//sets
$("div.header").css("margin","10px");
$("div.header").css("padding","10px");
Common sense, you would think this would work, but doesn’t seem to.
//gets
$("div.header").css("margin");
$("div.header").css("padding");
Try it yourself (paste code below into Firebug). You’ll see empty values returned for margin and padding. Maybe I’m missing something here?
(function($){
function logMarginPadding(elem)
{
//gets
var margin = elem.css("margin"),
padding = elem.css("padding");
console.log("margin="+margin+" padding="+padding);
}
var elem = $("div.header"); //set the element to inspect
logMarginPadding(elem);
//sets
elem.css("margin","10px");
elem.css("padding","10px");
logMarginPadding(elem);
})(jQuery);
This seems to work.
//get top margin of element
alert($("div.header").css("margin-top"));
//get top margin of element as integer value
alert($("a").css("margin-top").replace("px", ""));
//if using it with a calculation you must convert it to an integer
parseInt($("a").css('padding-left').replace("px", ""));
Anyways, this is how I got the padding for the element. Not ideal and only works if the padding is uniform (same on each side) Simply get the width and outer width divided by 2.
var item = $('div.header');
width = item.width(),
padding = (item.outerWidth()-width)/2;
There is pretty popular plugin called jsizes which may a good solution to getting/setting margin/padding values on elements.
JSizes is a small plugin for the jQuery JavaScript library which adds support for querying and setting the CSS min-width, min-height, max-width, max-height, border-*-width, margin, and padding properties.Further readings jQuery has the funtions outerWidth and outerHeight which include the border and padding by default, and also the margin if the first argument of the function is true http://api.jquery.com/outerHeight/ http://api.jquery.com/outerWidth/
Frequently Asked Questions (FAQs) about jQuery Element Padding/Margin
How Can I Use jQuery to Get the Padding of an Element?
To get the padding of an element using jQuery, you can use the CSS() method. This method returns the computed style property values. For instance, to get the padding of a div element, you can use the following code:var padding = $("div").css("padding");
This will return the padding value of the div element in pixels.
How Can I Set the Margin of an Element Using jQuery?
You can set the margin of an element using the CSS() method in jQuery. This method sets one or more style properties for the selected elements. Here’s an example of how to set the margin of a div element:$("div").css("margin", "20px");
This will set the margin of the div element to 20 pixels.
What is the Difference Between outerWidth() and outerHeight() in jQuery?
The outerWidth() method in jQuery returns the width of an element, including padding and border. If the optional parameter is set to true, it also includes the margin. On the other hand, the outerHeight() method returns the height of an element, including padding and border. If the optional parameter is set to true, it also includes the margin.
How Can I Get the Dimensions of an Element Using jQuery?
jQuery provides several methods to get the dimensions of an element. These include width(), height(), innerWidth(), innerHeight(), outerWidth(), and outerHeight(). Each of these methods returns the respective dimension of the selected element.
How Can I Use jQuery to Change the Dimensions of an Element?
You can use the width(), height(), innerWidth(), innerHeight(), outerWidth(), and outerHeight() methods to change the dimensions of an element in jQuery. These methods not only return the respective dimension of the selected element but can also set a new dimension when a value is passed as an argument.
What is the Difference Between innerWidth() and outerWidth() in jQuery?
The innerWidth() method in jQuery returns the width of an element, including padding but not the border or margin. On the other hand, the outerWidth() method returns the width of an element, including padding and border. If the optional parameter is set to true, it also includes the margin.
How Can I Use jQuery to Get the Border Width of an Element?
jQuery does not provide a direct method to get the border width of an element. However, you can use the CSS() method to get the computed style property values, including the border width. Here’s an example:var borderWidth = $("div").css("border-width");
This will return the border width of the div element in pixels.
How Can I Use jQuery to Set the Padding and Margin of an Element Simultaneously?
You can use the CSS() method in jQuery to set the padding and margin of an element simultaneously. Here’s an example:$("div").css({
"padding": "10px",
"margin": "20px"
});
This will set the padding and margin of the div element to 10 pixels and 20 pixels, respectively.
How Can I Use jQuery to Get the Margin of an Element?
You can use the CSS() method in jQuery to get the margin of an element. This method returns the computed style property values. For instance, to get the margin of a div element, you can use the following code:var margin = $("div").css("margin");
This will return the margin value of the div element in pixels.
How Can I Use jQuery to Set the Border Width of an Element?
You can use the CSS() method in jQuery to set the border width of an element. This method sets one or more style properties for the selected elements. Here’s an example of how to set the border width of a div element:$("div").css("border-width", "2px");
This will set the border width of the div element to 2 pixels.
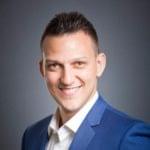
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.