str.match(/\d+/g);
numbers.sort(function(a, b) {
return a - b;
});
console.log(numbers); // Output: ["10", "25", "50"]
How can I extract numbers from a string and find the largest or smallest number?
To extract numbers from a string and find the largest or smallest number using jQuery, you can use the match() method to extract the numbers, and then use the Math.max() or Math.min() function to find the largest or smallest number. Here is an example:var str = "The numbers are 25, 50, and 10";
var numbers = str.match(/\d+/g);
var maxNumber = Math.max.apply(null, numbers);
var minNumber = Math.min.apply(null, numbers);
console.log(maxNumber); // Output: 50
console.log(minNumber); // Output: 10
Can I extract numbers from a string and find the average?
Yes, you can extract numbers from a string and find the average using jQuery. After extracting the numbers as strings using the match() method, you can add them together using the reduce() method, and then divide the sum by the number of numbers. Here is an example:var str = "The numbers are 25, 50, and 10";
var numbers = str.match(/\d+/g);
var sum = numbers.reduce(function(a, b) {
return parseInt(a) + parseInt(b);
}, 0);
var average = sum / numbers.length;
console.log(average); // Output: 28.333333333333332
How can I extract numbers from a string and find the median?
To extract numbers from a string and find the median using jQuery, you can use the match() method to extract the numbers, sort them, and then find the middle number. If there is an even number of numbers, the median is the average of the two middle numbers. Here is an example:var str = "The numbers are 25, 50, and 10";
var numbers = str.match(/\d+/g);
numbers.sort(function(a, b) {
return a - b;
});
var median;
if (numbers.length % 2 === 0) {
median = (parseInt(numbers[numbers.length / 2 - 1]) + parseInt(numbers[numbers.length / 2])) / 2;
} else {
median = parseInt(numbers[(numbers.length - 1) / 2]);
}
console.log(median); // Output: 25
Can I extract numbers from a string and find the mode?
Yes, you can extract numbers from a string and find the mode using jQuery. After extracting the numbers as strings using the match() method, you can count the frequency of each number using a JavaScript object, and then find the number with the highest frequency. Here is an example:var str = "The numbers are 25, 25, 50, and 10";
var numbers = str.match(/\d+/g);
var frequency = {};
var maxFrequency = 0;
var mode;
numbers.forEach(function(number) {
frequency[number] = (frequency[number] || 0) + 1;
if (frequency[number] > maxFrequency) {
maxFrequency = frequency[number];
mode = number;
}
});
console.log(mode); // Output: "25"
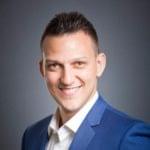
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.